TypeScript and Big Data: Processing Large Datasets
Big Data is transforming industries, and the need to process vast amounts of data efficiently is more critical than ever. TypeScript, with its statically-typed nature and scalability, can be a game-changer when it comes to handling large datasets. In this blog, we will explore the intersection of TypeScript and Big Data, showcasing how TypeScript can help you tackle massive datasets with ease.
Table of Contents
1. Why TypeScript for Big Data?
1.1. Strong Typing for Data Integrity
One of the key advantages of TypeScript in the context of Big Data is its strong typing system. TypeScript enforces strict data types, which can help prevent common data-related errors that can be challenging to spot in dynamically-typed languages like JavaScript. When working with large datasets, data integrity is paramount, and TypeScript’s type checking can catch many potential issues at compile time rather than runtime.
typescript // TypeScript ensures data types match. const userCount: number = 1000; const averageAge: string = "35"; // Error: Type 'string' is not assignable to type 'number'.
1.2. Scalability and Maintainability
As datasets grow, the complexity of data processing also increases. TypeScript’s support for scalability through code organization and maintainability is a significant benefit. It allows you to break down your data processing tasks into modular components with well-defined types and interfaces, making it easier to manage and extend your codebase as your data processing needs evolve.
1.3. IDE Support and Tooling
TypeScript enjoys excellent support from modern Integrated Development Environments (IDEs) like Visual Studio Code. This means you’ll have access to features such as intelligent code completion, real-time error checking, and debugging, which can significantly improve your productivity when working with large datasets.
2. Setting Up Your TypeScript Environment
Before diving into Big Data processing, you’ll need to set up a TypeScript environment. Here’s a high-level overview of the steps involved:
- Install Node.js and npm: TypeScript runs on Node.js, so ensure you have it installed on your system.
- Create a TypeScript Project: Initialize a new TypeScript project using tsc (the TypeScript compiler) or use a build tool like webpack to manage your TypeScript files.
- Install Necessary Packages: Depending on your data processing requirements, you may need additional packages like csv-parser for CSV file handling or rxjs for stream processing.
- Configuration: Set up a tsconfig.json file to configure TypeScript options, including target ECMAScript version, module resolution, and more.
- Coding: Start coding your data processing logic.
3. Reading and Parsing Large Datasets
Processing Big Data begins with reading and parsing the dataset. TypeScript provides various approaches for handling large datasets, depending on your specific use case:
3.1. Streaming Data
When dealing with extremely large datasets that cannot fit into memory, streaming is the way to go. You can use libraries like readline to read files line by line, processing each chunk of data as it becomes available. This approach minimizes memory usage and allows you to work with datasets of practically any size.
Here’s a simplified example of reading and processing a large CSV file using Node.js and TypeScript:
typescript import * as fs from 'fs'; import * as readline from 'readline'; const fileStream = fs.createReadStream('large_data.csv'); const rl = readline.createInterface({ input: fileStream, crlfDelay: Infinity, }); rl.on('line', (line) => { // Process each line of data here. });
3.2. Batch Processing
For moderately-sized datasets that can fit into memory comfortably, batch processing may be a more straightforward approach. You can load the entire dataset into memory and process it as needed. TypeScript’s strong typing ensures data consistency and integrity during this process.
typescript import fs from 'fs'; const data = fs.readFileSync('medium_data.json', 'utf-8'); const jsonData: Array<{ name: string; age: number }> = JSON.parse(data); // Process jsonData as needed.
4. Data Transformation and Cleansing
Once you have the data in memory, you can perform various data transformations and cleansing operations. TypeScript’s static typing helps you catch data transformation errors early in the development process.
typescript const cleanedData = jsonData.map((entry) => ({ name: entry.name.trim(), age: entry.age, })); // Further data transformations...
5. Aggregating and Analyzing Data
With the data prepared, you can start aggregating and analyzing it. TypeScript allows you to define complex data structures and perform calculations with confidence.
typescript const ageSum = cleanedData.reduce((sum, entry) => sum + entry.age, 0); const averageAge = ageSum / cleanedData.length; console.log(`Average Age: ${averageAge}`);
6. Handling Data Quality and Errors
In real-world Big Data scenarios, data quality is a significant concern. TypeScript’s strong typing can help you implement data quality checks and error handling strategies to deal with imperfect data gracefully.
typescript const validData = cleanedData.filter((entry) => { if (entry.age >= 0 && entry.age <= 120) { return true; } else { console.error(`Invalid age: ${entry.age}`); return false; } });
7. Optimizing Performance
Efficient Big Data processing often requires optimizing for performance. TypeScript offers several avenues for optimization:
7.1. Concurrency and Parallelism
To speed up data processing, consider using concurrency and parallelism techniques. TypeScript’s support for asynchronous programming with Promises and async/await can help you process data concurrently.
typescript async function processDataConcurrently(data: any[]) { const processedData = await Promise.all( data.map(async (entry) => { // Process each entry concurrently. }) ); return processedData; }
7.2. TypeScript Compiler Optimizations
TypeScript’s compiler, tsc, offers various optimizations that can improve the performance of your code. Experiment with options like –target, –lib, and –module to generate more efficient JavaScript code.
Conclusion
In this blog, we’ve explored the marriage of TypeScript and Big Data, demonstrating how TypeScript’s strong typing, scalability, and tooling support make it a valuable choice for processing large datasets. From reading and parsing data to data transformation, cleansing, aggregation, and optimization, TypeScript can streamline the entire Big Data processing pipeline.
As you embark on your Big Data journey, remember that TypeScript is not just a language for web development—it’s a versatile tool that can empower you to conquer the challenges posed by massive datasets and extract valuable insights from them. With TypeScript in your toolkit, you’ll be well-equipped to tackle the world of Big Data head-on.
Whether you’re analyzing customer behavior, processing sensor data, or working on any other data-intensive task, TypeScript can be your ally in harnessing the potential of Big Data.
Start exploring TypeScript’s capabilities in the realm of Big Data today, and unlock new possibilities for your data-driven projects. Happy coding!
Table of Contents
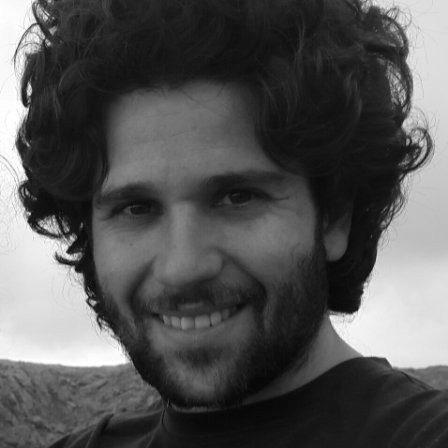
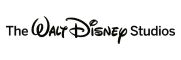