TypeScript and Data Visualization: Creating Stunning Charts
In today’s data-driven world, the ability to present information in a visually appealing and understandable way is paramount. Whether you’re analyzing sales trends, tracking user behavior, or simply trying to communicate complex data to your audience, data visualization is the key to success. TypeScript, with its strong typing and object-oriented features, is an ideal language for building robust and beautiful data visualizations. In this blog, we’ll explore how TypeScript can be leveraged to create stunning charts that not only convey data effectively but also enhance the overall user experience.
Table of Contents
1. Why TypeScript for Data Visualization?
Before we dive into the nitty-gritty of creating charts with TypeScript, let’s briefly discuss why TypeScript is a compelling choice for this task.
1.1. Strong Typing
One of TypeScript’s standout features is its static typing system. This means that TypeScript enforces strict data types, reducing the likelihood of runtime errors. When working with data visualization, especially in a large and complex project, type safety is a crucial factor. TypeScript ensures that your code is more predictable and less error-prone, ultimately leading to smoother development and better-maintained code.
1.2. IDE Support
TypeScript is well-supported by popular integrated development environments (IDEs) like Visual Studio Code. These IDEs offer features such as code completion, type checking, and real-time error highlighting, which can significantly boost your productivity when creating data visualizations.
1.3. Scalability
Data visualizations can grow in complexity as your project evolves. TypeScript’s modular and object-oriented nature makes it easier to scale your codebase without sacrificing maintainability. You can encapsulate different chart types and features into reusable components, making it simpler to manage a growing codebase.
2. Setting Up Your TypeScript Environment
To get started with TypeScript for data visualization, you’ll need a development environment that supports TypeScript. Here are the steps to set up a basic TypeScript environment:
2.1. Install Node.js and npm
If you don’t have Node.js and npm (Node Package Manager) installed on your system, download and install them from the official website (https://nodejs.org/). They are essential for running TypeScript and managing dependencies.
2.2. Create a Project Directory
Create a new directory for your TypeScript project and navigate to it using your terminal or command prompt.
shell mkdir data-visualization cd data-visualization
2.3. Initialize a TypeScript Project
Run the following command to initialize a new TypeScript project. This will create a tsconfig.json file, which is used to configure TypeScript settings.
shell npm init -y
2.4. Install TypeScript
Next, install TypeScript globally on your system and save it as a development dependency for your project.
shell npm install -g typescript npm install --save-dev typescript
2.5. Create a TypeScript File
Create a new TypeScript file, e.g., app.ts, in your project directory. This is where you’ll write your data visualization code.
3. Building Interactive Charts with TypeScript
Now that your TypeScript environment is set up, it’s time to start creating stunning charts. For this example, we’ll use the popular charting library, Chart.js, to build interactive charts in TypeScript.
3.1. Install Chart.js
To include Chart.js in your project, install it as a dependency:
shell npm install --save chart.js
3.2. Create an HTML File
In your project directory, create an index.html file to display your chart. Here’s a basic HTML structure:
html <!DOCTYPE html> <html> <head> <title>Stunning TypeScript Charts</title> </head> <body> <canvas id="myChart"></canvas> <script src="app.js"></script> </body> </html>
3.3. Write TypeScript Code
Now, let’s write TypeScript code in your app.ts file to create a simple bar chart using Chart.js. First, import Chart.js at the beginning of your app.ts file:
typescript import 'chart.js'; // Your TypeScript code for creating the chart goes here
Next, create a function to initialize and render the chart:
typescript function createBarChart() { const ctx = document.getElementById('myChart') as HTMLCanvasElement; const data = { labels: ['January', 'February', 'March', 'April', 'May'], datasets: [{ label: 'Monthly Sales', data: [65, 59, 80, 81, 56], backgroundColor: 'rgba(75, 192, 192, 0.2)', borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1 }] }; const options = { scales: { y: { beginAtZero: true } } }; const myChart = new Chart(ctx, { type: 'bar', data: data, options: options }); } createBarChart();
This code sets up a simple bar chart with some sample data. You can customize it further according to your data and design preferences.
