TypeScript and Kubernetes: Container Orchestration Made Easy
In the ever-evolving world of software development, containerization and orchestration have become essential components for building scalable and resilient applications. Kubernetes has emerged as the de facto standard for container orchestration, while TypeScript has gained popularity as a statically typed superset of JavaScript, providing type safety and improved code quality. Combining TypeScript with Kubernetes can make container orchestration a breeze. In this blog, we’ll explore how TypeScript can simplify Kubernetes development, from writing custom controllers to handling configurations and deploying applications.
Table of Contents
1. TypeScript: A Brief Introduction
Before diving into the world of Kubernetes, let’s briefly introduce TypeScript and understand why it’s a valuable tool for developers.
1.1. What is TypeScript?
TypeScript is a statically typed, open-source superset of JavaScript developed and maintained by Microsoft. It brings optional static typing to JavaScript, enabling developers to catch type-related errors at compile time rather than runtime. This leads to more reliable and maintainable code, especially in large codebases.
Key features of TypeScript include:
- Static Typing: TypeScript allows developers to specify the types of variables, function parameters, and return values, which helps identify and prevent type-related bugs.
- IDE Support: Popular integrated development environments (IDEs) like Visual Studio Code offer excellent TypeScript support, including autocompletion, error checking, and documentation.
- Tooling: TypeScript comes with a powerful type system and a compiler that generates clean and efficient JavaScript code, making it suitable for both frontend and backend development.
Now that we have a basic understanding of TypeScript, let’s explore how it can enhance Kubernetes development.
2. TypeScript and Kubernetes: A Powerful Combo
Kubernetes is a powerful container orchestration platform that automates the deployment, scaling, and management of containerized applications. While Kubernetes offers a rich set of features and abstractions, developing for Kubernetes can be challenging due to its complex ecosystem and the need to manage resources, configurations, and custom controllers.
This is where TypeScript shines as a development language for Kubernetes. TypeScript’s strong typing, tooling, and robust ecosystem can significantly simplify the development process. Let’s delve into specific areas where TypeScript makes Kubernetes development easier.
2.1. Custom Controllers with TypeScript
Custom controllers are an integral part of Kubernetes, allowing you to extend the platform’s functionality and automate tasks specific to your application. Writing custom controllers in TypeScript offers several advantages:
- Type Safety: TypeScript’s static typing helps catch errors early in the development process, reducing the chances of runtime issues in your custom controllers.
typescript import { CustomResource } from 'k8s'; import { MyCustomResource } from './types'; function reconcileResource(resource: CustomResource<MyCustomResource>) { // Type-safe operations on MyCustomResource }
- IDE Support: TypeScript provides excellent code completion and navigation in modern IDEs. This makes it easier to explore Kubernetes APIs and understand the structure of custom resources.
typescript const myCustomResource = new MyCustomResource(); myCustomResource.metadata.name = 'my-resource'; // TypeScript suggests available properties and methods
- Better Refactoring: Renaming or refactoring resources becomes more manageable with TypeScript, as you can rely on IDE tools to update references throughout your codebase.
2.2. Enhanced Configurations
Kubernetes applications often rely on configuration files for various purposes, such as defining deployment settings, specifying environment variables, or creating secrets. TypeScript can improve how you work with these configurations.
Type-Checked Configurations: By defining TypeScript interfaces that match your configuration files, you can ensure that the configurations are correct at compile time.
typescript interface AppConfig { apiUrl: string; apiKey: string; } const config: AppConfig = { apiUrl: 'https://api.example.com', apiKey: 'my-secret-key', };
Configuration Validation: You can leverage TypeScript’s type checking to validate configuration files before deploying them to Kubernetes, reducing the risk of misconfigurations causing runtime issues.
typescript function validateConfig(config: AppConfig): void { if (!config.apiUrl || !config.apiKey) { throw new Error('Incomplete configuration'); } }
2.3. Kubernetes Resource Definitions as Code
In Kubernetes, you define resources like pods, services, and deployments using YAML or JSON manifests. While these declarative files are powerful, they lack the benefits of a programming language. TypeScript can bring the advantages of code to your resource definitions.
