TypeScript and Microservices: Building Scalable Architectures
In the ever-evolving landscape of software development, microservices have emerged as a powerful architectural paradigm. Microservices enable the decomposition of complex applications into smaller, independently deployable services. This architecture promotes scalability, maintainability, and rapid development. To harness the full potential of microservices, developers often turn to TypeScript—a statically typed superset of JavaScript. In this blog, we’ll explore how TypeScript plays a pivotal role in building scalable microservices architectures, complete with code samples and best practices.
Table of Contents
1. The Microservices Revolution
Microservices architecture has revolutionized the way we design, develop, and deploy software applications. Unlike traditional monolithic architectures, where an application is a single, tightly-coupled unit, microservices break down the application into smaller, loosely-coupled services. Each microservice is responsible for a specific business capability and communicates with others via well-defined APIs.
1.1. Advantages of Microservices
- Scalability: Microservices can be independently scaled, allowing you to allocate resources where they are needed the most. This elasticity is invaluable for handling varying workloads.
- Maintainability: Smaller codebases are easier to manage, test, and maintain. Teams can work on individual microservices without affecting the entire application.
- Rapid Development: Microservices foster agility by enabling teams to develop and release new features faster. You can also use different programming languages and technologies for each service.
- Fault Isolation: If one microservice fails, it doesn’t bring down the entire application. This ensures fault tolerance and high availability.
- Technology Diversity: You can choose the most suitable technology stack for each microservice, optimizing performance and scalability.
While microservices offer these compelling benefits, the key to a successful implementation lies in choosing the right tools and technologies to build and manage them. TypeScript is one such tool that can significantly enhance your microservices architecture.
2. TypeScript: A Strong Typing Companion
TypeScript, often dubbed as “JavaScript that scales,” brings static typing to the dynamic world of JavaScript. It’s an open-source language developed by Microsoft and extends JavaScript by adding optional static typing. TypeScript is transpiled into JavaScript, making it compatible with all JavaScript runtimes and browsers.
2.1. The Power of TypeScript
Type Safety: TypeScript enforces strong typing, catching type-related errors at compile time rather than runtime. This reduces bugs and enhances code quality.
- Editor Tooling: TypeScript provides excellent tooling support in modern code editors, offering features like auto-completion, type checking, and refactoring assistance.
- Readability: Strong typing makes code more self-documenting and easier to understand. Developers can quickly grasp the intent of functions and variables.
- Refactoring: TypeScript simplifies large-scale code refactoring, as it can accurately identify and update all references to a given symbol.
3. TypeScript in Microservices: A Natural Fit
Now, let’s dive into how TypeScript complements microservices architecture and contributes to building scalable and maintainable systems.
3.1. Service Isolation
In a microservices ecosystem, each service is a standalone component responsible for a specific function. TypeScript helps ensure that these services remain isolated by providing strong typing and well-defined interfaces. Here’s an example:
typescript // UserService.ts interface User { id: number; name: string; } class UserService { getUsers(): User[] { // Fetch users from the database // ... } } export default new UserService();
In this example, the UserService exposes a clear interface (getUsers) with well-defined input and output types. This separation ensures that any changes made to the service don’t inadvertently affect other parts of the application.
3.2. API Contracts
Microservices communicate with each other through APIs. TypeScript allows you to define and enforce these contracts effectively. Consider a scenario where a user service communicates with an order service. TypeScript enables you to specify the expected data structure:
typescript // User-to-Order API contract interface Order { orderId: number; userId: number; totalAmount: number; } class OrderService { createOrder(order: Order): void { // Create the order // ... } }
Here, TypeScript ensures that both services adhere to the agreed-upon contract, preventing runtime errors and mismatches.
3.3. Code Quality
Maintaining code quality is paramount in microservices, where each service operates independently. TypeScript’s static type checking minimizes runtime errors and ensures that your codebase remains reliable. Additionally, TypeScript’s readability aids in onboarding new team members and simplifies collaboration.
3.4. Ecosystem Compatibility
TypeScript seamlessly integrates with existing JavaScript libraries and frameworks. This compatibility means you can leverage TypeScript’s benefits without having to rewrite your entire codebase. Whether you’re using Node.js, Express, or a frontend framework like React or Angular, TypeScript has you covered.
3.5. Testing and Documentation
TypeScript makes it easier to write comprehensive unit tests for your microservices. With well-defined types and interfaces, you can create test cases that cover various scenarios, ensuring robustness and reliability.
