Error Handling in TypeScript: Best Practices
In software development, errors and bugs are inevitable. As developers, we need to be prepared to handle errors gracefully and ensure our applications can recover from unexpected situations. TypeScript, being a statically typed superset of JavaScript, provides powerful features that can aid in effective error handling. In this blog, we will explore the best practices for error handling in TypeScript, covering strategies, tools, and code samples to build robust and reliable applications.
1. Understanding Error Handling in TypeScript
1.1 What are Errors?
In the context of programming, an error is an unintended deviation or an exceptional condition that occurs during the execution of a program. These errors can arise due to various reasons, such as invalid input, network failures, or unexpected behavior of third-party libraries.
1.2 The Importance of Error Handling
Ignoring errors or handling them poorly can lead to disastrous consequences for our applications. Crashes, unexpected behaviors, and security vulnerabilities can be the result of improper error handling. Effective error handling can significantly improve the user experience, as it allows applications to gracefully handle problems and provide helpful feedback.
2. Built-in Error Types in TypeScript
TypeScript provides several built-in error types that we can use to represent different kinds of errors. Understanding these error types helps us to choose the appropriate one for our use case.
2.1 Error
The generic Error class in TypeScript represents an error that occurs during the runtime of a program. It includes a message property, which provides a human-readable description of the error.
typescript function divide(a: number, b: number): number { if (b === 0) { throw new Error("Division by zero is not allowed."); } return a / b; } try { const result = divide(10, 0); } catch (error) { console.error(error.message); }
2.2 TypeError
The TypeError class is a specific type of error that occurs when an operation is performed on a value of an inappropriate type.
typescript function greet(name: string) { if (typeof name !== "string") { throw new TypeError("Name must be a string."); } console.log(`Hello, ${name}!`); } try { greet(42); } catch (error) { console.error(error.message); }
2.3 RangeError
A RangeError is thrown when a numeric value is not within the expected range or set of allowed values.
typescript function getValidAge(age: number): number { if (age < 0 || age > 120) { throw new RangeError("Age must be between 0 and 120."); } return age; } try { const age = getValidAge(150); } catch (error) { console.error(error.message); }
2.4 Custom Errors
While TypeScript provides built-in error types, sometimes it’s beneficial to create custom error classes to represent specific application-related errors. Custom errors can provide more context and information about the issue, making it easier to handle them appropriately.
typescript class DatabaseError extends Error { constructor(message: string) { super(message); this.name = "DatabaseError"; } } function queryDatabase() { // Simulating a database error throw new DatabaseError("Failed to connect to the database."); } try { queryDatabase(); } catch (error) { if (error instanceof DatabaseError) { console.error(`Database Error: ${error.message}`); } else { console.error("An unexpected error occurred."); } }
3. Error Handling Strategies
To handle errors effectively in TypeScript, we can use various strategies depending on the situation and complexity of our application.
3.1 Try-Catch Blocks
The most common error handling mechanism is the use of try-catch blocks. We place the code that might throw an error inside the try block and catch the error with the catch block.
typescript try { // Code that might throw an error // For example, API calls, file operations, etc. } catch (error) { // Handle the error here console.error(error.message); }
Using try-catch blocks is particularly useful when dealing with synchronous code. However, when it comes to asynchronous operations, we need to take a different approach.
3.2 Error Objects and Stack Traces
When handling errors, it’s essential to capture and log the stack trace. The stack trace provides a snapshot of the call stack at the time of the error, helping developers trace the source of the error and debug it effectively.
typescript function performComplexTask() { try { // Code that might throw an error } catch (error) { console.error(error); console.error(error.stack); } }
3.3 Error Codes and Messages
Using error codes and custom error messages can significantly improve error handling. Instead of relying solely on error messages, error codes provide a structured way to identify errors and act accordingly.
typescript enum ErrorCode { DivisionByZero = "DIVISION_BY_ZERO", InvalidInput = "INVALID_INPUT", DatabaseError = "DATABASE_ERROR", } function divide(a: number, b: number): number { if (b === 0) { const error = new Error("Division by zero is not allowed."); error.code = ErrorCode.DivisionByZero; throw error; } return a / b; } try { const result = divide(10, 0); } catch (error) { if (error.code === ErrorCode.DivisionByZero) { console.error("Division by zero error occurred."); } else { console.error("An unexpected error occurred."); } }
3.4 Logging Errors
Proper logging of errors is crucial for monitoring and debugging applications in production environments. Using libraries like winston or log4js can simplify error logging and provide valuable insights into the application’s health.
typescript import winston from "winston"; const logger = winston.createLogger({ level: "error", format: winston.format.simple(), transports: [new winston.transports.Console()], }); function fetchData() { try { // Code that might throw an error } catch (error) { logger.error(error.message, { stack: error.stack }); } }
4. Asynchronous Error Handling
Asynchronous code introduces a new layer of complexity for error handling. Promises and async/await are common patterns in TypeScript for handling asynchronous operations.
