TypeScript and Internationalization: Building Multilingual Apps
In our increasingly interconnected world, creating applications that cater to a global audience is essential. Multilingual apps are not just a feature; they are a necessity. TypeScript, a statically typed superset of JavaScript, provides a powerful foundation for building robust and maintainable multilingual applications. In this comprehensive guide, we’ll explore how to harness the capabilities of TypeScript to internationalize your web or mobile applications effectively.
Table of Contents
1. Understanding Internationalization (i18n)
1.1 What is Internationalization?
Internationalization, often abbreviated as i18n, is the process of designing and developing software applications in a way that allows them to be easily adapted to different languages, regions, and cultures. This involves not only translating text but also adapting date formats, number formats, currency symbols, and other elements to suit the preferences of the target audience.
1.2 Why Internationalization Matters
Internationalization is crucial for several reasons:
- Global Reach: It enables your app to reach a wider audience, potentially increasing your user base and revenue.
- User Experience: Users are more likely to engage with an app that speaks their language and respects their cultural norms.
- Compliance: In some regions, there are legal requirements to provide software in the local language.
- Competitive Advantage: Offering a multilingual app can give you an edge over competitors who don’t.
2. TypeScript: A Solid Foundation
TypeScript, developed by Microsoft, is a statically typed superset of JavaScript. It provides the benefits of static typing, which helps catch errors early in the development process, while still maintaining compatibility with JavaScript. TypeScript is an excellent choice for building multilingual apps because it offers strong support for type checking and code maintainability.
To get started, ensure you have TypeScript installed globally:
bash npm install -g typescript
3. Setting Up Your Multilingual TypeScript Project
Building a multilingual app with TypeScript requires careful project setup. Let’s walk through the essential steps.
3.1 Initializing a TypeScript Project
Start by creating a new TypeScript project or converting an existing JavaScript project to TypeScript using:
bash tsc --init
This command generates a tsconfig.json file, where you can configure TypeScript settings for your project.
3.2 Adding Internationalization Libraries
For internationalization support, you’ll need external libraries like i18next or react-intl for React applications. Install these libraries using npm or yarn:
bash npm install i18next # or yarn add i18next
3.3 Project Structure
Organize your project structure to accommodate internationalization. Create a separate folder for language files, translation keys, and components that need translation. A typical structure might look like this:
plaintext src/ |-- assets/ | |-- locales/ | | |-- en.json | | |-- es.json |-- components/ | |-- Header.tsx | |-- ... |-- App.tsx
4. Translating Your App
4.1 Extracting Text for Translation
To make your app multilingual, you need to identify and extract all translatable text. Replace hard-coded strings with keys that correspond to translation files. For example:
tsx // Before <h1>Hello, World!</h1> // After <h1>{t('welcome.heading')}</h1>
4.2 Using Localization Libraries
Utilize localization libraries like i18next to load and apply translations to your app. Configure the library with language files and initialize it in your app’s entry point. Here’s a simplified example:
tsx // Initialize i18next import i18next from 'i18next'; import { initReactI18next } from 'react-i18next'; i18next .use(initReactI18next) // For React applications .init({ resources: { en: { translation: { 'welcome.heading': 'Hello, World!', }, }, es: { translation: { 'welcome.heading': '¡Hola, Mundo!', }, }, }, lng: 'en', // Default language fallbackLng: 'en', // Fallback language interpolation: { escapeValue: false, }, });
5. Language Detection and User Preferences
5.1 Detecting User’s Language
To enhance the user experience, automatically detect the user’s preferred language. You can use the navigator.language property or other methods to determine the user’s locale.
tsx const userLanguage = navigator.language.split('-')[0]; // Extract the language code
5.2 Allowing Language Preferences
Give users the option to change the app’s language preferences. Provide a language selector that allows them to switch between available languages. Update the i18next configuration when the user selects a different language.
6. Implementing Internationalization in React and Angular Apps
Internationalization in React and Angular apps follows similar principles but may have some framework-specific implementations.
6.1 React Apps
In React, you can use the useTranslation hook from react-i18next to access translations in your components. Here’s an example:
tsx import { useTranslation } from 'react-i18next'; function Welcome() { const { t } = useTranslation(); return <h1>{t('welcome.heading')}</h1>; }
6.2 Angular Apps
In Angular, you can use the ngx-translate/core library for internationalization. Configure the library and use the translate pipe in your templates.
7. Testing and Debugging
Thoroughly test your multilingual app with different languages and locales to ensure translations are accurate and UI elements are properly adjusted. Use tools like the developer console to debug any localization issues that may arise.
8. Performance Considerations
When building multilingual apps, consider performance implications, especially if your app supports a large number of languages. Optimize asset loading and minimize the impact of translations on load times.
9. Deploying and Scaling Your Multilingual App
Deploy your multilingual app to a hosting platform that supports global content delivery. Consider scaling strategies to handle increased traffic from a diverse user base. Monitor user feedback and make improvements as necessary.
Conclusion
Creating multilingual apps using TypeScript is not just about translating text; it’s about providing a seamless experience for users from different language backgrounds. TypeScript’s type safety and maintainability, combined with internationalization libraries, make it a powerful choice for building applications that can thrive in a global market.
By following the steps outlined in this guide, you’ll be well-equipped to embark on your multilingual app development journey. Embrace diversity, reach a broader audience, and make your application truly global with TypeScript and internationalization.
Table of Contents
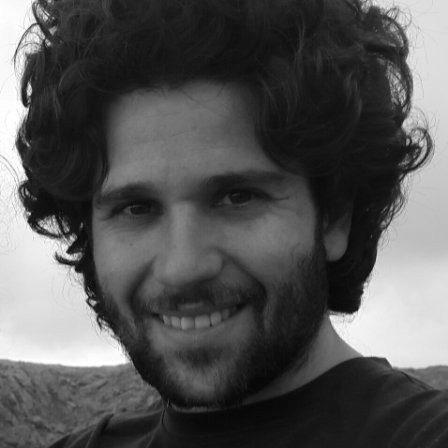
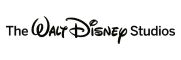