Mastering TypeScript: Essential Concepts and Techniques
TypeScript has gained immense popularity among developers due to its ability to add static typing to JavaScript, making it more robust and scalable. With its numerous features and strong typing system, TypeScript offers developers a powerful tool for building large-scale applications. Whether you’re a beginner or an experienced developer looking to level up your TypeScript skills, this comprehensive guide will take you through the essential concepts and techniques needed to master TypeScript.
1. Introduction to TypeScript:
Before diving into the core concepts of TypeScript, it’s important to understand its purpose and benefits. TypeScript is a statically typed superset of JavaScript that adds optional static typing, interfaces, classes, and other advanced features to the JavaScript language. It offers benefits like improved code maintainability, early detection of errors, and enhanced tooling support.
2. Type Annotations:
Type annotations allow you to specify the types of variables, function parameters, and return values in your TypeScript code. This section covers basic types such as numbers, strings, booleans, as well as more complex types like objects, arrays, and functions.
2.1 Basic Types:
typescript let age: number = 25; let name: string = "John Doe"; let isStudent: boolean = true;
2.2 Object Types:
typescript let person: { name: string, age: number } = { name: "John Doe", age: 25 };
2.3 Function Types:
typescript let greet: (name: string) => void = (name) => { console.log(`Hello, ${name}!`); };
2.4 Union and Intersection Types:
typescript let result: number | string = 10; result = "success";
3. Interfaces and Classes:
Interfaces and classes are powerful tools for building structured and reusable code in TypeScript. This section explains how to create interfaces, implement them in classes, and extend interfaces to define additional properties and methods.
3.1 Creating Interfaces:
typescript interface Shape { getArea(): number; } interface Rectangle extends Shape { width: number; height: number; } class Square implements Rectangle { constructor(public sideLength: number) {} getArea(): number { return this.sideLength ** 2; } }
3.2 Implementing Interfaces:
typescript interface Animal { makeSound(): void; } class Dog implements Animal { makeSound(): void { console.log("Woof!"); } }
3.3 Extending Interfaces:
typescript interface Shape { color: string; } interface Square extends Shape { sideLength: number; } let square: Square = { color: "red", sideLength: 5, };
3.4 Classes and Inheritance:
typescript class Vehicle { protected brand: string; constructor(brand: string) { this.brand = brand; } getBrand(): string { return this.brand; } } class Car extends Vehicle { private model: string; constructor(brand: string, model: string) { super(brand); this.model = model; } getModel(): string { return this.model; } }
4. Generics:
Generics provide a way to create reusable components that can work with different data types. This section explores generic functions and classes, enabling you to write more flexible and generic code.
4.1 Introduction to Generics:
typescript function identity<T>(arg: T): T { return arg; } let result = identity<string>("Hello, TypeScript!");
4.2 Generic Functions:
typescript function printArray<T>(arr: T[]): void { arr.forEach((item) => { console.log(item); }); }
4.3 Generic Classes:
typescript class Box<T> { private contents: T; constructor(contents: T) { this.contents = contents; } getContents(): T { return this.contents; } }
5. Advanced TypeScript Features:
This section covers advanced features of TypeScript, including type inference, type guards, type assertions, decorators, modules, and namespaces. Understanding these features will empower you to write more concise and expressive code.
5.1 Type Inference:
TypeScript’s type inference automatically infers the types of variables based on their usage, reducing the need for explicit type annotations.
5.2 Type Guards and Type Assertions:
Type guards allow you to narrow down the type of a variable within a conditional block, while type assertions enable you to override the inferred type of a variable.
5.3 Decorators:
Decorators are a powerful feature in TypeScript, enabling you to modify the behavior of classes, methods, and properties at runtime.
5.4 Modules and Namespaces:
TypeScript provides modules and namespaces to organize and encapsulate code. Modules help in creating reusable code, while namespaces prevent naming collisions.
6. Working with TypeScript in Real-World Projects:
This section provides practical guidance on setting up TypeScript projects, integrating TypeScript with popular frameworks like React and Node.js, and optimizing the TypeScript development workflow.
6.1 Setting Up a TypeScript Project:
Setting up a TypeScript project involves configuring the TypeScript compiler, setting up the project structure, and managing dependencies using package managers like npm or yarn.
6.2 Integrating TypeScript with Popular Frameworks:
TypeScript has excellent integration with popular frameworks like React, Angular, and Node.js, allowing you to build robust and type-safe applications.
6.3 TypeScript Tooling and Development Workflow:
TypeScript offers a rich ecosystem of tools and editors to enhance the development workflow, including features like auto-completion, static analysis, and code refactoring.
7. Best Practices and Tips for TypeScript Development:
This section shares best practices and tips for TypeScript development, including avoiding common pitfalls, using TypeScript with JavaScript libraries, and testing and debugging TypeScript applications effectively.
7.1 Avoiding Common Pitfalls:
Understanding common pitfalls in TypeScript, such as the difference between “undefined” and “null,” can help you write more reliable code.
7.2 Using TypeScript with JavaScript Libraries:
Learn how to leverage TypeScript’s declaration files or create your own type definitions to use JavaScript libraries seamlessly in your TypeScript projects.
7.3 Testing and Debugging TypeScript Applications:
Discover tools and techniques for testing and debugging TypeScript applications to ensure code correctness and catch errors early in the development process.
Conclusion:
In this comprehensive guide, we have covered the essential concepts and techniques required to master TypeScript. By understanding type annotations, interfaces, generics, and advanced features, you can write more robust, maintainable, and scalable code. Whether you’re a beginner or an experienced developer, TypeScript is a valuable skill to have in your toolkit for building modern web applications. So, start exploring TypeScript today and take your coding skills to the next level.
Table of Contents
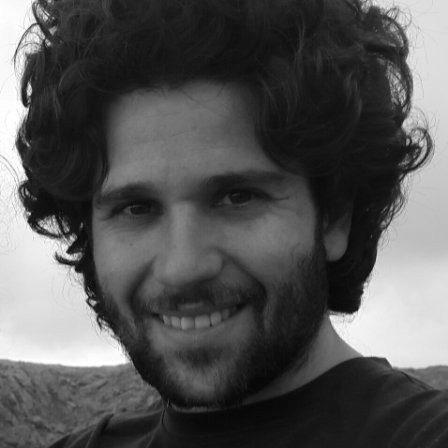
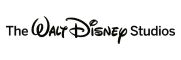