- Programmatic Resource Generation: With TypeScript, you can generate Kubernetes resource definitions programmatically, allowing for dynamic configurations and easier templating.
typescript import { Pod } from 'k8s'; function createPod(name: string, image: string): Pod { return new Pod({ metadata: { name }, spec: { containers: [{ name: 'container', image }] }, }); } const myPod = createPod('my-pod', 'nginx:latest');
- Reusability: TypeScript enables you to encapsulate common resource patterns into reusable functions or libraries, reducing duplication and improving maintainability.
2.4. Strongly Typed Kubernetes APIs
Kubernetes exposes a vast API surface, and navigating this landscape can be challenging. TypeScript helps you interact with Kubernetes APIs in a more reliable and intuitive manner.
- Type-Checked API Requests: When making API requests to Kubernetes, TypeScript ensures that you provide the correct resource types and request parameters.
typescript import { CoreV1Api, V1Pod } from 'k8s'; const api = new CoreV1Api(); const podList = await api.listPodForAllNamespaces(); // TypeScript knows that podList.items is an array of V1Pod
- Intuitive Object Structures: TypeScript interfaces mirror the structure of Kubernetes objects, making it easier to understand and work with Kubernetes resources.
typescript import { V1Pod } from 'k8s'; function getPodName(pod: V1Pod): string { return pod.metadata?.name || 'Unknown'; }
3. Building and Deploying TypeScript Kubernetes Applications
Now that we’ve explored how TypeScript can simplify Kubernetes development, let’s look at the process of building and deploying TypeScript-based applications on a Kubernetes cluster.
3.1. Building TypeScript Applications
To build TypeScript applications for Kubernetes, you’ll need to compile your TypeScript code into JavaScript. Here’s how you can do it:
Step 1: Install TypeScript globally (if you haven’t already) using npm:
bash npm install -g typescript
Step 2: Navigate to your TypeScript project directory and create a tsconfig.json file:
bash tsc --init
Step 3: Configure your tsconfig.json to match your project requirements. You can specify the output directory and other compilation options.
Step 4: Compile your TypeScript code:
bash tsc
This will generate JavaScript files from your TypeScript code, ready to be bundled into containers.
3.2. Dockerizing TypeScript Applications
To deploy your TypeScript application on Kubernetes, you’ll need to package it into a Docker container. Here’s a simplified example of a Dockerfile for a TypeScript-based Node.js application:
Dockerfile # Use an official Node.js runtime as a parent image FROM node:14 # Set the working directory in the container WORKDIR /app # Copy package.json and package-lock.json to the working directory COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application code to the working directory COPY . . # Build the TypeScript code RUN tsc # Expose a port (if your application listens on a specific port) EXPOSE 3000 # Define the command to run your application CMD [ "node", "dist/main.js" ]
3.3. Deploying to Kubernetes
Once you have your Docker image ready, you can deploy it to Kubernetes using a Kubernetes manifest file. Here’s a minimal example of a Kubernetes Deployment:
yaml apiVersion: apps/v1 kind: Deployment metadata: name: my-app spec: replicas: 3 selector: matchLabels: app: my-app template: metadata: labels: app: my-app spec: containers: - name: my-app-container image: your-docker-registry/my-app:latest
Apply this manifest using kubectl apply -f deployment.yaml, and Kubernetes will create and manage the specified number of pods running your TypeScript application.
4. TypeScript and Kubernetes in Action
Let’s put our TypeScript and Kubernetes knowledge to work by building a simple application that demonstrates how they can work together effectively. In this example, we’ll create a TypeScript-based Kubernetes controller that watches for changes to a custom resource and deploys a corresponding pod.
