Debugging TypeScript in Visual Studio Code
Debugging is an integral part of the development process, allowing developers to identify and fix errors in their code efficiently. When working with TypeScript, a statically typed superset of JavaScript, having a powerful and reliable debugging environment is crucial. Visual Studio Code (VS Code), a popular code editor, offers robust features for debugging TypeScript applications, making the debugging process smoother and more productive. In this blog post, we’ll dive into various techniques, tools, and best practices for debugging TypeScript in Visual Studio Code.
Table of Contents
1. Setting Up Your TypeScript Project in Visual Studio Code
Before diving into debugging, you need to have your TypeScript project set up in Visual Studio Code.
1.1. Installing TypeScript and Node.js
Ensure you have Node.js installed on your system. You can download it from the official Node.js website. Once Node.js is installed, you can install TypeScript globally using npm:
bash npm install -g typescript
1.2. Configuring tsconfig.json
A tsconfig.json file is crucial for configuring your TypeScript project. It specifies compiler options and other project-specific settings. Create a tsconfig.json file in your project’s root directory and configure it according to your project’s needs. This configuration file helps VS Code understand your project’s structure and compile TypeScript files correctly.
2.2. Basic Debugging Workflow
Visual Studio Code offers a user-friendly interface for debugging TypeScript code. Here’s a basic debugging workflow:
2.1. Setting Breakpoints
Breakpoints allow you to pause the execution of your code at a specific line, giving you the opportunity to inspect variables and the program’s state at that point. To set a breakpoint, simply click on the left margin of the code editor next to the line number where you want the breakpoint. A red dot will appear, indicating that a breakpoint is set.
2.2. Running the Debugger
- Open the TypeScript file you want to debug.
- Make sure your launch.json file (located in the .vscode directory) is configured properly. This file specifies the debugging settings for your project.
- Click the “Run and Debug” button in the left sidebar or press F5.
- Choose the appropriate configuration from the dropdown if prompted.
- Your application will start running, and execution will pause at the breakpoints you’ve set.
2.3. Inspecting Variables
While debugging, you can inspect the values of variables, objects, and expressions. In the “Variables” section of the debug sidebar, you can see a list of variables in the current scope. You can hover over variables in your code to see their values, or you can add them to your watch list for continuous monitoring.
3.3. Advanced Debugging Techniques
As you become more comfortable with the basic debugging workflow, you can explore advanced techniques to enhance your debugging experience.
3.1. Conditional Breakpoints
Sometimes, you might want your breakpoint to be hit only when a certain condition is met. In VS Code, you can set conditional breakpoints by right-clicking on a breakpoint and adding a condition.
3.2. Watch Expressions
Watch expressions allow you to evaluate complex expressions and variables while debugging. You can add watch expressions to the “Watch” section of the debug sidebar. These expressions will be evaluated each time the debugger hits a breakpoint.
3.3. Call Stack Navigation
The call stack shows you the sequence of function calls that led to the current point in your code. This can be immensely helpful in understanding the flow of your program. You can navigate through the call stack in the debug sidebar and see the local variables for each function call.
4. Using Debugging Extensions
Visual Studio Code’s extensibility allows developers to enhance their debugging experience through various extensions. Here are a couple of popular extensions:
4.1. Augury for Angular Apps
If you’re developing Angular applications, the Augury extension provides powerful debugging tools specifically tailored for Angular projects. It helps you inspect components, their state, and the structure of your application’s UI.
4.2. Redux DevTools Extension
For projects using Redux for state management, the Redux DevTools extension provides an excellent debugging toolset. It allows you to track state changes, actions, and dispatches in a clear and organized manner.
5. Handling Common Debugging Scenarios
Debugging TypeScript applications can sometimes involve specific challenges. Here’s how to handle some common scenarios:
5.1. Asynchronous Code Debugging
Debugging asynchronous code can be tricky, as breakpoints might not pause execution at the desired point. To address this, use async/await and ensure your breakpoints are set within the asynchronous functions.
5.2. Handling Exceptions
When an exception occurs, the debugger can help you identify the source of the problem. Make use of the call stack and inspect variables to pinpoint where the exception originated.
5.3. Debugging in Browser
For web applications, you can debug TypeScript code running in a browser directly from VS Code. Utilize tools like the “Debugger for Chrome” extension, which allows you to debug TypeScript code that’s transpiled to JavaScript and executed in a Chrome browser.
Conclusion
Debugging TypeScript in Visual Studio Code empowers developers to catch and resolve issues efficiently, leading to higher-quality code and faster development cycles. By mastering the techniques outlined in this blog post, you’ll be equipped to tackle a wide range of debugging challenges in your TypeScript projects. Remember that debugging is not just about fixing bugs; it’s an opportunity to deeply understand your code’s behavior and improve your overall development skills. So, leverage the power of Visual Studio Code’s debugging capabilities and enhance your TypeScript development journey. Happy debugging!
Table of Contents
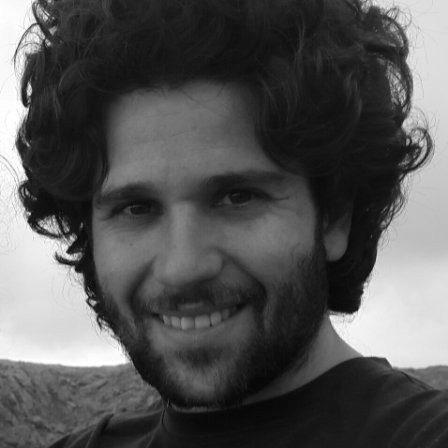
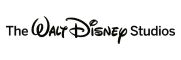