Implementing Data Structures in TypeScript
As developers, understanding data structures is crucial for writing efficient and optimized code. Data structures are fundamental building blocks that organize and store data in memory. They play a vital role in algorithms, ensuring data manipulation, retrieval, and storage are performed as efficiently as possible. In this blog post, we will explore the implementation of essential data structures using TypeScript. By the end, you’ll have a solid foundation in creating and utilizing data structures effectively.
Table of Contents
1. Introduction to Data Structures
1.1. What are Data Structures?
Data structures are containers that organize and store data in a specific way to facilitate efficient access, manipulation, and storage. They provide a set of operations to work with the stored data. Different data structures are designed for various tasks, and the choice of the right data structure is crucial for optimizing algorithms and achieving better performance.
1.2. Importance of Data Structures
Efficient algorithms are built on the foundation of effective data structures. Choosing the appropriate data structure can significantly impact the speed and efficiency of your code. For instance, using the right data structure can lead to faster searching, sorting, and insertion operations. Understanding data structures also enhances problem-solving skills, enabling developers to tackle complex challenges more effectively.
2. Getting Started with TypeScript
2.1. Setting Up a TypeScript Project
Before diving into data structures, make sure you have a TypeScript environment set up. You can create a new TypeScript project using tools like npm or yarn. Once your project is set up, you can start creating TypeScript files and writing code using TypeScript syntax.
2.2. TypeScript Basics
TypeScript is a superset of JavaScript that introduces static typing and advanced features to JavaScript development. With TypeScript, you can define types for variables, functions, and other data structures. This helps catch type-related errors during development and provides better code documentation.
3. Implementing Data Structures
3.1. Arrays
Arrays are one of the simplest and most common data structures. They store elements of the same type in contiguous memory locations, allowing constant-time access to elements using their indices.
typescript const numbers: number[] = [1, 2, 3, 4, 5]; const firstElement: number = numbers[0]; // Access first element numbers.push(6); // Add an element to the end
3.2. Linked Lists
Linked lists consist of nodes, where each node contains data and a reference to the next node in the list. Linked lists are efficient for insertions and deletions, especially in the middle of the list.
typescript class Node<T> { constructor(public data: T, public next: Node<T> | null = null) {} } class LinkedList<T> { constructor(public head: Node<T> | null = null) {} // Insert a new node at the end of the list append(data: T) { const newNode = new Node(data); if (!this.head) { this.head = newNode; return; } let current = this.head; while (current.next) { current = current.next; } current.next = newNode; } }
3.3. Stacks
Stacks follow the Last-In-First-Out (LIFO) principle. Elements are added and removed from the top of the stack. Stacks are useful for tasks like tracking function calls, evaluating expressions, and implementing backtracking algorithms.
typescript class Stack<T> { private items: T[] = []; push(item: T) { this.items.push(item); } pop(): T | undefined { return this.items.pop(); } peek(): T | undefined { return this.items[this.items.length - 1]; } isEmpty(): boolean { return this.items.length === 0; } }
3.4. Queues
Queues follow the First-In-First-Out (FIFO) principle. Elements are added to the back of the queue and removed from the front. Queues are used in scenarios like task scheduling, breadth-first traversal, and more.
typescript class Queue<T> { private items: T[] = []; enqueue(item: T) { this.items.push(item); } dequeue(): T | undefined { return this.items.shift(); } peek(): T | undefined { return this.items[0]; } isEmpty(): boolean { return this.items.length === 0; } }
3.5. Hash Tables (HashMaps)
Hash tables, also known as hash maps, are used to store key-value pairs. They provide fast access to values based on their keys. Hash tables use a hashing function to map keys to specific indices in an array.
typescript class HashMap<K, V> { private data: Record<string, V> = {}; set(key: K, value: V) { const hash = String(key); this.data[hash] = value; } get(key: K): V | undefined { const hash = String(key); return this.data[hash]; } delete(key: K) { const hash = String(key); delete this.data[hash]; } }
3.6. Trees
Trees are hierarchical structures with nodes connected by edges. They have a root node and can have one or more child nodes. Trees are used in various applications, such as representing hierarchical data, organizing file systems, and implementing search algorithms.
