Building Scalable Web Applications with TypeScript
In today’s rapidly evolving digital landscape, the demand for web applications that can handle massive user traffic and maintain peak performance is higher than ever. To meet these expectations, developers need to embrace technologies and techniques that enable scalability. TypeScript, a statically typed superset of JavaScript, has gained significant popularity in recent years for its ability to enhance code quality, maintainability, and scalability. In this blog post, we will delve into the world of building scalable web applications using TypeScript. We’ll explore various strategies, best practices, and code examples that will empower you to create robust and efficient systems capable of handling growing user bases and heavy workloads.
Table of Contents
1. Understanding Scalability in Web Applications
Scalability is the capability of a system to handle increasing amounts of work, traffic, or data without sacrificing performance. When it comes to web applications, there are two primary types of scalability: horizontal and vertical.
1.1. Horizontal vs. Vertical Scalability
- Horizontal Scalability: Also known as “scaling out,” this approach involves adding more machines or instances to distribute the workload. It’s like adding more lanes to a highway to accommodate more vehicles. TypeScript’s dynamic typing and object-oriented features can make scaling out more manageable as codebase complexity grows.
- Vertical Scalability: Referred to as “scaling up,” this method focuses on enhancing the performance of a single machine. It involves upgrading the resources (CPU, RAM) of a server to handle increased load. TypeScript’s static typing can help identify potential performance bottlenecks early in the development process.
1.2. The Role of TypeScript in Scalable Development
TypeScript’s static typing helps catch errors at compile time, reducing the likelihood of runtime errors that could lead to scalability problems. Additionally, TypeScript’s support for modern ECMAScript features enables developers to write clean and efficient code. This aids in creating maintainable codebases, which is crucial for long-term scalability.
2. Designing for Scalability
When building scalable web applications, the architecture and design choices play a pivotal role. Here are some best practices to consider:
2.1. Modular Architecture
Divide your application into smaller, self-contained modules that can be developed, tested, and deployed independently. TypeScript’s module system provides a structured way to organize code, making it easier to manage and scale.
typescript // Example of a modular TypeScript module // userService.ts export class UserService { // ... } // authService.ts export class AuthService { // ... } // app.ts import { UserService } from './userService'; import { AuthService } from './authService'; const userService = new UserService(); const authService = new AuthService();
2.2. Separation of Concerns
Adhere to the SOLID principles and separate different concerns of your application. This makes it easier to make changes to specific parts without affecting the entire system.
typescript // Example of separation of concerns class UserValidator { // ... } class UserManager { // ... } class UserController { constructor(private userManager: UserManager, private userValidator: UserValidator) { // ... } }
2.3. Microservices and APIs
Consider using a microservices architecture for complex applications. Each microservice can be developed and scaled independently, allowing you to focus resources on specific functionalities. TypeScript’s strong typing can help ensure API contracts are well-defined and maintained.
typescript // Example of a microservice architecture with TypeScript // userMicroservice.ts export class UserMicroservice { // ... } // productMicroservice.ts export class ProductMicroservice { // ... } // orderMicroservice.ts export class OrderMicroservice { // ... }
3. Leveraging Asynchronous Programming
Asynchronous programming is essential for scalability, as it enables applications to handle multiple tasks concurrently without blocking the main thread.
3.1. Promises and Async/Await
Use Promises and Async/Await to write asynchronous code that is more readable and maintainable. TypeScript’s type annotations help ensure that promises are used correctly.
typescript // Example of using Async/Await with TypeScript async function fetchData(url: string): Promise<any> { const response = await fetch(url); const data = await response.json(); return data; }
3.2. Handling Concurrency
To manage concurrency, consider using tools like worker threads or thread pools. This can help distribute CPU-intensive tasks and prevent bottlenecks.
typescript // Example of using worker threads with TypeScript import { Worker, isMainThread, parentPort } from 'worker_threads'; if (isMainThread) { const worker = new Worker(__filename); worker.on('message', (result) => { // Handle the result from the worker }); } else { // Perform CPU-intensive tasks const result = performTasks(); parentPort.postMessage(result); }
3.3. Reactive Programming with RxJS
Reactive programming with libraries like RxJS can help manage asynchronous data streams effectively. This is particularly useful when dealing with real-time updates and event-driven architectures.
typescript // Example of using RxJS for reactive programming import { Observable, fromEvent } from 'rxjs'; const button = document.getElementById('myButton'); const clickStream = fromEvent(button, 'click'); clickStream.subscribe(() => { // Handle button clicks });
4. Database and Data Management
Choosing the right database and implementing efficient data management strategies are crucial for scalable applications.
