Integrating Express.js with TypeScript
In the world of web development, the robustness of your codebase and the efficiency of your workflow are critical factors. As projects grow in complexity, maintaining code quality and preventing bugs becomes increasingly challenging. This is where TypeScript and Express.js come into play. In this blog post, we will explore the benefits of integrating Express.js with TypeScript and provide a comprehensive guide to help you get started.
Table of Contents
1. Why Choose TypeScript and Express.js?
1.1. TypeScript: Enhancing Type Safety and Development Experience
TypeScript is a superset of JavaScript that introduces static typing to the language. This means you can catch type-related errors at compile time, leading to more robust and reliable code. When building web applications, TypeScript’s type checking helps prevent common runtime errors, improves code readability, and enhances collaboration among developers.
2. Express.js: A Powerful Node.js Framework
Express.js, often referred to as Express, is a fast and minimalist web application framework for Node.js. It provides a range of features for building web and mobile applications, APIs, and more. Express simplifies common tasks like routing, middleware management, and handling HTTP requests, allowing developers to focus on creating efficient and scalable applications.
3. Benefits of Integrating Express.js with TypeScript
Integrating Express.js with TypeScript offers several advantages that contribute to a smoother development process and more reliable applications:
3.1. Type Safety
TypeScript’s static typing helps catch errors during development, reducing the chances of encountering unexpected issues in production. With type definitions for Express.js, you can ensure that your route handlers, middleware functions, and request/response objects are used correctly.
typescript import express, { Request, Response } from 'express'; const app = express(); app.get('/hello', (req: Request, res: Response) => { const name: string = req.query.name; res.send(`Hello, ${name}!`); });
3.2. Code Readability and Maintainability
TypeScript’s type annotations make your code more self-documenting. By explicitly specifying types, you provide clear information about the structure of data and function parameters. This enhances collaboration among team members and simplifies maintenance as the project scales.
3.3. Enhanced IDE Support
TypeScript’s type information enables powerful code completion, navigation, and refactoring tools in popular integrated development environments (IDEs) like Visual Studio Code. This results in a more efficient and enjoyable coding experience.
3.4. Early Error Detection
TypeScript’s static analysis identifies potential errors before runtime, allowing you to catch and fix issues early in the development process. This saves time and effort compared to debugging runtime errors in a pure JavaScript project.
3.5. Improved Documentation
TypeScript encourages developers to write detailed type annotations and JSDoc comments. This practice leads to more comprehensive and accurate documentation, making it easier for both developers and other stakeholders to understand the codebase.
4. Getting Started: Integrating Express.js with TypeScript
Now that we’ve covered the benefits, let’s dive into the practical steps of integrating Express.js with TypeScript in your Node.js project.
4.1. Setting Up Your Project
Start by initializing a new Node.js project if you haven’t already:
bash mkdir my-express-app cd my-express-app npm init -y
4.2. Installing Dependencies
Install TypeScript and Express.js packages as project dependencies:
bash npm install typescript @types/express express
The @types/express package provides TypeScript type definitions for Express.js.
4.3. Creating a Basic Server
Create a src directory in your project’s root and add a TypeScript file, e.g., app.ts.
typescript // src/app.ts import express, { Request, Response } from 'express'; const app = express(); const PORT = 3000; app.get('/', (req: Request, res: Response) => { res.send('Hello, TypeScript with Express!'); }); app.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
4.4. Configuring TypeScript
Create a tsconfig.json file in the root directory of your project to configure TypeScript.
json { "compilerOptions": { "target": "ESNext", "module": "CommonJS", "outDir": "./dist", "rootDir": "./src", "strict": true, "esModuleInterop": true } }
Here, we’re configuring TypeScript to transpile our code from the src directory to the dist directory.
4.5. Running the Application
Add scripts to your package.json to build and start the application:
json { "scripts": { "build": "tsc", "start": "node dist/app.js" } }
Now you can build and run your application:
bash npm run build npm start
5. Advanced Tips and Techniques
5.1. Using Middleware with Type Annotations
When working with middleware in Express.js, you can ensure type safety by defining custom interfaces for your middleware functions. For instance:
typescript import express, { Request, Response, NextFunction } from 'express'; interface CustomRequest extends Request { user: { id: number; username: string; }; } const app = express(); function authenticate(req: CustomRequest, res: Response, next: NextFunction) { // Check authentication logic req.user = { id: 1, username: 'john_doe' }; next(); } app.use(authenticate); app.get('/profile', (req: CustomRequest, res: Response) => { res.send(`Welcome, ${req.user.username}!`); });
5.2. Using Third-Party Middleware
When using third-party middleware that doesn’t have type definitions available, you can create declaration files (.d.ts) to provide type information. This ensures your project maintains type safety even with external libraries.
Conclusion
Integrating Express.js with TypeScript brings together the power of a robust web framework and the benefits of static typing. This combination enhances your development experience, improves code quality, and reduces the likelihood of runtime errors. By following the steps outlined in this guide, you can seamlessly set up a project that harnesses the strengths of both technologies. Whether you’re starting a new project or migrating an existing one, adopting TypeScript with Express.js is a strategic choice that will pay off in the long run. So go ahead and dive into the world of expressive, type-safe Node.js web applications!
Table of Contents
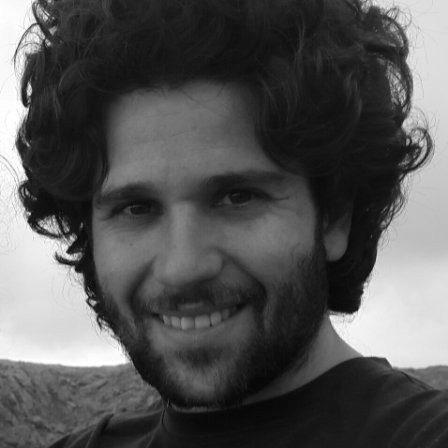
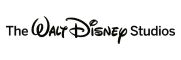