TypeScript and Game Development: Creating Interactive Experiences
In the world of game development, creating interactive and engaging experiences is the ultimate goal. To achieve this, developers need a versatile and powerful language that can handle complex logic while maintaining code readability and scalability. TypeScript, a statically typed superset of JavaScript, has emerged as a game-changer in this regard. In this blog, we will explore how TypeScript can be harnessed to create interactive experiences in game development.
Table of Contents
1. Why TypeScript for Game Development?
1.1. Strong Typing for Robust Code
One of the primary reasons TypeScript shines in game development is its strong typing system. Unlike JavaScript, where variable types can change dynamically, TypeScript enforces strict type checking at compile-time. This leads to fewer runtime errors and a more robust codebase.
Let’s take a look at an example of strong typing in TypeScript:
typescript // TypeScript function calculateDamage(playerHealth: number, enemyAttack: number): number { return playerHealth - enemyAttack; }
In this code snippet, we explicitly define the types of playerHealth and enemyAttack. If you attempt to pass a string or any other incompatible type, TypeScript will catch the error during compilation.
1.2. Enhanced Code Maintainability
Games are complex software projects that often involve large teams of developers. TypeScript’s static typing and the ability to define custom types and interfaces make codebase maintenance easier. Developers can clearly understand the structure of data and functions, reducing the chances of introducing bugs when making changes.
1.3. Code Navigation and Auto-completion
TypeScript integrates seamlessly with modern code editors like Visual Studio Code. This means you benefit from code navigation, intelligent auto-completion, and real-time error checking. These features significantly speed up the development process.
2. Setting Up Your TypeScript Game Development Environment
Before diving into game development with TypeScript, you need to set up your development environment. Here’s a step-by-step guide:
2.1. Install Node.js and npm
TypeScript is a superset of JavaScript and runs on the Node.js platform. Install Node.js and npm (Node Package Manager) if you haven’t already. You can download them from nodejs.org.
2.2. Install TypeScript
Once Node.js is installed, open your terminal and run the following command to install TypeScript globally:
bash npm install -g typescript
2.3. Create a New TypeScript Project
Now that TypeScript is installed, you can create a new TypeScript project. Create a directory for your project and navigate to it in the terminal. Then, run:
bash tsc --init
This command generates a tsconfig.json file, which contains TypeScript configuration options for your project.
2.4. Set Up a Build Process
To transpile TypeScript code into JavaScript, you need a build process. A popular choice is to use a bundler like Webpack or a task runner like Gulp. Configure your build process according to your project’s needs.
2.5. Install Game Development Libraries
Depending on your game’s requirements, you may need additional libraries and frameworks. Some popular choices for game development in TypeScript include Phaser, Three.js, and Babylon.js. Install these libraries using npm:
bash npm install phaser
2.6. Start Coding
With your environment set up, you’re ready to start coding your TypeScript game.
3. Creating Interactive Gameplay with TypeScript
Now that you have your development environment ready, let’s dive into creating interactive gameplay using TypeScript. We’ll use the Phaser game framework as an example.
3.1. Setting Up a Phaser Game
Phaser is a fast and robust 2D game framework for TypeScript. To create a basic Phaser game, follow these steps:
3.1.1. Import Phaser
First, import Phaser at the top of your TypeScript file:
typescript import * as Phaser from 'phaser';
3.1.2. Create a Game Configuration
Next, create a game configuration object that defines the game’s size, rendering context, and initial scene:
typescript const config: Phaser.Types.Core.GameConfig = { type: Phaser.AUTO, width: 800, height: 600, scene: { preload: preload, create: create, update: update } };
3.1.3. Initialize the Game
Initialize the game with the configuration object:
typescript const game = new Phaser.Game(config);
3.2. Creating Interactive Elements
Now that you have a basic game structure in place, let’s add some interactive elements. In Phaser, you can easily create sprites, add physics, and handle user input.
3.2.1. Creating a Player Sprite
typescript let player: Phaser.Physics.Arcade.Sprite; function preload() { // Load player sprite image this.load.image('player', 'assets/player.png'); } function create() { // Create player sprite player = this.physics.add.sprite(400, 300, 'player'); } function update() { // Add player movement logic here }
In this code, we load a player sprite image and create a player sprite at coordinates (400, 300). You can add movement logic and interactions in the update function.
3.3. Handling User Input
To make your game truly interactive, you need to handle user input. Phaser provides easy-to-use input handling functions:
typescript function update() { // Handle user input const cursors = this.input.keyboard.createCursorKeys(); if (cursors.left.isDown) { player.setVelocityX(-160); } else if (cursors.right.isDown) { player.setVelocityX(160); } else { player.setVelocityX(0); } }
In this example, we use Phaser’s input system to detect arrow key presses and move the player sprite accordingly.
4. Advanced TypeScript Techniques for Game Development
As your game development skills progress, you’ll encounter more complex scenarios that require advanced TypeScript techniques. Here are a few tips and techniques to consider:
4.1. Design Patterns
Consider using design patterns like the Singleton pattern for managing global game state or the Observer pattern for handling events and notifications within your game.
typescript // Singleton pattern example class GameManager { private static instance: GameManager; private constructor() { // Initialize game manager } public static getInstance(): GameManager { if (!GameManager.instance) { GameManager.instance = new GameManager(); } return GameManager.instance; } }
4.2. Type Safety with Custom Types
Define custom types and interfaces to ensure type safety throughout your codebase. For example, create a Player interface to represent player data:
typescript interface Player { name: string; health: number; score: number; }
4.3. State Management
Implement state management to handle different game states such as menus, gameplay, and game over screens. You can use finite state machines or state design patterns for this purpose.
4.4. Code Splitting
As your game project grows, consider code splitting techniques to keep your codebase manageable and maintainable. This involves breaking down your code into smaller modules that can be loaded on-demand.
Conclusion
TypeScript offers a powerful toolkit for creating interactive experiences in game development. Its strong typing, code maintainability, and integration with popular game frameworks make it an excellent choice for both beginners and experienced developers. Whether you’re building a 2D platformer or a 3D immersive world, TypeScript can help you bring your game ideas to life with confidence and ease. Start experimenting with TypeScript in your game development projects and unlock a world of possibilities. Happy coding!
Table of Contents
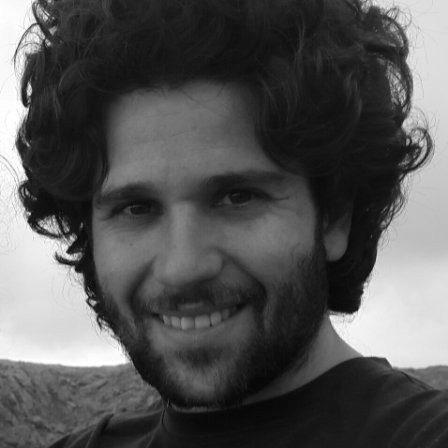
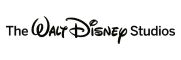