TypeScript and Image Processing: Transforming Visual Data
In the ever-evolving world of web development, TypeScript has gained widespread popularity for its strong typing and scalability features. One fascinating application of TypeScript is in the realm of image processing, where it can be leveraged to manipulate and transform visual data. In this blog post, we will embark on a journey to uncover the seamless integration of TypeScript with image processing, showcasing its potential and utility.
Table of Contents
1. Why TypeScript for Image Processing?
Before delving into the nitty-gritty of image processing with TypeScript, let’s take a moment to understand why TypeScript is an excellent choice for this task.
1.1. Strong Typing for Safety
TypeScript’s greatest strength lies in its static typing system, which helps catch errors at compile-time rather than runtime. When working with visual data, ensuring data integrity is crucial. Strong typing guarantees that you are manipulating images with the expected data types, reducing the chances of runtime errors significantly.
1.2. Scalability and Maintainability
As image processing tasks can quickly become complex, TypeScript’s scalability and maintainability features come in handy. TypeScript allows you to write modular and organized code, making it easier to manage and extend your image processing pipelines as your project grows.
1.3. Wide Ecosystem and Tooling
TypeScript’s vast ecosystem includes libraries, frameworks, and tools that seamlessly integrate with image processing. You can find well-established image processing libraries and harness them within your TypeScript projects, saving time and effort.
Now that we understand why TypeScript is a fantastic choice for image processing let’s explore some practical examples and use cases.
2. Getting Started with TypeScript and Image Processing
To begin our journey into TypeScript-powered image processing, we need to set up our development environment and install the necessary dependencies. For this demonstration, we’ll use the popular image processing library, ‘Jimp,’ which offers a wide range of features and is compatible with TypeScript.
2.1. Setting Up Your Development Environment
Before we can dive into image processing, let’s ensure we have Node.js and TypeScript installed on our machine. If you haven’t already, follow these steps to get started:
bash # Install Node.js (if not already installed) https://nodejs.org/ # Install TypeScript globally npm install -g typescript
Once you have Node.js and TypeScript installed, you’re ready to create a new TypeScript project for image processing.
2.2. Initializing Your TypeScript Project
Let’s start by creating a new directory for our project and initializing it with TypeScript.
bash # Create a new directory for your project mkdir image-processing-with-typescript # Navigate to your project directory cd image-processing-with-typescript # Initialize a TypeScript project tsc --init
Now, you have a TypeScript project set up and ready to go.
2.3. Installing the Jimp Library
Next, we’ll install the ‘Jimp’ library, which will be our go-to tool for image processing in TypeScript. Run the following command in your project directory:
bash npm install jimp
With ‘Jimp’ installed, we are all set to start transforming visual data using TypeScript.
3. Basic Image Manipulation with TypeScript
Let’s begin with some fundamental image processing tasks to get a feel for how TypeScript and ‘Jimp’ work together.
3.1. Loading and Displaying an Image
First, create an ‘index.ts’ file in your project directory and import the ‘Jimp’ library:
typescript import Jimp from 'jimp'; // Load an image Jimp.read('input.jpg') .then((image) => { // Display the image image.write('output.jpg'); }) .catch((err) => { console.error(err); });
In this code, we load an image named ‘input.jpg’ using ‘Jimp.read.’ Then, we display the image and save it as ‘output.jpg.’ Run the script using the following command:
bash tsc && node index.js
You will see the ‘input.jpg’ image displayed and saved as ‘output.jpg’ in your project directory.
3.2. Resizing an Image
Resizing images is a common image processing task. Here’s how you can resize an image using TypeScript and ‘Jimp’:
typescript import Jimp from 'jimp'; // Load an image Jimp.read('input.jpg') .then((image) => { // Resize the image to a specific width and height image.resize(300, 200); // Save the resized image image.write('resized.jpg'); }) .catch((err) => { console.error(err); });
In this example, we load an image, resize it to a width of 300 pixels and a height of 200 pixels, and save the resized image as ‘resized.jpg.’
3.3. Applying Filters
‘Jimp’ provides a variety of filters that can be applied to images. Here’s how you can apply a sepia filter to an image:
typescript import Jimp from 'jimp'; // Load an image Jimp.read('input.jpg') .then((image) => { // Apply a sepia filter image.sepia(); // Save the filtered image image.write('sepia.jpg'); }) .catch((err) => { console.error(err); });\
This code loads an image, applies a sepia filter, and saves the filtered image as ‘sepia.jpg.’
4. Advanced Image Processing with TypeScript
Now that we’ve covered the basics, let’s dive into more advanced image processing techniques using TypeScript.
4.1. Image Cropping
Cropping an image allows you to focus on a specific part of it. Here’s how you can crop an image using TypeScript and ‘Jimp’:
typescript import Jimp from 'jimp'; // Load an image Jimp.read('input.jpg') .then((image) => { // Crop the image to the specified coordinates and dimensions image.crop(100, 50, 300, 200); // Save the cropped image image.write('cropped.jpg'); }) .catch((err) => { console.error(err); });
In this example, we load an image and then crop it to a specified set of coordinates and dimensions, saving the cropped image as ‘cropped.jpg.’
4.2. Adding Text to Images
You can enhance images by adding text to them. Here’s how you can add text to an image using TypeScript:
typescript import Jimp from 'jimp'; // Load an image Jimp.read('input.jpg') .then((image) => { // Add text to the image image.print( Jimp.loadFont(Jimp.FONT_SANS_32_BLACK), 10, // x-coordinate 10, // y-coordinate 'Hello, TypeScript!' ); // Save the image with text image.write('image_with_text.jpg'); }) .catch((err) => { console.error(err); });
In this code, we load an image, add text to it, and save the modified image as ‘image_with_text.jpg.’
Conclusion
In this blog post, we’ve explored the exciting fusion of TypeScript and image processing. We’ve seen how TypeScript’s strong typing, scalability, and vast ecosystem make it an excellent choice for working with visual data. By using the ‘Jimp’ library, we’ve covered essential image processing tasks, from resizing and cropping to applying filters and adding text.
As you continue your journey into image processing with TypeScript, you’ll discover even more possibilities, such as face detection, image recognition, and custom image filters. TypeScript’s flexibility and the extensive capabilities of libraries like ‘Jimp’ empower you to tackle complex visual data processing tasks with confidence.
So, whether you’re enhancing website images, automating image manipulation, or diving into computer vision projects, TypeScript and image processing are a dynamic duo that can bring your vision to life. With TypeScript’s safety net and the power of image processing at your fingertips, the possibilities are limitless.
Start experimenting with TypeScript and image processing today, and unlock a world of creative potential at your fingertips. Happy coding!
Table of Contents
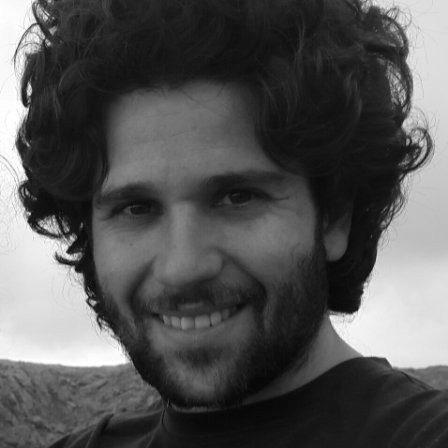
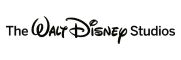