TypeScript and DevOps: Automating Your Workflow
In today’s fast-paced software development landscape, efficiency is key. Developers are constantly seeking ways to streamline their workflows and reduce manual tasks to focus on what matters most: writing high-quality code. This is where TypeScript and DevOps come into play. TypeScript, a statically typed superset of JavaScript, offers enhanced code quality and maintainability, while DevOps practices provide automation and collaboration to accelerate the development cycle. In this blog, we’ll explore how you can combine TypeScript and DevOps to automate your workflow, boost productivity, and deliver better software faster.
Table of Contents
1. Why TypeScript?
TypeScript is a statically typed superset of JavaScript that offers several advantages for developers. By adding type annotations to your code, you catch errors at compile time rather than runtime, which leads to more robust and maintainable software. Additionally, TypeScript provides excellent tooling, including code completion and refactoring support, making it easier to write and maintain code.
2. The Power of DevOps
DevOps is a set of practices that combines development and IT operations to automate and streamline the software delivery process. DevOps emphasizes collaboration, automation, and continuous improvement. By adopting DevOps principles, you can reduce manual intervention, shorten development cycles, and ensure a more reliable release process.
3. Setting Up Your TypeScript Environment
Before diving into the world of automation, you need to set up your TypeScript development environment. Here are the essential steps:
3.1. Installing Node.js and npm
TypeScript relies on Node.js and npm (Node Package Manager) to manage packages and dependencies. To get started, download and install Node.js from the official website. Once installed, npm will be available in your command line. Verify the installations by running the following commands:
bash node -v npm -v
3.2. Creating a TypeScript Project
To create a TypeScript project, start by creating a directory for your project and navigating to it in your terminal. Then, run the following command to initialize a new Node.js project:
bash npm init -y
This command generates a package.json file with default settings. Next, install TypeScript as a development dependency:
bash npm install typescript --save-dev
Now, you can create a TypeScript configuration file named tsconfig.json in your project’s root directory. You can either generate a basic tsconfig.json file with TypeScript’s tsc command or create one manually. Here’s a minimal tsconfig.json:
json { "compilerOptions": { "target": "ESNext", "module": "CommonJS", "outDir": "./dist", "rootDir": "./src" } }
This configuration tells TypeScript to compile your TypeScript files (.ts) from the src directory into JavaScript files (.js) in the dist directory.
4. Integrating TypeScript with Version Control
Now that your TypeScript environment is set up, it’s time to integrate it with version control, typically Git. Proper version control is crucial for collaboration and tracking changes in your codebase.
4.1. Initializing Git
If you haven’t already, install Git and initialize a Git repository in your project directory:
bash git init
4.2. Creating a .gitignore File
To avoid committing unnecessary files and directories, create a .gitignore file and specify the files and directories you want Git to ignore. Here’s a sample .gitignore file for a TypeScript project:
gitignore # Ignore node_modules directory node_modules/ # Ignore compiled JavaScript files dist/ # Ignore editor-specific files .vscode/ .idea/
Now that your TypeScript project is under version control, you can start tracking changes and collaborating with your team more effectively.
5. Automating Code Builds with Continuous Integration (CI)
Continuous Integration (CI) is a fundamental DevOps practice that involves automatically building and testing your code whenever changes are pushed to a version control repository. This ensures that your codebase remains functional and bug-free.
5.1. Choosing a CI/CD Platform
Several CI/CD platforms are available, such as Travis CI, Jenkins, CircleCI, and GitHub Actions. Choose one that integrates seamlessly with your version control system and meets your project’s requirements.
For this example, we’ll use GitHub Actions, which is tightly integrated with GitHub repositories.
5.2. Configuring a CI Pipeline
To set up a CI pipeline with GitHub Actions, create a .github/workflows directory in your project’s root directory and add a YAML configuration file, such as ci.yml. Here’s a sample configuration for building and testing a TypeScript project:
yaml name: CI on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v2 - name: Setup Node.js uses: actions/setup-node@v2 with: node-version: '14' - name: Install dependencies run: npm install - name: Build and test run: npm run build && npm test
This configuration file specifies that the CI pipeline should trigger on pushes to the main branch. It checks out the code, sets up Node.js, installs project dependencies, and finally builds and tests the code.
With this CI pipeline in place, every push to the main branch will automatically trigger a build and test process, providing rapid feedback on code changes.
6. Testing and Code Quality
Testing is a crucial aspect of software development that ensures your code functions as expected. TypeScript encourages writing tests by providing strong typing and tooling support for testing frameworks like Jest.
