TypeScript Generics: Unlocking the Potential
TypeScript has gained immense popularity among developers due to its ability to add static typing to JavaScript. One of its most powerful features is generics, which provide a way to create reusable and flexible code. With generics, developers can write type-safe and reusable functions, classes, and interfaces, making their code more robust and maintainable. In this blog, we will delve into the world of TypeScript generics, exploring their capabilities, benefits, and best practices.
1. What are Generics?
Generics in TypeScript allow developers to create components that can work with a variety of types, providing flexibility and reusability. They enable the definition of functions, classes, and interfaces that can operate on different types without sacrificing type safety.
Generics are denoted by angle brackets (<>) and can accept one or more type parameters. These type parameters act as placeholders for specific types that will be provided when using the generic component. For example, Array<T> is a generic array type where T represents the type of elements in the array.
2. Benefits of Generics
2.1 Reusability and Flexibility
One of the primary advantages of generics is their ability to create reusable code. By using generics, developers can write functions, classes, and interfaces that are not tied to a specific type. This enables the creation of components that can work with different data types, reducing code duplication and improving productivity.
Consider a simple function to reverse an array:
typescript function reverseArray<T>(array: T[]): T[] { return array.reverse(); }
Here, the reverseArray function uses a generic type parameter T to represent the type of elements in the array. This allows the function to work with arrays of different types, such as numbers, strings, or custom objects.
typescript const numbers = [1, 2, 3, 4, 5]; const reversedNumbers = reverseArray(numbers); // [5, 4, 3, 2, 1] const names = ["Alice", "Bob", "Charlie"]; const reversedNames = reverseArray(names); // ["Charlie", "Bob", "Alice"]
The same function can be used with different types, showcasing the reusability and flexibility provided by generics.
2.2 Type Safety
TypeScript is known for its static type checking capabilities, and generics further enhance type safety. Generics allow developers to specify the expected type of arguments and return values in a generic component, ensuring that only compatible types are used.
For instance, consider a generic function that retrieves the first element from an array:
typescript function getFirstElement<T>(array: T[]): T | undefined { return array.length > 0 ? array[0] : undefined; }
The getFirstElement function takes an array of type T and returns either an element of type T or undefined. This ensures that the returned value matches the type of the array elements, providing type safety.
typescript const numbers = [1, 2, 3, 4, 5]; const firstNumber = getFirstElement(numbers); // Type: number const names = ["Alice", "Bob", "Charlie"]; const firstName = getFirstElement(names); // Type: string
In the above examples, the generic function infers the correct types based on the provided arrays, preventing any potential type errors at compile-time.
2.3 Code Readability and Maintainability
Generics can significantly enhance code readability and maintainability. By using generics, developers can write self-descriptive code that clearly communicates the expected types. This makes the code easier to understand, especially when working on larger projects or collaborating with other developers.
Generics also contribute to maintainability by reducing code duplication. Instead of writing similar functions or classes for each specific type, generics allow developers to create a single implementation that can handle multiple types. This reduces the amount of code that needs to be written and maintained.
3. Using Generics with Functions
Generics can be effectively used with functions to create reusable and type-safe code. Let’s explore some common patterns and techniques when working with generic functions.
3.1 Basic Usage
The simplest form of a generic function is where a single type parameter is used. Here’s an example of a generic identity function:
typescript function identity<T>(arg: T): T { return arg; }
The identity function takes an argument of type T and returns the same value. The type parameter T represents the type of the argument and the return value. This allows the function to preserve the type information.
typescript const numberResult = identity<number>(42); // Type: number const stringResult = identity<string>("Hello"); // Type: string
By explicitly specifying the type argument, the generic function ensures type safety and enables the TypeScript compiler to catch any type mismatches.
3.2 Constraint Types
Generics can be constrained to specific types or a subset of types using type constraints. This allows developers to define generic functions that work only with certain types or types that meet specific criteria.
typescript interface Printable { print(): void; } function printValue<T extends Printable>(value: T): void { value.print(); }
In the above example, the printValue function accepts a value of type T that extends the Printable interface. The function guarantees that the passed value has a print method, ensuring type safety.
typescript class Person implements Printable { print() { console.log("Printing person"); } } class Car { print() { console.log("Printing car"); } } const person = new Person(); const car = new Car(); printValue(person); // OK printValue(car); // OK printValue(42); // Error: Type 'number' does not satisfy the constraint 'Printable'
By using type constraints, we can ensure that only compatible types are passed to the function, preventing runtime errors.
