TypeScript and Cybersecurity: Protecting Your Applications
In today’s digital age, cybersecurity is a top priority for businesses and developers alike. With the increasing sophistication of cyber threats, it’s crucial to implement robust security measures in your applications from the very beginning. One powerful tool in your cybersecurity arsenal is TypeScript, a statically typed superset of JavaScript. In this blog post, we’ll explore how TypeScript can bolster the security of your applications, offering protection against common cybersecurity vulnerabilities.
Table of Contents
1. Understanding TypeScript
Before we dive into how TypeScript can enhance your application’s security, let’s briefly understand what TypeScript is and why it’s gaining popularity among developers.
1.1. What is TypeScript?
TypeScript is a statically typed programming language developed by Microsoft. It builds upon JavaScript by adding static type checking to the language. This means that TypeScript allows you to specify the types of variables, function parameters, and return values. The TypeScript compiler then checks your code for type-related errors during development, before it’s even run.
1.2. Why Choose TypeScript?
TypeScript offers several benefits that make it a preferred choice for many developers:
- Type Safety: TypeScript helps catch type-related errors at compile time, reducing the chances of runtime errors. This is a significant advantage when it comes to security.
- Code Maintainability: Strong typing leads to cleaner and more maintainable code, making it easier to spot and fix security issues.
- Tooling Support: TypeScript has excellent tooling support, including integrated development environments (IDEs) like Visual Studio Code, which provide real-time feedback and suggestions to improve code quality and security.
2. Protecting Against Injection Attacks
One of the most common cybersecurity vulnerabilities is injection attacks, where malicious code is injected into an application, often through user inputs. TypeScript can help prevent such attacks through strict typing.
Example: SQL Injection
Let’s consider a scenario where a user inputs data into a web application, and this input is used to construct an SQL query. Without proper type checking, an attacker could inject malicious SQL code, compromising the security of your database.
typescript // JavaScript code without TypeScript const userInput = req.body.username; const query = `SELECT * FROM users WHERE username = '${userInput}'`; // Malicious userInput value: 'admin' OR '1'='1' // Resulting query: SELECT * FROM users WHERE username = 'admin' OR '1'='1'
In the TypeScript world, you can specify the expected type for user input, ensuring it matches the intended usage.
typescript // TypeScript code const userInput: string = req.body.username; const query = `SELECT * FROM users WHERE username = '${userInput}'`; // TypeScript compiler error if userInput is not a string
By using TypeScript, you explicitly declare that userInput should be a string, preventing the injection of malicious code.
3. Enhancing Authentication and Authorization
Authentication and authorization are critical aspects of cybersecurity. TypeScript can help you build more robust authentication and authorization systems by enforcing type-safe checks.
Example: Role-Based Access Control
Consider an application where users have different roles, and certain functionalities are accessible only to users with specific roles. Without proper type checking, a developer might accidentally grant unauthorized access.
typescript // JavaScript code without TypeScript function hasAccess(user, role) { return user.roles.includes(role); } // Malicious usage const user = { roles: ['admin', 'user'] }; const isAdmin = hasAccess(user, 'admin'); // true
With TypeScript, you can define user roles as a finite set of string literals, ensuring that only valid roles are used.
typescript // TypeScript code type UserRole = 'admin' | 'user'; function hasAccess(user: { roles: UserRole[] }, role: UserRole): boolean { return user.roles.includes(role); } // TypeScript compiler error if an invalid role is used
In this TypeScript example, any attempt to use an invalid role would result in a compilation error, preventing unauthorized access.
4. Mitigating Cross-Site Scripting (XSS) Attacks
Cross-Site Scripting (XSS) attacks occur when an attacker injects malicious scripts into web applications, which are then executed in the context of other users’ browsers. TypeScript can help prevent XSS attacks by enforcing proper encoding and escaping of user-generated content.
Example: Unsafe Rendering
In a typical JavaScript application, rendering user-generated content directly in the HTML can be dangerous.
javascript // JavaScript code without TypeScript const userMessage = '<script>alert("You are hacked!");</script>'; const messageElement = document.getElementById('message'); messageElement.innerHTML = userMessage; // Executes the script
In TypeScript, you can use the innerHTML property safely by explicitly defining the type of the content.
typescript // TypeScript code const userMessage: string = '<script>alert("You are hacked!");</script>'; const messageElement = document.getElementById('message'); messageElement.innerHTML = userMessage; // TypeScript compiler error
By specifying userMessage as a string, TypeScript ensures that no code is executed when rendering user-generated content.