6.1. Writing Tests with TypeScript
To write tests for your TypeScript code, follow these steps:
Install Jest as a development dependency:
bash npm install jest @types/jest --save-dev
Create a test file, typically with a .spec.ts extension, alongside your source code files.
Write test cases using Jest’s testing functions and TypeScript’s type annotations.
Here’s a simple example:
typescript // math.ts export function add(a: number, b: number): number { return a + b; } // math.spec.ts import { add } from './math'; test('add function adds two numbers', () => { expect(add(1, 2)).toBe(3); });
Run your tests using the npm test command.
6.2. Running Tests Automatically
To automate test execution during development, you can use tools like jest-watch-typeahead or ts-jest to watch for file changes and run tests automatically.
bash npm install jest-watch-typeahead --save-dev
6.3. Static Code Analysis
Static code analysis tools like ESLint and TSLint help maintain code quality by identifying potential issues and enforcing coding standards. TypeScript integrates seamlessly with these tools.
6.3.1. Installing ESLint for TypeScript
bash npm install eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin --save-dev
6.3.2. Creating an ESLint Configuration
Create an ESLint configuration file, .eslintrc.js:
javascript module.exports = { parser: '@typescript-eslint/parser', plugins: ['@typescript-eslint'], extends: ['eslint:recommended', 'plugin:@typescript-eslint/recommended'], rules: { // Your custom rules here }, };
You can customize the ESLint configuration to suit your project’s coding style and requirements.
7. Automating Deployment with Continuous Deployment (CD)
Continuous Deployment (CD) automates the process of deploying your application to production or other environments. CD pipelines ensure that code changes are deployed reliably and consistently.
7.1. CD Strategies
Before automating deployment, you must decide on a deployment strategy. Common strategies include:
- Blue-Green Deployment: Two identical environments (blue and green) run concurrently. You deploy changes to one environment while keeping the other live. This allows for easy rollbacks.
- Canary Deployment: You release changes to a small subset of users to gather feedback and ensure stability before deploying to the entire user base.
- Feature Toggles: You deploy new features but keep them hidden behind feature toggles until they are ready for release.
7.2. Deployment Automation
Automating deployment can be achieved using various tools and services, such as Docker, Kubernetes, and serverless platforms like AWS Lambda.
For example, if you’re using AWS Lambda, you can set up deployment automation by creating AWS CloudFormation templates or using serverless frameworks like the Serverless Framework or AWS CDK.
8. Monitoring and Logging
Monitoring and logging are essential for identifying issues and performance bottlenecks in your application. DevOps practices include setting up monitoring tools and centralizing logs for easy analysis.
8.1. Setting Up Monitoring Tools
Tools like Prometheus, Grafana, and New Relic can provide valuable insights into your application’s performance and health. Integrate these tools into your deployment pipeline to monitor metrics and receive alerts when issues arise.
8.2. Log Aggregation
Centralized log aggregation services like the ELK Stack (Elasticsearch, Logstash, and Kibana) or cloud-based solutions like AWS CloudWatch Logs can help you gather, analyze, and visualize logs from different parts of your application.
By setting up monitoring and logging, you can proactively address issues, improve application performance, and ensure a seamless user experience.
Conclusion
In this blog post, we’ve explored how TypeScript and DevOps can supercharge your development workflow by automating key aspects of the software development lifecycle. By following the steps outlined in this guide, you can:
- Set up a TypeScript development environment for robust and maintainable code.
- Integrate version control with Git to collaborate effectively.
- Implement Continuous Integration (CI) to automate code builds and testing.
- Write tests and perform static code analysis to ensure code quality.
- Automate deployment with Continuous Deployment (CD) strategies.
- Set up monitoring and logging for better visibility into your application.
By combining TypeScript and DevOps practices, you’ll be well-equipped to deliver high-quality software faster and more efficiently, all while maintaining a streamlined and automated workflow. Embrace these practices, and watch your development process soar to new heights.
Table of Contents
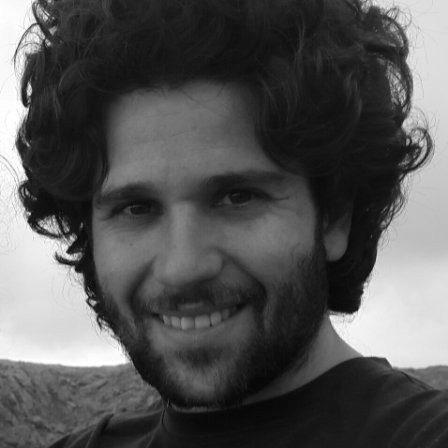
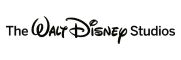