typescript class TreeNode<T> { constructor(public data: T, public children: TreeNode<T>[] = []) {} } class Tree<T> { constructor(public root: TreeNode<T>) {} // Tree traversal methods (e.g., in-order, pre-order, post-order) }
3.7. Graphs
Graphs consist of nodes (vertices) connected by edges. They can be used to model complex relationships between entities. Graphs come in various forms, such as directed graphs, undirected graphs, weighted graphs, and more.
typescript class Graph<T> { private vertices: Map<T, T[]> = new Map(); addVertex(vertex: T) { this.vertices.set(vertex, []); } addEdge(vertex1: T, vertex2: T) { this.vertices.get(vertex1)?.push(vertex2); this.vertices.get(vertex2)?.push(vertex1); } getNeighbors(vertex: T): T[] | undefined { return this.vertices.get(vertex); } }
4. Code Examples and Implementation Details
The above code snippets provide a starting point for implementing various data structures in TypeScript. In practice, you can enhance these implementations by adding more methods, optimizing performance, and handling edge cases.
5. Advantages of Using TypeScript
5.1. Type Safety and Static Typing
TypeScript’s static typing helps catch type-related errors during development, leading to more robust and reliable code. It provides better code understanding and documentation by explicitly defining the types of variables and functions.
5.2. Enhanced Code Maintainability
With TypeScript, your codebase becomes more maintainable and readable. The enforced types make it easier to understand the purpose and usage of different variables and functions, reducing ambiguity and potential bugs.
5.3. Improved Developer Productivity
TypeScript’s features, such as auto-completion and code navigation, boost developer productivity. The early detection of type-related errors prevents runtime surprises and allows developers to focus on writing high-quality code.
6. Best Practices for Data Structure Implementation
6.1. Choosing the Right Data Structure
Selecting the appropriate data structure depends on the problem’s requirements and constraints. Analyze the operations you need to perform frequently and choose a data structure that optimizes those operations.
6.2. Time and Space Complexity Considerations
When implementing data structures, consider the time and space complexity of various operations. A data structure may excel in one operation but might be inefficient in another. Strive for a balance between different complexity factors.
6.3. Balancing Performance and Readability
While optimizing for performance is important, maintain code readability as well. Comment your code, follow consistent naming conventions, and use meaningful variable names. A clear and understandable implementation is valuable for collaboration and future maintenance.
7. Real-world Applications
Different data structures find applications in various scenarios. For instance:
- Arrays are used for quick access to elements by index.
- Linked lists are efficient for dynamic memory allocation and frequent insertions/deletions.
- Stacks and queues play a role in function call management, parsing expressions, and more.
- Hash maps are crucial for fast data retrieval based on keys.
- Trees and graphs are used in search algorithms, hierarchical data representation, and network modeling.
Conclusion
Understanding and implementing data structures in TypeScript empowers developers to write efficient and optimized code. Each data structure has its strengths and weaknesses, making them suitable for specific tasks. By mastering these fundamental building blocks, you’ll be better equipped to design elegant solutions and solve complex problems in your coding journey. Remember to practice regularly and explore real-world applications to deepen your understanding of data structures and algorithms. Happy coding!
Table of Contents
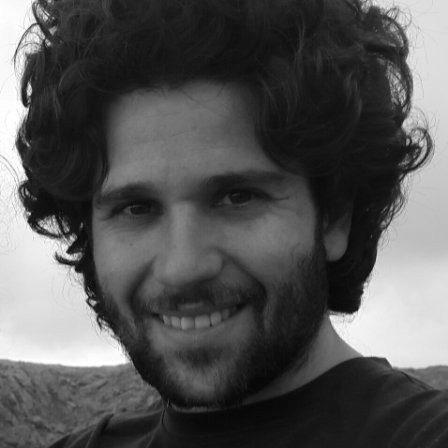
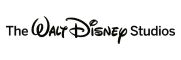