TypeScript: A Complete Guide for JavaScript Developers
TypeScript has emerged as a powerful tool for JavaScript developers, providing enhanced productivity, code maintainability, and improved tooling support. This comprehensive guide aims to introduce JavaScript developers to the world of TypeScript, covering key concepts, syntax, the type system, and advanced features. Whether you’re a seasoned JavaScript developer looking to level up your skills or a newcomer eager to learn, this guide will equip you with the knowledge to harness the full potential of TypeScript.
1. What is TypeScript?
1.1. JavaScript, but with Types
At its core, TypeScript is a superset of JavaScript that introduces static typing to the language. It allows developers to annotate variables, functions, and other constructs with types, enabling the detection of potential errors during development rather than at runtime. TypeScript compiles down to plain JavaScript, ensuring compatibility with all modern browsers and JavaScript runtime environments.
1.2. Advantages of Using TypeScript
TypeScript brings several advantages to JavaScript development:
- Enhanced Tooling Support: TypeScript provides powerful tools like autocompletion, intelligent code navigation, and refactoring support, making developers more productive.
- Early Error Detection: The static type system of TypeScript catches common errors at compile-time, preventing many runtime issues and reducing the debugging effort.
- Improved Code Maintainability: By adding explicit types, TypeScript code becomes more self-documenting and easier to understand, especially in large codebases. It also enables better collaboration among developers.
- Modern JavaScript Features: TypeScript allows developers to use the latest ECMAScript features even when the target environment doesn’t support them natively. It achieves this through a process called transpiling, which converts modern JavaScript syntax into backward-compatible code.
2. Setting Up a TypeScript Development Environment
Before diving into TypeScript, setting up a development environment is necessary. Follow these steps to get started:
2.1. Installing TypeScript
To install TypeScript, you can use npm (Node Package Manager) or yarn, depending on your preference. Open your terminal and run the following command:
bash npm install -g typescript
2.2. Configuring tsconfig.json
The tsconfig.json file contains the configuration options for the TypeScript compiler. Create a file named tsconfig.json in the root of your project and add the following content:
json { "compilerOptions": { "target": "ES2020", "module": "ESNext", "outDir": "dist", "strict": true }, "include": [ "src/**/*.ts" ], "exclude": [ "node_modules" ] }
Here, we specify the target ECMAScript version, the output directory, and enable strict type checking.
2.3. Integrating TypeScript with Popular Editors
Most popular code editors provide excellent support for TypeScript out of the box. Visual Studio Code, for instance, offers features like automatic error checking, code completion, and quick fixes. If you’re using another editor, consult its documentation for TypeScript integration instructions.
3. TypeScript Basics
Now that your development environment is set up, let’s explore the basics of TypeScript.
3.1. Variables and Types
In TypeScript, variables can have explicit types. For example:
typescript let message: string = "Hello, TypeScript!"; let count: number = 42; let isEnabled: boolean = true;
Here, we explicitly annotate the types of message, count, and isEnabled.
3.2. Functions and Arrow Functions
Functions in TypeScript can have parameter and return type annotations:
typescript function add(x: number, y: number): number { return x + y; }
const multiply = (x: number, y: number): number => x * y;
In the first example, add is a traditional function with explicit parameter and return types. In the second example, multiply is an arrow function with inferred return type.
3.3. Interfaces and Type Aliases
Interfaces allow us to define object shapes and their associated types:
typescript interface Person { name: string; age: number; } const john: Person = { name: "John Doe", age: 30, };
Here, we define an interface Person with name and age properties. The variable john conforms to this interface.
Type aliases provide a way to create reusable type definitions:
typescript type Point = [number, number]; const coordinates: Point = [10, 20];
In this example, Point is a type alias for a tuple representing coordinates.
3.4. Arrays and Tuples
Arrays in TypeScript can have a specific element type:
typescript const numbers: number[] = [1, 2, 3]; const fruits: Array<string> = ["apple", "banana", "orange"];
Here, numbers and fruits are arrays with specific element types.
Tuples represent fixed-length arrays with predefined types for each position:
typescript const user: [number, string] = [1, "John Doe"];
The user tuple has a number at the first position and a string at the second position.
With these basics in place, you’re ready to explore the more advanced features of TypeScript. In the next section, we’ll delve deeper into the TypeScript type system and discover its capabilities.
4. Understanding the TypeScript Type System
TypeScript’s type system is one of its key strengths, providing developers with the ability to express complex types and enforce strict type checking. Let’s explore some important concepts of the TypeScript type system.
