TypeScript and Natural Language Processing: Text Analytics
In a world overflowing with textual data, the ability to analyze and derive insights from this vast sea of information is invaluable. Natural Language Processing (NLP) is the field of study that empowers machines to understand, interpret, and generate human language. When coupled with TypeScript, a statically-typed superset of JavaScript, NLP becomes a potent tool for text analytics. In this blog, we will embark on a journey to explore how TypeScript and NLP combine forces to unlock the potential of text analytics.
Table of Contents
1. The Power of Text Analytics
Text analytics, also known as text mining or text data mining, is the process of deriving meaningful information from unstructured text data. This information can range from extracting keywords and phrases to sentiment analysis, making it a valuable tool for businesses, researchers, and organizations across various domains.
Some common applications of text analytics include:
- Customer Feedback Analysis: Analyzing customer reviews and feedback to gain insights into product or service quality.
- Social Media Monitoring: Tracking and understanding public sentiment, trends, and mentions on social media platforms.
- Content Categorization: Automatically categorizing and organizing large volumes of textual content.
- Information Extraction: Extracting structured information from unstructured text, such as extracting names, dates, and addresses from a document.
2. TypeScript: A Strong Foundation
TypeScript, developed by Microsoft, is a statically-typed superset of JavaScript. It adds static typing to JavaScript, which helps catch errors at compile-time rather than runtime, making code more reliable and maintainable. TypeScript’s strong typing system, combined with its tooling support, makes it an excellent choice for developing applications that require robustness and scalability.
In this blog, we will explore how TypeScript’s strengths can be harnessed in combination with Natural Language Processing (NLP) techniques to perform advanced text analytics.
3. Natural Language Processing Fundamentals
Before diving into TypeScript and its integration with NLP, it’s essential to understand some fundamental NLP concepts that we will be using in our text analytics journey.
3.1. Tokenization
Tokenization is the process of breaking a text into individual words or tokens. These tokens are the building blocks for various NLP tasks, such as text classification and sentiment analysis. Let’s see a TypeScript code sample for tokenization using the natural library:
typescript import natural from 'natural'; const tokenizer = new natural.WordTokenizer(); const text = "Tokenization is crucial for text analytics."; const tokens = tokenizer.tokenize(text); console.log(tokens); // Output: [ 'Tokenization', 'is', 'crucial', 'for', 'text', 'analytics', '.' ]
3.2. Part-of-Speech Tagging
Part-of-Speech tagging involves assigning a grammatical category (e.g., noun, verb, adjective) to each word in a text. TypeScript can be used to implement Part-of-Speech tagging with libraries like compromise-nlp:
typescript import nlp from 'compromise'; const doc = nlp('TypeScript is powerful.'); doc.sentences().tag(); console.log(doc.out('tags')); // Output: [ [ 'TypeScript', 'Noun' ], [ 'is', 'Verb' ], [ 'powerful', 'Adjective' ], [ '.', 'Punctuation' ] ]
3.3. Named Entity Recognition
Named Entity Recognition (NER) identifies and classifies entities such as names of people, organizations, locations, dates, and more in a text. The node-nlp library provides NER capabilities in TypeScript:
typescript import { NlpManager } from 'node-nlp'; const manager = new NlpManager({ languages: ['en'] }); manager.load(); const text = "Apple Inc. was founded by Steve Jobs in Cupertino."; const entities = manager.extractNamedEntities(text); console.log(entities); // Output: [ { entity: 'Apple Inc.', type: 'organization' }, { entity: 'Steve Jobs', type: 'person' }, { entity: 'Cupertino', type: 'location' } ]
3.4. Sentiment Analysis
Sentiment analysis aims to determine the sentiment or emotion expressed in a piece of text, typically classifying it as positive, negative, or neutral. Libraries like sentiment can be used for sentiment analysis in TypeScript:
typescript import sentiment from 'sentiment'; const text = "I love TypeScript. It's fantastic!"; const result = sentiment(text); console.log(result.score); // Output: 5 (positive sentiment)
These fundamental NLP techniques serve as building blocks for more complex text analytics tasks, and TypeScript provides a strong foundation to implement them effectively.
4. TypeScript for Text Analytics
4.1. Benefits of TypeScript
Using TypeScript in text analytics brings several advantages:
- Type Safety: TypeScript’s static typing system helps catch errors early in the development process, reducing the likelihood of runtime errors in your text analytics code.
- IntelliSense and Code Navigation: TypeScript-aware editors provide features like IntelliSense, code navigation, and autocompletion, making development more efficient and less error-prone.
- Maintainability: TypeScript’s readability and self-documenting nature make it easier to maintain and collaborate on text analytics projects.
- Ecosystem and Tooling: TypeScript integrates seamlessly with popular JavaScript libraries and frameworks, including NLP libraries, providing a vast ecosystem of tools and resources.
