Continuous Integration with TypeScript Projects
In today’s fast-paced software development landscape, efficiency and quality are paramount. As projects grow in complexity, so do the challenges associated with ensuring code stability and reliability. This is where Continuous Integration (CI) comes into play. CI is a software development practice that focuses on automating the integration and testing of code changes. In this blog post, we’ll explore how Continuous Integration can greatly benefit TypeScript projects, streamline development workflows, and ensure the delivery of high-quality code.
Table of Contents
1. Introduction to Continuous Integration
1.1. Understanding the Basics:
Continuous Integration revolves around the idea of frequently integrating code changes from multiple developers into a shared repository. With each integration, automated tests are executed to catch potential issues early in the development cycle. This proactive approach helps identify bugs and compatibility problems, leading to quicker resolutions and smoother collaboration.
1.2. Benefits of CI in TypeScript Projects:
For TypeScript projects, Continuous Integration offers several advantages. It ensures that TypeScript code is compiled correctly, dependencies are up to date, and tests pass consistently. This guarantees that new code additions do not inadvertently break existing functionality, thus maintaining a higher level of stability throughout the development process.
2. Setting Up Continuous Integration for TypeScript Projects
2.1. Version Control and Repository Hosting:
Before diving into CI, a solid foundation is essential. Utilizing version control systems like Git and hosting repositories on platforms like GitHub or GitLab provides the basis for collaboration and code management.
2.2. Choosing a CI/CD Service:
Several CI/CD services, such as Travis CI, CircleCI, Jenkins, and GitHub Actions, are available. These services automate the process of building, testing, and deploying code changes. They offer integration with popular repository hosting platforms and allow for customizable workflows.
2.3. Configuration with CI/CD Files:
CI/CD services rely on configuration files placed within the project repository. These files define the steps to be executed during the CI process. In TypeScript projects, these steps typically involve installing dependencies, building the project, running tests, and performing code quality checks.
Here’s an example of a .travis.yml configuration file for Travis CI:
yaml language: node_js node_js: - "14" install: - npm install script: - npm run build - npm test
3. Automating TypeScript Project Builds
3.1. Building with TypeScript Compiler (tsc):
TypeScript projects need to be compiled to JavaScript before they can be executed in a browser or a Node.js environment. The TypeScript Compiler (tsc) performs this compilation. Integrating the TypeScript compilation step into your CI process ensures that any compilation errors are caught early.
3.2. Leveraging Package Managers (npm, Yarn):
Package managers like npm and Yarn simplify the management of project dependencies. Incorporating package manager commands into your CI workflow ensures that all required dependencies are installed before building and testing the project.
3.3. Ensuring Consistent Environments:
CI/CD services often provide isolated build environments to ensure consistency across different runs. This prevents issues caused by variations in development environments and dependencies.
4. Running Automated Tests
4.1. Writing Test Suites with Testing Frameworks (Jest, Mocha):
Automated tests are a cornerstone of CI. Testing frameworks like Jest and Mocha help create and run test suites. These frameworks allow you to define various types of tests, such as unit tests, integration tests, and end-to-end tests.
4.2. Incorporating Test Coverage Reports:
Test coverage reports provide insights into which parts of your codebase are covered by tests. Integrating coverage reporting tools like Istanbul helps maintain a healthy test coverage percentage and identifies areas that require additional testing.
4.3. Test Parallelization for Speed:
As your test suite grows, test execution can become time-consuming. Test parallelization, offered by some testing frameworks, allows you to run tests concurrently, reducing the overall test execution time.
5. Code Quality Checks
5.1. Linting TypeScript Code (ESLint, TSLint):
Linting tools analyze your code for potential issues, enforcing consistent code style and identifying potential bugs. Tools like ESLint and TSLint offer TypeScript-specific linting configurations.
5.2. Enforcing Code Formatting (Prettier):
Maintaining consistent code formatting enhances readability and collaboration. Prettier is a popular tool that automatically formats code according to predefined rules, ensuring consistent code style across the project.
5.3. Static Code Analysis:
Static analysis tools, such as SonarQube, can help identify complex code segments, potential security vulnerabilities, and maintainability issues. Integrating static code analysis into your CI pipeline contributes to overall code quality.
6. Integrating Continuous Deployment
6.1. Creating Deployment Pipelines:
Continuous Deployment takes CI a step further by automating the deployment of code changes to various environments. This ensures that validated and tested code reaches staging and production environments seamlessly.
6.2. Deploying to Staging and Production Environments:
By automating deployment, you reduce the risk of manual errors and ensure consistency between different environments. Deploying to staging environments first allows for final testing before code reaches production.
6.3. Monitoring Deployed Applications:
Continuous Deployment should be accompanied by continuous monitoring. Monitoring tools and services like Prometheus and Grafana help track the performance and health of deployed applications, allowing for prompt issue identification and resolution.
7. Best Practices for Effective Continuous Integration
7.1. Small and Frequent Commits:
Encourage developers to commit changes frequently in small increments. This reduces the complexity of integrations and makes it easier to pinpoint issues when they arise.
7.2. Feature Branches and Pull Requests:
Utilize feature branches to isolate changes for specific features or bug fixes. Pull requests enable peer review, ensuring that code adheres to standards and doesn’t introduce regressions.
7.3. Feedback Loop and Collaboration:
CI fosters collaboration among team members by providing continuous feedback on code quality and test results. This shortens the feedback loop, allowing developers to address issues promptly.
Conclusion
In conclusion, Continuous Integration is a game-changer for TypeScript projects, promoting code stability, collaboration, and efficient development processes. By automating build, test, and deployment processes, CI enhances the quality of software, reduces risks, and accelerates delivery. Incorporating these practices into your TypeScript projects can lead to smoother development workflows, higher code quality, and ultimately, more satisfied users.
Table of Contents
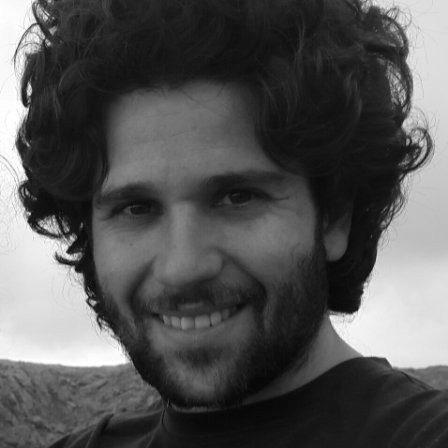
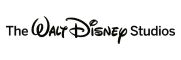