5. Preventing Data Tampering
Data tampering is a common threat where an attacker modifies data sent to the server or received from it. TypeScript can assist in identifying and preventing data tampering vulnerabilities.
Example: Insecure Data Transmission
In a scenario where sensitive data is transmitted to the server, a developer might inadvertently send data in an insecure manner.
javascript // JavaScript code without TypeScript const password = 'sensitive_password'; fetch('/login', { method: 'POST', body: JSON.stringify({ password }), });
In TypeScript, you can define a more secure way to transmit sensitive data using HTTPS.
typescript // TypeScript code const password: string = 'sensitive_password'; fetch('/login', { method: 'POST', body: JSON.stringify({ password }), }); // TypeScript compiler error if password is not a string
TypeScript ensures that sensitive data, such as passwords, is handled with the necessary care and type safety.
6. Auditing and Code Analysis
Beyond static typing, TypeScript provides tools for code analysis that can help identify security issues in your codebase.
6.1. Static Code Analysis
Static code analysis tools, like TSLint or ESLint with security plugins, can be configured to catch common security issues during development. These tools can flag insecure coding practices, such as using eval() or storing sensitive information in plain text.
typescript // Example ESLint rule for detecting the use of 'eval()' { "rules": { "no-eval": "error" } }
By integrating such tools into your TypeScript development workflow, you can proactively identify and rectify security vulnerabilities before they make it into production.
7. Security in the Dependency Ecosystem
Another area where TypeScript can enhance cybersecurity is in the management of dependencies. The npm ecosystem, while rich in packages, can introduce security risks if not managed properly.
7.1. Dependency Scanning
Tools like npm audit or third-party services like Snyk can be used to scan your project’s dependencies for known vulnerabilities. By maintaining a clear understanding of your dependencies and regularly updating them, you can reduce the risk of using packages with security issues.
bash # Using npm audit to check for vulnerabilities npm audit
TypeScript’s strong typing and static analysis can also assist in identifying potential security concerns in your dependencies, as they often manifest as type-related issues.
Conclusion
In an era where cyber threats are ever-evolving and increasingly sophisticated, developers need to take proactive steps to secure their applications. TypeScript, with its strong typing and code analysis capabilities, can be a valuable ally in your quest to build secure software. By leveraging TypeScript’s features, you can prevent common vulnerabilities such as injection attacks, authentication flaws, XSS attacks, and data tampering. Additionally, you can use static code analysis tools and dependency scanning to further bolster your application’s security.
As you continue to develop and maintain your applications, keep cybersecurity at the forefront of your concerns. TypeScript is just one of the many tools at your disposal, but it’s a powerful one that can significantly contribute to the protection of your digital assets. So, embrace TypeScript and build with confidence, knowing that you’re taking important steps to safeguard your applications and data against the ever-present threats of the digital world.
Incorporating TypeScript into your development process is not just about enhancing security; it’s also about fostering a culture of secure coding practices. By doing so, you not only protect your own applications but also contribute to a safer online environment for everyone.
Start your journey toward more secure applications today by exploring TypeScript and its robust capabilities in the realm of cybersecurity.
In this blog post, we’ve explored how TypeScript can enhance the security of your applications, offering protection against common cybersecurity vulnerabilities. We discussed how TypeScript helps prevent injection attacks, strengthens authentication and authorization, mitigates XSS attacks, prevents data tampering, and aids in code analysis. By leveraging TypeScript’s features, you can build more secure software and protect your digital assets from evolving cyber threats.
Table of Contents
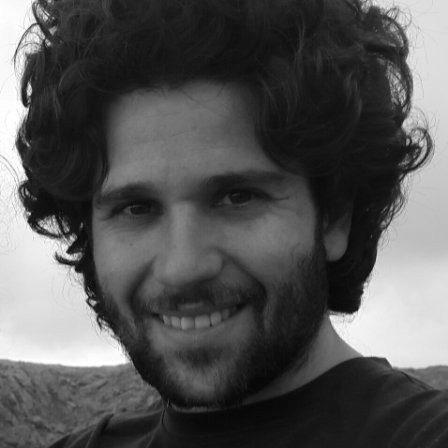
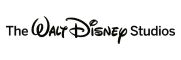