TypeScript and IoT: Developing Connected Devices
The Internet of Things (IoT) has revolutionized the way we interact with the world around us. From smart thermostats that regulate our home temperature to wearable devices that monitor our health, IoT has permeated every aspect of our lives. Behind these connected devices lies a complex ecosystem of hardware and software. In this blog, we will explore how TypeScript, a statically typed superset of JavaScript, can be a game-changer when it comes to developing IoT applications. We’ll delve into the advantages it offers, provide practical insights, and even showcase code samples to help you get started on your IoT journey.
Table of Contents
1. TypeScript: A Brief Overview
Before diving into the intricacies of IoT development with TypeScript, let’s take a moment to understand what TypeScript is and why it’s gaining traction in the world of IoT.
1.1. What Is TypeScript?
TypeScript is an open-source programming language developed by Microsoft. It is designed to build robust and scalable web applications. TypeScript is often referred to as a “superset” of JavaScript because it extends JavaScript by adding optional static typing to the language. This means you can write JavaScript code in TypeScript, but you can also take advantage of static type checking to catch errors at compile-time rather than runtime.
1.2. Why TypeScript for IoT?
IoT development involves working with a wide range of hardware devices, sensors, and communication protocols. The complexity of IoT projects demands a programming language that offers strong typing, code quality improvements, and tooling support. TypeScript excels in these areas and brings several key advantages to IoT development:
1.2.1. Strong Typing
In IoT development, data integrity and reliability are paramount. TypeScript’s strong typing ensures that data types are strictly adhered to, reducing the likelihood of runtime errors. This is particularly crucial when handling sensor data, as accuracy is vital in many IoT applications.
1.2.2. Improved Code Quality
The static type checking provided by TypeScript helps developers write cleaner and more maintainable code. This leads to fewer bugs, easier debugging, and a more robust IoT application overall.
1.2.3. Enhanced Device Connectivity
IoT devices often communicate with various protocols, such as MQTT, CoAP, or HTTP. TypeScript’s flexibility allows developers to create well-structured code for handling these communication protocols, making it easier to connect and interact with IoT devices.
2. Setting Up Your Development Environment
Now that we understand why TypeScript is a great choice for IoT development, let’s set up our development environment so we can start building connected devices.
2.1. Prerequisites
Before we begin, make sure you have the following prerequisites in place:
- Node.js: Ensure you have Node.js installed on your system. You can download it from the official Node.js website (https://nodejs.org/).
- Visual Studio Code: While you can use any code editor or IDE of your choice, Visual Studio Code (VS Code) is highly recommended for TypeScript development. You can download it from (https://code.visualstudio.com/).
3. Creating a TypeScript Project
Follow these steps to create a new TypeScript project for your IoT development:
- Initialize a New Node.js Project: Open your terminal and navigate to the directory where you want to create your project. Run the following command to initialize a new Node.js project:
bash npm init -y
This command creates a package.json file with default settings.
- Install TypeScript: Install TypeScript as a development dependency by running the following command:
bash npm install typescript --save-dev
- Create a TypeScript Configuration File: Generate a TypeScript configuration file using the following command:
bash npx tsc --init
This command creates a tsconfig.json file with default settings. You can customize this file to suit your project’s needs.
- Setting Up Your Project Structure: Organize your project structure by creating directories for your source code, tests, and any other assets you may need. Here’s a basic project structure to get you started:
css my-iot-project/ ??? src/ ? ??? main.ts ??? tests/ ??? tsconfig.json ??? package.json
Your TypeScript source code will go in the src directory, and you can add tests in the tests directory.
4. Writing TypeScript Code for IoT
With your development environment set up, let’s dive into writing TypeScript code for IoT. In this section, we’ll cover a simple example of reading data from a temperature sensor and sending it to a cloud service using TypeScript.
4.1. Example: Reading Temperature Data
Imagine you have a temperature sensor connected to a Raspberry Pi, and you want to read temperature data from the sensor and send it to a cloud platform. Here’s how you can accomplish this using TypeScript:
- Install Dependencies: Install the required npm packages for working with GPIO pins and making HTTP requests:
bash npm install rpio axios --save
Write TypeScript Code:
typescript // Import necessary modules import * as rpio from 'rpio'; import axios from 'axios'; // Define GPIO pin for the temperature sensor const sensorPin = 17; // Initialize GPIO rpio.init({ gpiomem: false }); rpio.open(sensorPin, rpio.INPUT); // Function to read temperature from the sensor function readTemperature() { const rawData = rpio.read(sensorPin); // Perform temperature conversion here (specific to your sensor) const temperature = convertRawDataToTemperature(rawData); return temperature; } // Function to send temperature data to the cloud async function sendTemperatureToCloud(temperature: number) { try { const response = await axios.post('https://api.your-cloud-service.com/temperature', { temperature: temperature, }); console.log('Temperature data sent successfully:', response.data); } catch (error) { console.error('Error sending temperature data:', error); } } // Main loop setInterval(() => { const temperature = readTemperature(); sendTemperatureToCloud(temperature); }, 10000); // Read and send data every 10 seconds
In this code sample, we use the rpio library to interact with GPIO pins on the Raspberry Pi, read temperature data from a sensor, and send it to a cloud service using the axios library.
5. TypeScript and IoT: The Future
As IoT continues to grow and evolve, the role of TypeScript in IoT development becomes even more significant. TypeScript’s strong typing, code quality improvements, and extensive ecosystem of libraries and tools make it a powerful choice for building connected devices.
Whether you’re working on a small hobby project or a large-scale industrial IoT solution, TypeScript can streamline your development process and help you create more reliable and efficient IoT applications. As you explore the world of IoT, consider TypeScript as your trusted companion in building the next generation of connected devices.
Conclusion
In this blog, we’ve explored how TypeScript can enhance IoT development by providing strong typing, improved code quality, and enhanced device connectivity. We’ve also walked through the process of setting up a TypeScript development environment for IoT and provided a practical example of reading temperature data from a sensor and sending it to a cloud service.
As you embark on your IoT journey, remember that TypeScript is just one piece of the puzzle. Successful IoT development also requires a solid understanding of hardware, communication protocols, and cloud services. With TypeScript in your toolkit, you’re well-equipped to tackle the challenges and opportunities of the IoT world.
So, roll up your sleeves, grab your hardware, and start building the next generation of connected devices with TypeScript by your side. Happy coding!
Table of Contents
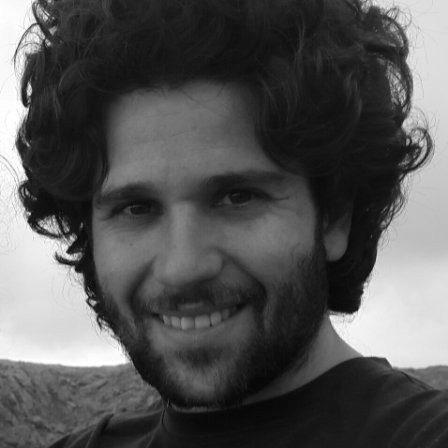
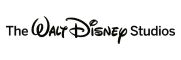