4.2. Setting Up a TypeScript Environment
To get started with TypeScript for text analytics, you’ll need to set up a TypeScript environment. Here are the steps:
- Install Node.js: If you haven’t already, install Node.js on your machine.
- Create a Project Directory: Create a directory for your TypeScript project and navigate to it using your terminal.
- Initialize a TypeScript Project: Run the following command to initialize a TypeScript project:
bash npm init -y
- Install TypeScript: Install TypeScript as a development dependency:
bash npm install typescript --save-dev
- Create a TypeScript Configuration File: Create a tsconfig.json file in your project directory. You can generate a basic configuration file by running:
bash npx tsc --init
Customize the tsconfig.json file according to your project’s needs.
- Install NLP Libraries: Depending on your text analytics requirements, install NLP libraries such as natural, compromise-nlp, or others as needed.
With your TypeScript environment set up, you’re ready to start building text analytics solutions.
5. Practical Text Analytics with TypeScript and NLP
In this section, we will walk through practical examples of text analytics tasks using TypeScript and NLP libraries.
5.1. Tokenization with TypeScript
Tokenization is the first step in many NLP tasks. We’ll use the natural library for tokenization:
typescript import natural from 'natural'; const tokenizer = new natural.WordTokenizer(); const text = "Tokenization is crucial for text analytics."; const tokens = tokenizer.tokenize(text); console.log(tokens); // Output: [ 'Tokenization', 'is', 'crucial', 'for', 'text', 'analytics', '.' ]
5.2. Part-of-Speech Tagging in TypeScript
Part-of-Speech tagging helps understand the grammatical structure of a sentence. Let’s use the compromise-nlp library for this task:
typescript import nlp from 'compromise'; const doc = nlp('TypeScript is powerful.'); doc.sentences().tag(); console.log(doc.out('tags')); // Output: [ [ 'TypeScript', 'Noun' ], [ 'is', 'Verb' ], [ 'powerful', 'Adjective' ], [ '.', 'Punctuation' ] ]
5.3. Named Entity Recognition with TypeScript
Named Entity Recognition is crucial for extracting entities from text. We’ll use the node-nlp library for NER:
typescript import { NlpManager } from 'node-nlp'; const manager = new NlpManager({ languages: ['en'] }); manager.load(); const text = "Apple Inc. was founded by Steve Jobs in Cupertino."; const entities = manager.extractNamedEntities(text); console.log(entities); // Output: [ { entity: 'Apple Inc.', type: 'organization' }, { entity: 'Steve Jobs', type: 'person' }, { entity: 'Cupertino', type: 'location' } ]
5.4. Sentiment Analysis Using TypeScript
Sentiment analysis helps gauge the sentiment in a text. We’ll use the sentiment library for this:
typescript import sentiment from 'sentiment'; const text = "I love TypeScript. It's fantastic!"; const result = sentiment(text); console.log(result.score); // Output: 5 (positive sentiment)
These examples showcase how TypeScript can be used with various NLP tasks to perform text analytics effectively.
6. Challenges and Considerations
While TypeScript and NLP offer powerful capabilities for text analytics, there are some challenges and considerations to keep in mind:
6.1. Handling Large Text Corpora
Analyzing large volumes of text data can be resource-intensive. It’s essential to optimize your code and consider techniques like distributed processing for scalability.
6.2. Choosing the Right NLP Library
Selecting the appropriate NLP library depends on your specific requirements. Consider factors such as language support, ease of use, and performance when choosing an NLP library for your project.
6.3. Performance Optimization
Optimizing the performance of your text analytics code is crucial, especially when dealing with real-time data streams or large datasets. Profiling your code and utilizing parallel processing can help improve performance.
Conclusion
The synergy of TypeScript and Natural Language Processing opens up exciting possibilities for text analytics. TypeScript’s strong typing system, combined with the capabilities of NLP libraries, empowers developers to build robust and efficient text analysis solutions. As the field of NLP continues to evolve, TypeScript will play a vital role in harnessing its potential. By leveraging the knowledge and practical examples shared in this blog, you can embark on your text analytics journey with confidence, extracting valuable insights from the vast world of textual data.
Table of Contents
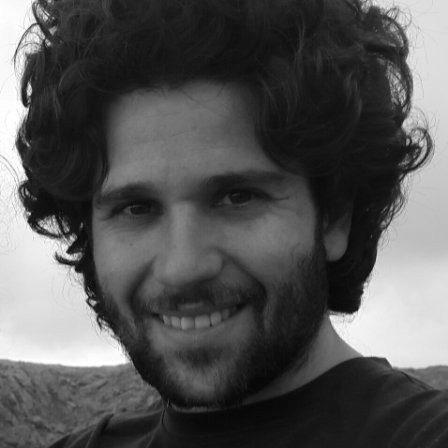
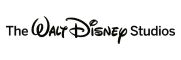