4.1. Choosing the Right Database
Select a database that aligns with your application’s requirements. NoSQL databases like MongoDB and Cassandra can provide horizontal scalability, while relational databases like PostgreSQL offer strong consistency and ACID compliance.
typescript // Example of using a MongoDB database with TypeScript import { MongoClient } from 'mongodb'; const client = new MongoClient('mongodb://localhost:27017'); client.connect(); const db = client.db('myapp'); const usersCollection = db.collection('users');
4.2. Data Indexing and Caching
Create appropriate indexes for database queries to improve query performance. Utilize in-memory caching solutions like Redis to store frequently accessed data and reduce database load.
typescript // Example of using Redis for caching with TypeScript import redis from 'redis'; const client = redis.createClient(); client.set('key', 'value'); client.get('key', (err, reply) => { console.log(reply); });
4.3. Replication and Sharding
Implement database replication to ensure data availability and fault tolerance. Sharding can help distribute data across multiple servers, preventing any single server from becoming a bottleneck.
typescript // Example of database replication and sharding // Replication const primary = new MongoDBServer('primary'); const replica1 = new MongoDBServer('replica1'); const replica2 = new MongoDBServer('replica2'); primary.syncData(replica1, replica2); // Sharding const shard1 = new MongoDBServer('shard1'); const shard2 = new MongoDBServer('shard2'); shard1.shardData(collectionA); shard2.shardData(collectionB);
5. Performance Optimization
Efficient algorithms, caching strategies, and load balancing are essential for achieving optimal performance in scalable applications.
5.1. Efficient Algorithms and Data Structures
Choose algorithms and data structures that offer fast and predictable performance. TypeScript’s static typing can help catch potential algorithmic inefficiencies during development.
typescript // Example of an efficient algorithm with TypeScript function findMaximum(arr: number[]): number { let max = arr[0]; for (const num of arr) { if (num > max) { max = num; } } return max; }
5.2. Caching Strategies
Implement caching to reduce the need for repetitive computations or database queries. Use cache eviction policies to manage memory consumption.
typescript // Example of caching with TypeScript class Cache { private data: Record<string, any> = {}; get(key: string): any { return this.data[key]; } set(key: string, value: any): void { this.data[key] = value; } delete(key: string): void { delete this.data[key]; } }
5.3. Load Balancing
Distribute incoming requests across multiple servers using load balancers. This prevents any single server from becoming overwhelmed and improves overall application responsiveness.
typescript // Example of load balancing with TypeScript import http from 'http'; import cluster from 'cluster'; import os from 'os'; if (cluster.isMaster) { const numWorkers = os.cpus().length; for (let i = 0; i < numWorkers; i++) { cluster.fork(); } } else { http.createServer((req, res) => { // Handle incoming requests }).listen(3000); }
6. Monitoring and Diagnostics
To maintain a scalable application, it’s essential to monitor its performance and diagnose issues promptly.
6.1. Logging Techniques
Implement comprehensive logging to track application behavior, errors, and performance metrics. TypeScript’s type annotations can provide clarity to log messages.
typescript // Example of logging with TypeScript function calculateTotalPrice(items: Item[]): number { const totalPrice = items.reduce((total, item) => { // Log item details for debugging console.log(`Calculating price for ${item.name}`); return total + item.price; }, 0); // Log total price console.log(`Total price: ${totalPrice}`); return totalPrice; }
6.2. Performance Monitoring
Use tools like Prometheus and Grafana to monitor application performance metrics. This helps identify performance bottlenecks and plan scalability improvements.
typescript // Example of using Prometheus for performance monitoring import promClient from 'prom-client'; const httpRequestDurationMicroseconds = new promClient.Histogram({ name: 'http_request_duration_seconds', help: 'Duration of HTTP requests in microseconds', labelNames: ['route'], buckets: [0.1, 5, 15, 50, 100, 500], }); app.use((req, res, next) => { const start = process.hrtime(); res.on('finish', () => { const end = process.hrtime(start); const duration = end[0] * 1e6 + end[1] / 1e3; httpRequestDurationMicroseconds .labels(req.route.path) .observe(duration); }); next(); });
6.3. Error Tracking and Reporting
Integrate error tracking tools like Sentry or Rollbar to receive real-time alerts about application errors. This enables quick response and resolution of issues that could impact scalability.
typescript // Example of error tracking with TypeScript import * as Sentry from '@sentry/node'; Sentry.init({ dsn: 'your-sentry-dsn', }); try { // Code that may throw errors } catch (error) { Sentry.captureException(error); }
Conclusion
Building scalable web applications with TypeScript requires a combination of smart architectural choices, effective coding practices, and proper tooling. By understanding the nuances of scalability, leveraging TypeScript’s features, and implementing best practices, developers can create systems that handle increased workloads and user demands while maintaining excellent performance. Remember, scalability is not just about handling growth; it’s about crafting a resilient and adaptable foundation for your web applications to thrive in an ever-evolving digital world.
Table of Contents
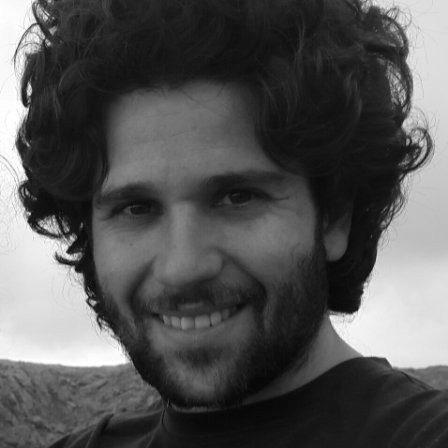
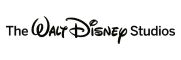