TypeScript and Blockchain: Building Decentralized Apps
Blockchain technology has disrupted industries and redefined trust on the internet. Its decentralized nature, immutability, and security features have given rise to a plethora of applications beyond cryptocurrencies. One of the most intriguing developments in this space is the creation of decentralized applications (dApps). In this blog post, we’ll explore how TypeScript, a statically-typed superset of JavaScript, can be leveraged to build robust and secure dApps on the blockchain.
Table of Contents
1. Understanding Blockchain and dApps
1.1. What is Blockchain?
Blockchain is a distributed ledger technology that records transactions across multiple computers, ensuring transparency, security, and immutability. It serves as the underlying infrastructure for various applications, with its most famous application being cryptocurrencies like Bitcoin and Ethereum.
1.2. What are dApps?
Decentralized applications (dApps) are software applications that run on a blockchain network rather than traditional centralized servers. These apps leverage the blockchain’s decentralized and trustless nature to provide secure and transparent services. dApps typically consist of two main components: smart contracts and a user interface.
2. TypeScript: The Perfect Companion for dApp Development
2.1. Why TypeScript?
TypeScript offers strong static typing, enhanced tooling, and improved code maintainability compared to JavaScript. These advantages are especially valuable when building dApps, where security and reliability are paramount. TypeScript helps catch potential issues at compile-time, reducing the risk of vulnerabilities in the final product.
2.2. Setting Up Your TypeScript Environment
Let’s start by setting up a TypeScript development environment for building dApps. We’ll use Node.js and the Truffle framework, a popular development framework for Ethereum dApps. First, ensure you have Node.js installed, and then proceed with the following steps:
Step 1: Install Truffle
bash npm install -g truffle
Step 2: Create a New Truffle Project
bash mkdir my-dapp cd my-dapp truffle init
Step 3: Install TypeScript
bash npm install --save-dev typescript ts-node @types/node
Step 4: Configure TypeScript
Create a tsconfig.json file in the project root with the following content:
json { "compilerOptions": { "target": "es2017", "module": "commonjs", "strict": true, "esModuleInterop": true, "skipLibCheck": true, "forceConsistentCasingInFileNames": true, "outDir": "./build", "types": ["node"] }, "include": ["./**/*.ts"], "exclude": ["node_modules"] }
Now your TypeScript environment is ready to go.
3. Smart Contracts in TypeScript
3.1. What Are Smart Contracts?
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They live on the blockchain and automatically enforce these terms when predefined conditions are met. Ethereum, a popular blockchain for dApps, uses a programming language called Solidity for writing smart contracts. However, you can write smart contracts in TypeScript for certain blockchain platforms like EOS.
3.2. Writing a Simple Smart Contract in TypeScript
Let’s create a basic example of a smart contract in TypeScript. We’ll write a simple token contract, which allows users to transfer tokens between each other. In this contract, we’ll define the structure of the token and implement transfer functions.
typescript // Token.sol.ts class Token { private balances: Map<string, number> = new Map(); constructor() {} transfer(sender: string, receiver: string, amount: number): void { const senderBalance = this.balances.get(sender) || 0; if (senderBalance < amount) { throw new Error("Insufficient balance"); } this.balances.set(sender, senderBalance - amount); const receiverBalance = this.balances.get(receiver) || 0; this.balances.set(receiver, receiverBalance + amount); } getBalance(account: string): number { return this.balances.get(account) || 0; } }
This is a simple TypeScript class representing a token contract. It maintains a balance for each account and allows transfers between them.
3.3. Compiling TypeScript Smart Contracts
To compile TypeScript smart contracts to a format compatible with the blockchain, you need to use a compiler like eosio.cdt for EOS or ethers.js for Ethereum. The compilation process may vary depending on the blockchain platform you choose.
4. Web3: Interacting with the Blockchain
4.1. What is Web3?
Web3.js is a JavaScript library that provides an interface for interacting with Ethereum-based blockchains. While Ethereum uses Solidity for smart contracts, TypeScript can be used to build the frontend of dApps, connecting to the blockchain through Web3.js.