4.1 Promises and Async/Await
typescript function fetchData(): Promise<any> { return new Promise((resolve, reject) => { // Simulating an API call setTimeout(() => { const data = { name: "John Doe", age: 30 }; if (data) { resolve(data); } else { reject(new Error("Failed to fetch data.")); } }, 1000); }); } async function getData() { try { const result = await fetchData(); console.log(result); } catch (error) { console.error(error.message); } } getData();
4.2 Handling Errors in Promises
When using promises, we can use the .then() and .catch() methods to handle the resolved value and any errors that occur during the promise’s execution.
typescript fetchData() .then((data) => console.log(data)) .catch((error) => console.error(error.message));
4.3 Error Boundaries in Async Functions
When working with multiple asynchronous tasks, handling errors for each task individually can become cumbersome. Error boundaries help us handle errors at a higher level and prevent them from propagating throughout the application.
typescript async function fetchData() { // Simulating multiple asynchronous tasks const task1 = fetchUserData(); const task2 = fetchUserPosts(); try { const userData = await task1; const userPosts = await task2; console.log(userData, userPosts); } catch (error) { console.error("Error occurred while fetching data."); } }
5. Properly Documenting Errors
Clear and accurate documentation is essential for maintaining and collaborating on projects. When it comes to error handling, proper documentation helps other developers understand the potential errors that can occur in a function or module.
5.1 JSDoc Annotations
Using JSDoc annotations, we can describe the types of errors that a function may throw and provide additional details about error handling.
typescript /** * Divide two numbers. * @param {number} a - The dividend. * @param {number} b - The divisor. * @returns {number} The result of the division. * @throws {Error} If the divisor is zero. */ function divide(a: number, b: number): number { if (b === 0) { throw new Error("Division by zero is not allowed."); } return a / b; }
5.2 Type Annotations for Error Objects
TypeScript allows us to create custom error types using interfaces or classes. Adding type annotations to error objects can improve code readability and help developers understand the structure of an error.
typescript interface CustomError { message: string; code: number; } function throwError(): never { throw { message: "Custom error", code: 500 } as CustomError; } try { throwError(); } catch (error) { const customError = error as CustomError; console.error(`Error Code ${customError.code}: ${customError.message}`); }
6. Error Handling Tools in TypeScript
6.1 ESLint
ESLint is a widely used linter for TypeScript and JavaScript that helps enforce coding standards and best practices, including error handling. It can catch common errors, suggest improvements, and ensure consistent code across the project.
6.2 TSLint
While ESLint is becoming the de facto standard for linting TypeScript projects, TSLint is worth mentioning as it was the original linter for TypeScript. TSLint can still be found in legacy projects, and some developers might be more familiar with it.
6.3 Sentry.io
Sentry.io is an error monitoring platform that allows developers to track, prioritize, and fix errors in real-time. It supports TypeScript and integrates seamlessly with popular frameworks and tools.
Conclusion
Error handling is a critical aspect of writing reliable and robust applications in TypeScript. By understanding the various error types, implementing appropriate strategies, and using the right tools, developers can ensure their code can gracefully handle unexpected situations. Properly documenting errors and adhering to best practices will result in more maintainable and user-friendly applications.
Remember, error handling is not just about fixing bugs; it’s about providing a better experience for users and building trust in your application. So, embrace these best practices and make error handling a top priority in your TypeScript projects!
Table of Contents
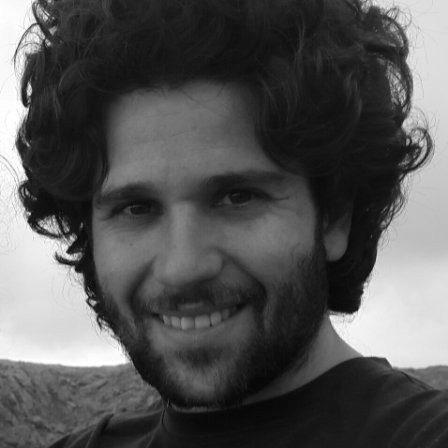
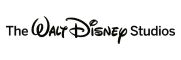