4.1. Primitive Types and Literal Types
TypeScript includes all the primitive types of JavaScript, such as string, number, boolean, null, and undefined. Additionally, TypeScript introduces literal types, which allow you to specify exact values for variables. For example:
typescript let status: "success" | "failure"; status = "success"; // Valid status = "failure"; // Valid status = "pending"; // Invalid
In this example, the status variable can only be assigned the values “success” or “failure”. Any other value assignment will result in a compilation error.
4.2. Union and Intersection Types
Union types allow you to combine multiple types into one. For instance:
typescript let id: string | number; id = "ABC"; // Valid id = 123; // Valid id = true; // Invalid
In this case, the id variable can accept values of type string or number.
On the other hand, intersection types allow you to combine multiple types into a single type. For example:
typescript interface Shape { color: string; } interface Area { area: number; } type ColoredShape = Shape & Area; const square: ColoredShape = { color: "red", area: 100, };
Here, the ColoredShape type is an intersection of Shape and Area, meaning it must have properties from both interfaces.
4.3. Type Inference and Type Assertions
TypeScript has a powerful type inference mechanism that can infer types based on context. For example:
typescript let message = "Hello, TypeScript!"; // Type: string
In this case, TypeScript infers the type of the message variable as string based on the assigned value.
Type assertions allow you to explicitly override the inferred type or specify a more specific type. For instance:
typescript let length = (message as string).length; // Type: number
In this example, the type assertion (message as string) tells TypeScript to consider message as a string, allowing access to the length property.
4.4. Type Guards and Discriminated Unions
Type guards enable you to narrow down the type of a variable within a conditional block. For example:
typescript interface Circle { kind: "circle"; radius: number; } interface Rectangle { kind: "rectangle"; width: number; height: number; } type Shape = Circle | Rectangle; function calculateArea(shape: Shape): number { if (shape.kind === "circle") { return Math.PI * shape.radius ** 2; } else if (shape.kind === "rectangle") { return shape.width * shape.height; } }
In this scenario, the kind property acts as a discriminator, allowing TypeScript to determine the specific shape within the conditional blocks.
By utilizing type guards and discriminated unions, you can write more robust and type-safe code.
5. Advanced TypeScript Features
TypeScript provides several advanced features that extend the capabilities of JavaScript. Let’s explore some of these features.
5.1. Generics
Generics enable the creation of reusable components that can work with different types. For example:
typescript function identity<T>(arg: T): T { return arg; } const result = identity<number>(42); // Type: number
In this example, the identity function uses a type variable T to indicate that it can accept and return any type.
5.2. Enums
Enums allow you to define a set of named values. For instance:
typescript enum Direction { Up = "UP", Down = "DOWN", Left = "LEFT", Right = "RIGHT", } let userDirection: Direction = Direction.Up;
Here, the Direction enum defines four possible directions, and the userDirection variable can only have one of those values.
5.3. Decorators
Decorators provide a way to add metadata and modify the behavior of classes, properties, or methods. They are widely used in frameworks like Angular. For example:
typescript function log(target: any, propertyKey: string, descriptor: PropertyDescriptor) { const originalMethod = descriptor.value; descriptor.value = function (...args: any[]) { console.log(`Calling method ${propertyKey} with arguments ${args}`); return originalMethod.apply(this, args); }; return descriptor; } class Calculator { @log add(x: number, y: number): number { return x + y; } } const calc = new Calculator(); calc.add(2, 3); // Logs: Calling method add with arguments 2, 3
In this example, the log decorator logs the method name and arguments before invoking the original method.
5.4. Modules and Namespaces
TypeScript supports modules, allowing you to organize your code into separate files and create a more modular architecture. Modules can be used to encapsulate related functionality and promote code reuse.
Namespaces provide a way to group related classes, interfaces, and functions into a single namespace. They help avoid naming conflicts between different parts of your codebase.
6. Integrating Existing JavaScript Code
TypeScript’s compatibility with JavaScript is a significant advantage. You can seamlessly integrate existing JavaScript code into TypeScript projects.
6.1. Declaration Files
Declaration files (*.d.ts) provide type information for existing JavaScript libraries. These files describe the shape of the library’s API, allowing TypeScript to provide accurate type checking and editor support. Declaration files can be created manually or obtained from community-maintained repositories like DefinitelyTyped.
6.2. Type Definitions with DefinitelyTyped
DefinitelyTyped is a repository that hosts declaration files for thousands of JavaScript libraries. You can use tools like @types to automatically install the appropriate declaration files for the libraries you’re using. This way, you can leverage TypeScript’s benefits while working with popular JavaScript frameworks and libraries.