Furthermore, TypeScript encourages inline documentation and comments, making it easier to understand the purpose and usage of each function or module. This is especially helpful when multiple teams collaborate on a large microservices project.
4. Implementing TypeScript in Microservices
Let’s walk through the process of implementing TypeScript in a microservices project.
4.1. Setting Up Your Environment
To get started, you’ll need Node.js installed on your system. You can initialize a TypeScript project using npm or yarn:
bash npm init -y npm install typescript --save-dev npx tsc --init
This sets up a tsconfig.json file, which you can customize based on your project’s needs.
4.2. Creating Services
Next, create individual microservices as separate TypeScript projects. You can structure your project as follows:
css my-microservices-app/ ??? user-service/ ? ??? src/ ? ? ??? index.ts ? ? ??? UserService.ts ? ??? package.json ? ??? tsconfig.json ??? order-service/ ? ??? src/ ? ? ??? index.ts ? ? ??? OrderService.ts ? ??? package.json ? ??? tsconfig.json ??? …
Each service should have its own tsconfig.json for specific configuration settings.
4.3. Defining Interfaces
In TypeScript, interfaces play a crucial role in defining contracts between services. Ensure that you create well-documented interfaces for all data structures exchanged between services.
4.4. Using a Service Registry
In a microservices architecture, services need to discover and communicate with each other. Consider using a service registry like Consul, Eureka, or etcd to manage service discovery and registration.
4.5. Continuous Integration and Deployment
Implement CI/CD pipelines for each microservice to automate testing and deployment. Tools like Jenkins, Travis CI, or GitHub Actions can help streamline this process.
4.6. Monitoring and Logging
Implement robust monitoring and logging solutions to track the health and performance of your microservices. Tools like Prometheus, Grafana, and ELK Stack can be invaluable in this regard.
4.7. Error Handling and Resilience
Design your microservices with error handling and resilience in mind. Implement retry mechanisms, circuit breakers, and fallback mechanisms to ensure the overall system’s reliability.
5. TypeScript and the Micro Frontend Revolution
While we’ve primarily focused on microservices on the backend, TypeScript also plays a pivotal role in the frontend microservices world. Micro frontend architecture decomposes the frontend of an application into smaller, independently deployable services, just like its backend counterpart.
Here’s how TypeScript contributes to micro frontends:
5.1. Strong Typing for Components
In micro frontends, each component is a standalone service. TypeScript provides strong typing for React, Angular, and other popular frontend frameworks, reducing runtime errors and enhancing the component’s reliability.
5.2. Code Splitting
TypeScript’s modular approach aligns well with code splitting techniques used in micro frontends. You can efficiently load only the code necessary for a particular view, improving page load times.
5.3. Shared Interfaces
In micro frontends, components often need to communicate and share data. TypeScript allows you to define shared interfaces that ensure compatibility and consistency across different components.
5.4. Team Collaboration
Just as in backend microservices, TypeScript’s readability and strong typing facilitate collaboration among frontend development teams working on different micro frontends.
Conclusion
TypeScript and microservices make a formidable pair in the quest to build scalable and maintainable software architectures. TypeScript’s type safety, code quality enhancements, and ecosystem compatibility perfectly complement the principles of microservices.
As you embark on your microservices journey, consider TypeScript as a valuable tool to harness the full potential of this architectural paradigm. Whether you’re developing backend microservices, frontend micro frontends, or both, TypeScript’s benefits will shine through in terms of productivity, reliability, and maintainability.
So, start exploring TypeScript for your microservices projects and experience the difference in code quality and scalability. The combination of TypeScript and microservices might just be the winning formula for your next software endeavor. Happy coding!
Table of Contents
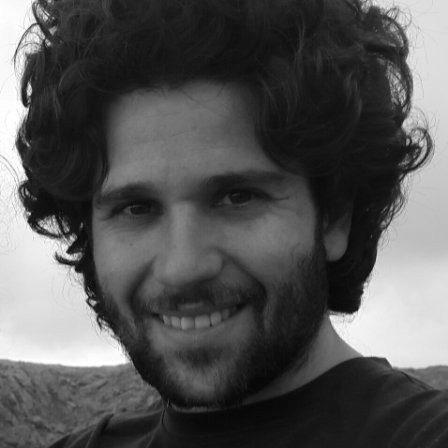
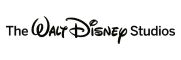