Step 1: Set Up Your Development Environment
Before we begin, ensure you have the following prerequisites installed:
- Node.js and npm
- TypeScript
- Docker
- Kubernetes cluster (you can use Minikube or any other Kubernetes setup)
Step 2: Create a TypeScript Project
Create a new directory for your project and initialize it:
bash mkdir k8s-ts-controller cd k8s-ts-controller npm init -y tsc --init
Step 3: Install Dependencies
Install the necessary dependencies:
bash npm install k8s @kubernetes/client-node
Step 4: Define the Custom Resource
Create a TypeScript file (e.g., MyCustomResource.ts) to define the custom resource:
typescript import { CustomResource } from 'k8s'; export class MyCustomResource extends CustomResource { apiVersion: 'example.com/v1'; kind: 'MyCustomResource'; metadata: { name: string; }; spec: { // Define your custom resource properties here }; }
Step 5: Create the Kubernetes Controller
Create another TypeScript file (e.g., controller.ts) for the Kubernetes controller:
typescript import { KubeConfig, CustomResource } from 'k8s'; import { MyCustomResource } from './MyCustomResource'; const kubeconfig = new KubeConfig(); kubeconfig.loadFromDefault(); const crdClient = kubeconfig.makeApiClient(CustomResource); async function main() { const watch = await crdClient.list('example.com', 'v1', 'mycustomresources'); for await (const event of watch) { const resource = event.object as MyCustomResource; // Handle changes to MyCustomResource here console.log(`Received event for MyCustomResource: ${resource.metadata.name}`); } } main().catch((err) => { console.error(err); });
Step 6: Build and Deploy the Controller
Build your TypeScript code:
bash tsc
Dockerize your controller:
bash docker build -t my-controller .
Create a Kubernetes Deployment manifest (e.g., controller-deployment.yaml) to deploy your controller:
yaml apiVersion: apps/v1 kind: Deployment metadata: name: my-controller spec: replicas: 1 selector: matchLabels: app: my-controller template: metadata: labels: app: my-controller spec: containers: - name: my-controller image: my-controller:latest
Apply the deployment to your Kubernetes cluster:
bash kubectl apply -f controller-deployment.yaml
Step 7: Create a Custom Resource
Create a custom resource YAML file (e.g., mycustomresource.yaml) to test your controller:
yaml apiVersion: example.com/v1 kind: MyCustomResource metadata: name: my-resource spec: // Define your custom resource properties here
Apply the custom resource to your cluster:
bash kubectl apply -f mycustomresource.yaml
Step 8: Observe the Controller’s Actions
Watch the logs of your controller to observe its actions:
bash kubectl logs -f deployment/my-controller
You should see log messages indicating that your controller is reacting to changes in the custom resource.
Congratulations! You’ve successfully built a Kubernetes controller using TypeScript.
Conclusion
TypeScript and Kubernetes make a powerful combination for container orchestration and management. TypeScript’s static typing, IDE support, and tooling enhance Kubernetes development, making it more reliable and efficient. By leveraging TypeScript, you can simplify the creation of custom controllers, handle configurations with confidence, and write Kubernetes resource definitions as code.
With TypeScript, you can streamline your Kubernetes development workflow, catch errors early, and create more maintainable applications. Whether you’re developing custom controllers, building microservices, or managing complex Kubernetes deployments, TypeScript is a valuable tool in your toolkit.
Start exploring TypeScript and Kubernetes today, and unlock the potential of container orchestration made easy. Happy coding!
Table of Contents
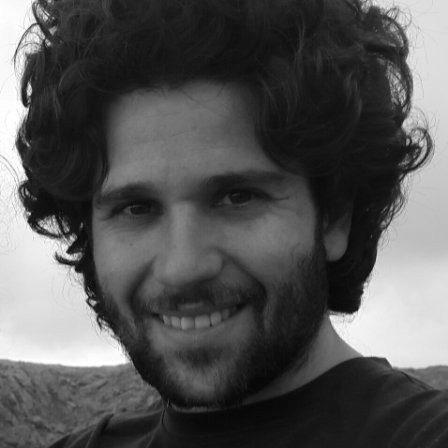
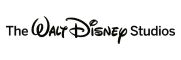