6.3. Working with Dynamic Libraries
When working with JavaScript libraries that don’t have type definitions, TypeScript allows you to use the any type to opt-out of type checking for those specific parts of your code. While this provides flexibility, it’s essential to use it sparingly and consider creating type definitions if possible.
7. TypeScript and Modern JavaScript Features
TypeScript supports many modern JavaScript features, even when the target runtime environment lacks native support. Let’s explore a few examples.
7.1. ECMAScript Modules
TypeScript supports ECMAScript modules, enabling you to use the import and export syntax to create modular code. This promotes better code organization and improves maintainability.
7.2. Optional Chaining and Nullish Coalescing
Optional chaining (?.) and nullish coalescing (??) are powerful operators introduced in recent versions of JavaScript. TypeScript allows you to use these operators even when targeting older JavaScript versions, thanks to its transpilation capabilities.
7.3. Async/Await and Promises
TypeScript has excellent support for working with asynchronous operations using async/await syntax. It provides type inference for promises and helps catch common mistakes when working with asynchronous code.
7.4. Class Properties and Private Fields
TypeScript allows you to declare class properties directly in the constructor parameters, reducing boilerplate code. Additionally, it supports private fields, providing encapsulation and better class privacy.
8. Debugging and Tooling Support
TypeScript integrates seamlessly with popular development tools, providing an enhanced developer experience.
8.1. Debugging TypeScript Code
When debugging TypeScript code, most modern browsers and development tools understand source maps generated by TypeScript. This allows you to set breakpoints, inspect variables, and step through your TypeScript code directly.
8.2. Type Checking and Compiler Options
TypeScript offers a wide range of compiler options that allow you to customize the behavior of the TypeScript compiler. You can enforce stricter type checking, adjust the ECMAScript target version, enable strict null checks, and more.
8.3. Linting and Code Formatting
TypeScript integrates well with popular linting and formatting tools like ESLint and Prettier. These tools help enforce consistent coding styles, catch potential errors, and improve code readability.
9. Building and Deploying TypeScript Projects
To deploy a TypeScript project, you need to compile TypeScript code into JavaScript that can be executed by the target runtime environment.
9.1. Compiling TypeScript Code
Compiling TypeScript code can be done using the TypeScript compiler (tsc) or build tools like Webpack or Rollup. These tools take care of the compilation process, generating the necessary JavaScript files and ensuring compatibility.
9.2. Bundling and Minification
When deploying TypeScript projects, bundling tools like Webpack or Rollup can combine multiple JavaScript files into a single bundle. Additionally, minification techniques can be applied to reduce the bundle size for better performance.
9.3. Optimizing TypeScript for Production
To optimize TypeScript code for production, you can enable the –prod flag during the build process. This enables additional optimizations like dead code elimination, tree shaking, and minification, resulting in smaller and faster JavaScript bundles.
10. TypeScript and the Future of JavaScript
TypeScript has gained significant popularity among JavaScript developers and continues to evolve alongside the JavaScript ecosystem.
10.1. ECMAScript Proposals and Compatibility
TypeScript actively incorporates new ECMAScript proposals, allowing developers to experiment with upcoming JavaScript features before they are fully standardized and supported by browsers.
10.2. The Evolution of TypeScript
TypeScript is continuously improving and adding new features. The TypeScript team actively engages with the community to understand developers’ needs and provide a more robust and productive development experience.
Conclusion
TypeScript offers a wide range of benefits to JavaScript developers, including enhanced tooling support, early error detection, improved code maintainability, and compatibility with modern JavaScript features. By leveraging TypeScript’s static type system and advanced features, developers can write more robust, scalable, and maintainable code. Whether you’re a JavaScript developer looking to level up your skills or a newcomer eager to learn, embracing TypeScript can significantly enhance your JavaScript development experience.
Table of Contents
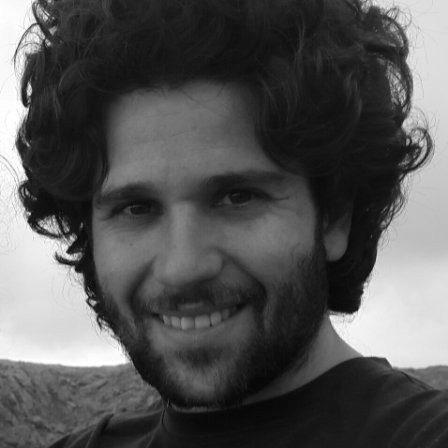
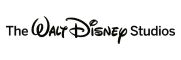