3.3 Default Types
TypeScript allows providing default types for generic parameters, which can be useful when a generic type parameter is not explicitly specified.
typescript function defaultValue<T = string>(): T { return "default" as T; }
In the above example, the defaultValue function returns a default value of type T, which defaults to string if no type argument is provided.
typescript const result = defaultValue<number>(); // Type: number const defaultResult = defaultValue(); // Type: string
By providing a default type, the function becomes more convenient to use, as developers can rely on the default type when it fits their needs.
4. Leveraging Generics with Classes
Generics can also be applied to classes, enabling the creation of reusable and type-safe components. Let’s explore how generics can be used with classes and how constraints can be applied.
4.1 Creating a Generic Class
To create a generic class, the generic type parameters are declared at the class level. These parameters can then be used as types for properties, methods, or constructor arguments within the class.
typescript class Box<T> { private value: T; constructor(value: T) { this.value = value; } getValue(): T { return this.value; } }
In the above example, the Box class is defined as a generic class with a type parameter T. The constructor accepts a value of type T, which is then stored in the private value property. The getValue method returns the stored value of type T.
typescript const numberBox = new Box<number>(42); console.log(numberBox.getValue()); // Type: number const stringBox = new Box<string>("Hello"); console.log(stringBox.getValue()); // Type: string
By specifying the type argument when creating instances of the class, the generic class ensures type safety and preserves the type information.
4.2 Working with Constraints in Generic Classes
Similar to generic functions, generic classes can also have constraints to limit the types they can work with.
typescript interface Serializable { serialize(): string; } class Serializer<T extends Serializable> { serializeObject(obj: T): string { return obj.serialize(); } }
In the above example, the Serializer class is a generic class that accepts a type argument T constrained to types that implement the Serializable interface. The serializeObject method takes an object of type T and calls its serialize method.
typescript class Person implements Serializable { serialize() { return JSON.stringify(this); } } class Car { serialize() { return "Car object"; } } const personSerializer = new Serializer<Person>(); const carSerializer = new Serializer<Car>(); // Error: Type 'Car' does not satisfy the constraint 'Serializable'
By using constraints, the generic class ensures that only compatible types are used and prevents potential runtime errors.
5. Generic Interfaces
In addition to functions and classes, TypeScript generics can also be applied to interfaces. Generic interfaces allow the definition of interfaces that can be implemented by different types while maintaining type safety and code reusability.
typescript interface Repository<T> { getById(id: number): T | undefined; getAll(): T[]; create(item: T): void; update(item: T): void; delete(id: number): void; }
In the above example, the Repository interface is defined as a generic interface with a type parameter T. The interface provides common CRUD (Create, Read, Update, Delete) operations for working with a data store. The methods operate on items of type T.
typescript class User { // User implementation } class UserRepository implements Repository<User> { // Repository implementation for User type }
By implementing the Repository interface with a specific type argument, such as User, the UserRepository class ensures that it correctly handles User objects and provides the required operations.
6. Best Practices for Using Generics
To make the most of TypeScript generics, it’s essential to follow some best practices:
6.1 Use Descriptive Names
When defining generic types, choose descriptive names for the type parameters. This helps improve code readability and provides clarity on the intended purpose of the type parameter.
6.2 Avoid Overcomplicating Generics
While generics offer great flexibility, it’s important not to overcomplicate them. Use generics when they genuinely add value and make the code more reusable. Avoid unnecessary complexity that can make the code harder to understand.
6.3 Provide Default Types Where Applicable
In some cases, it may be helpful to provide default types for generic type parameters. This can make the usage of generics more convenient and reduce the need for explicit type arguments.
Conclusion
TypeScript generics unlock the potential for writing flexible, reusable, and type-safe code. By leveraging generics with functions, classes, and interfaces, developers can enhance code quality, improve maintainability, and reduce code duplication. Understanding the capabilities of generics and following best practices can significantly improve the development experience and enable the creation of robust TypeScript applications.
In this blog, we explored the fundamentals of TypeScript generics, their benefits, and best practices. Armed with this knowledge, you can now unlock the full potential of TypeScript generics in your projects and write more efficient and maintainable code. Happy coding!
Table of Contents
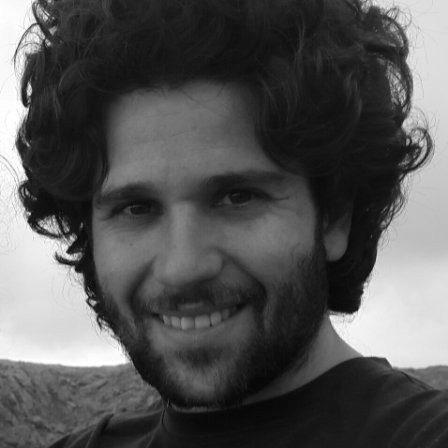
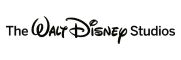