4.2. Setting Up Web3 in TypeScript
To set up Web3.js in your TypeScript project, follow these steps:
Step 1: Install Web3.js
bash npm install web3
Step 2: Import Web3 in Your TypeScript File
typescript import Web3 from 'web3'; const web3 = new Web3(new Web3.providers.HttpProvider('http://localhost:8545')); // Replace with your Ethereum node URL
Now you have a Web3 instance ready to connect to your Ethereum node.
4.3. Interacting with Smart Contracts
Once you have Web3.js set up, you can interact with smart contracts on the Ethereum blockchain. Let’s create a TypeScript function to send tokens using our previously defined token contract.
typescript async function sendTokens(sender: string, receiver: string, amount: number): Promise<void> { const contractAddress = '0xContractAddress'; // Replace with your contract address const contractAbi = [...]; // Replace with your contract ABI const contract = new web3.eth.Contract(contractAbi, contractAddress); const senderPrivateKey = '0xYourPrivateKey'; // Replace with your sender's private key const senderAccount = web3.eth.accounts.privateKeyToAccount(senderPrivateKey); const data = contract.methods.transfer(receiver, amount).encodeABI(); const gasPrice = await web3.eth.getGasPrice(); const gasLimit = 21000; // Adjust the gas limit as needed const transaction = { to: contractAddress, gas: gasLimit, gasPrice: gasPrice, data: data, }; const signedTx = await web3.eth.accounts.signTransaction(transaction, senderPrivateKey); await web3.eth.sendSignedTransaction(signedTx.rawTransaction); }
This function allows you to send tokens from one Ethereum account to another using your smart contract.
5. Security Considerations for dApp Development
Building decentralized applications comes with unique security challenges. Here are some best practices to ensure the security of your TypeScript-based dApps:
5.1. Avoid Hardcoding Private Keys
Never hardcode private keys in your source code. Use environment variables or secure key management solutions to store and access private keys.
5.2. Implement Access Control
Ensure that only authorized users can interact with your smart contracts. Implement role-based access control to restrict certain actions to specific users or addresses.
5.3. Test Extensively
Thoroughly test your smart contracts using tools like Truffle, Hardhat, or Ganache. Test for various scenarios, including edge cases, to identify and fix vulnerabilities.
5.4. Use Standard Libraries
Whenever possible, use well-established and audited libraries for your smart contract development. Avoid reinventing the wheel, as custom code can introduce vulnerabilities.
5.5. Keep Contracts Simple
Complexity often leads to bugs and vulnerabilities. Keep your smart contracts as simple as possible, following the principle of “simplicity is security.”
5.6. Continuous Monitoring
Regularly monitor your dApp and the blockchain for any unusual activity. Implement logging and alerts to detect and respond to security incidents.
Conclusion
TypeScript is a powerful tool for building decentralized applications on the blockchain. Its strong typing and development advantages make it an excellent choice for ensuring the security and reliability of dApps. By combining TypeScript with Web3.js, you can create secure and user-friendly interfaces for interacting with smart contracts on blockchain networks like Ethereum.
As you embark on your journey to dApp development, remember to prioritize security, keep your code clean, and stay updated with the rapidly evolving blockchain ecosystem. With the right tools and practices, you can contribute to the growing world of decentralized applications and blockchain technology.
Start building your TypeScript-powered dApp today and be part of the blockchain revolution!
Table of Contents
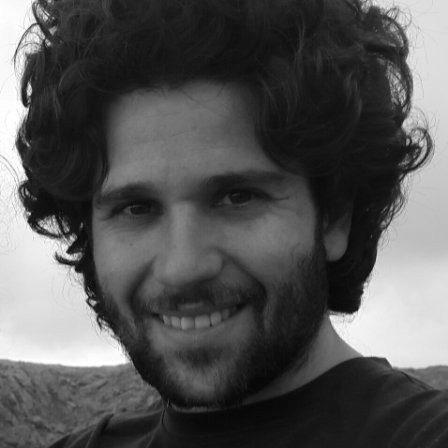
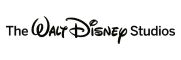