TypeScript Linters and Code Quality Tools
In the fast-paced world of software development, maintaining code quality and catching errors early are paramount. Enter TypeScript linters and code quality tools – your indispensable companions in the quest for cleaner, more reliable code. In this blog, we’ll dive into the world of TypeScript linters, explore their benefits, and showcase some popular tools that can help elevate your development workflow to new heights.
Table of Contents
1. Why TypeScript Linters Matter
Before delving into the specifics, let’s understand why TypeScript linters are crucial for your projects. Linters are automated tools that analyze your codebase for potential issues, inconsistencies, and violations of coding standards. In the TypeScript ecosystem, these tools perform static code analysis to identify problems without executing the code. Here’s why they matter:
1.1. Early Error Detection
Linters excel at catching errors early in the development process. They analyze your code as you write it, highlighting issues such as syntax errors, type mismatches, and undefined variables before you even run your application. This early detection saves time and effort, reducing the likelihood of bugs reaching production.
1.2. Consistent Coding Standards
Maintaining consistent coding standards across a team or project is challenging. Linters enforce these standards by flagging deviations from predefined rules. This leads to cleaner, more readable code that’s easier to maintain, understand, and collaborate on.
1.3. Enhanced Code Readability
Readable code is essential for long-term maintainability. Linters suggest improvements in naming conventions, formatting, and organization, resulting in code that is not only functional but also easily comprehensible.
1.4. Improved Codebase Robustness
By identifying potential issues and enforcing best practices, linters contribute to a more robust codebase. This means fewer bugs, easier troubleshooting, and a smoother development process overall.
2. Exploring TypeScript Linters
Let’s explore some popular TypeScript linters and code quality tools that can significantly enhance your development experience.
2.1. ESLint
ESLint is a widely used linter that supports TypeScript among other languages. It’s highly configurable and extensible, allowing you to tailor linting rules to your project’s specific needs. With its vast array of plugins and community-driven configurations, you can enforce a wide range of coding standards, from style consistency to best practices.
Setting up ESLint for TypeScript is straightforward:
Install ESLint and TypeScript as development dependencies:
bash npm install eslint typescript --save-dev
Create an ESLint configuration file (.eslintrc.js or .eslintrc.json) in your project’s root directory.
Configure ESLint to use TypeScript parser and recommended TypeScript rules.
javascript module.exports = { parser: '@typescript-eslint/parser', plugins: ['@typescript-eslint'], extends: ['plugin:@typescript-eslint/recommended'], };
2.2. TSLint
While TSLint has been deprecated in favor of using ESLint with TypeScript, it’s worth mentioning due to its historical significance. If your project still uses TSLint, consider transitioning to ESLint for better community support and ongoing development.
2.3. Prettier
While not strictly a linter, Prettier plays a complementary role in code formatting. It ensures consistent code formatting across your entire project, eliminating debates over style preferences. When used in tandem with ESLint, Prettier can be integrated to format code according to ESLint rules.
Setting up Prettier is simple:
Install Prettier as a development dependency:
bash npm install prettier --save-dev
Create a Prettier configuration file (.prettierrc.js or .prettierrc.json) in your project’s root directory.
Configure formatting rules as per your project’s preferences:
javascript module.exports = { semi: true, singleQuote: true, trailingComma: 'all', };
2.4. TypeScript-ESLint
TypeScript-ESLint combines the power of TypeScript with the flexibility and extensibility of ESLint. It’s an officially endorsed solution by TypeScript and ESLint teams, offering a seamless integration of TypeScript-specific linting rules and analysis.
Setting up TypeScript-ESLint involves a few additional steps compared to ESLint:
Install TypeScript-ESLint and required plugins as development dependencies:
bash npm install @typescript-eslint/{parser,eslint-plugin} --save-dev
Configure ESLint to use TypeScript-ESLint parser and recommended TypeScript-ESLint rules in your ESLint configuration file.
javascript module.exports = { parser: '@typescript-eslint/parser', plugins: ['@typescript-eslint'], extends: ['plugin:@typescript-eslint/recommended'], };
3. Integrating Linters into Your Workflow
Having explored some powerful TypeScript linters, let’s discuss how to integrate them seamlessly into your development workflow.
3.1. Automated Code Checks
Integrate linters into your continuous integration (CI) pipeline to automatically check code quality on every code push. This ensures that all contributors adhere to coding standards, preventing regressions and maintaining a high-quality codebase.
3.2. Editor Integration
Use linters with your code editor or integrated development environment (IDE) for real-time feedback as you write code. Many popular editors, such as Visual Studio Code, offer extensions that integrate linters directly into your workflow, highlighting issues and suggesting improvements as you type.
3.3. Custom Rules
Tailor linting rules to your project’s unique requirements. While adopting recommended rules is a good starting point, you can create custom rules that align with your team’s coding style and specific needs.
Conclusion
TypeScript linters and code quality tools are indispensable companions on your journey to writing cleaner, more reliable code. With their ability to catch errors early, enforce coding standards, and improve the overall quality of your codebase, these tools play a vital role in enhancing your development workflow. Whether you opt for the flexibility of ESLint, the TypeScript-specific features of TypeScript-ESLint, or the code formatting prowess of Prettier, integrating these tools into your workflow can elevate your development experience to new heights. So, why wait? Start integrating TypeScript linters and code quality tools into your projects today, and watch your codebase flourish.
Table of Contents
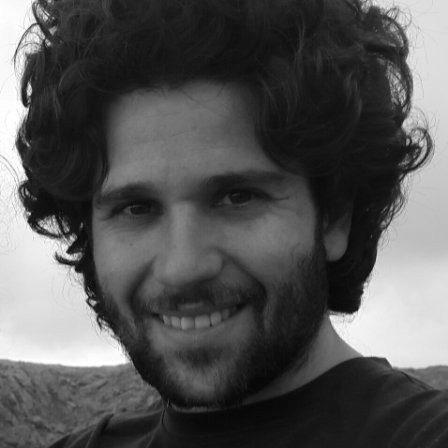
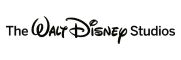