3.4. Compile TypeScript
Before you can view your chart, you need to compile your TypeScript code into JavaScript. Run the following command in your project directory:
shell tsc
This will generate an app.js file from your app.ts code.
3.5. View Your Chart
Open your index.html file in a web browser, and you should see your stunning TypeScript bar chart in action.
4. Enhancing Your Charts with TypeScript
Now that you’ve created a basic chart, let’s explore how TypeScript can be used to enhance the interactivity and functionality of your data visualizations.
4.1. Adding User Interaction
Interactive charts can provide a more engaging experience for your audience. TypeScript makes it easy to add interactivity to your charts. For example, you can add tooltips to display data values when users hover over chart elements or implement zooming and panning for large datasets.
4.2. Real-time Updates
In many scenarios, your data might change over time. TypeScript’s ability to handle real-time updates can be invaluable. You can set up WebSocket connections or fetch data from APIs, and TypeScript will help you seamlessly update your charts without compromising type safety.
4.3. Custom Animations
Animating chart transitions and updates can make your data visualizations more visually appealing. TypeScript’s support for custom animations allows you to create unique and eye-catching effects.
4.4. Accessibility
Accessibility is a crucial aspect of data visualization. TypeScript, when combined with HTML5 and ARIA attributes, can help ensure that your charts are accessible to all users, including those with disabilities.
5. TypeScript and D3.js for Advanced Data Visualizations
While Chart.js is an excellent choice for simple charts, you might encounter scenarios where you need more advanced and customized visualizations. In such cases, combining TypeScript with D3.js (Data-Driven Documents) is a powerful approach.
5.1. Install D3.js
To use D3.js in your TypeScript project, install it as a dependency:
shell npm install --save d3
5.2. Create a D3.js Chart
Here’s a brief example of how to create a simple scatter plot using D3.js and TypeScript:
typescript import * as d3 from 'd3'; function createScatterPlot() { const svg = d3.select('#myChart'); const data = [ { x: 1, y: 3 }, { x: 2, y: 5 }, { x: 3, y: 8 }, { x: 4, y: 12 }, { x: 5, y: 18 } ]; const xScale = d3.scaleLinear() .domain([0, d3.max(data, d => d.x)!]) .range([0, 300]); const yScale = d3.scaleLinear() .domain([0, d3.max(data, d => d.y)!]) .range([300, 0]); svg.selectAll('circle') .data(data) .enter() .append('circle') .attr('cx', d => xScale(d.x)) .attr('cy', d => yScale(d.y)) .attr('r', 5); } createScatterPlot();
This code creates a scatter plot using D3.js, which offers extensive customization options for creating unique and complex visualizations.
Conclusion
In this blog, we’ve explored how TypeScript can be a powerful tool for creating stunning data visualizations and charts. Its strong typing, IDE support, and scalability make it an excellent choice for both simple and complex projects. Whether you’re using libraries like Chart.js for quick and elegant charts or diving into the world of D3.js for advanced visualizations, TypeScript’s capabilities will help you bring your data to life in a way that captivates your audience.
Data visualization is not just about displaying numbers; it’s about telling a story. With TypeScript, you have the tools to craft compelling narratives through your data, making your charts not only informative but also visually stunning.
So, roll up your sleeves, start coding, and watch your data come to life with TypeScript-powered data visualizations that leave a lasting impression.
What are your thoughts on TypeScript and data visualization? Share your experiences and insights in the comments below!
Table of Contents
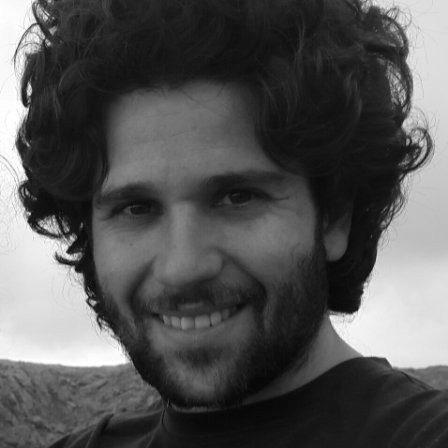
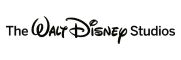