Promises and Async/Await in TypeScript
Asynchronous programming is a crucial aspect of modern web development. It allows us to perform tasks without blocking the execution of other code. JavaScript, the language of the web, has evolved significantly to handle asynchronous operations seamlessly. One of the most powerful features that facilitate asynchronous programming in TypeScript is the combination of Promises and Async/Await.
In this blog, we will explore the concepts of Promises and Async/Await in TypeScript, their benefits, and how they can simplify asynchronous code. We’ll dive into their usage, understand their differences, and see how to handle errors effectively. By the end, you’ll be equipped to write clean and maintainable asynchronous code in your TypeScript projects.
1. Understanding Promises
1.1 What are Promises?
Promises are a modern approach to handling asynchronous operations in JavaScript and TypeScript. They represent a value that may not be available yet but will be resolved at some point in the future. A Promise can be in one of three states:
- Pending: The initial state; the Promise is still being processed.
- Fulfilled: The asynchronous operation has completed successfully, and the Promise has a resolved value.
- Rejected: The asynchronous operation has failed, and the Promise has a reason for the failure.
1.2 Creating a Promise
In TypeScript, creating a Promise is straightforward. Let’s say we have a function fetchData that fetches data from an API. We can convert it into a Promise using the Promise constructor:
typescript function fetchData(): Promise<string> { return new Promise((resolve, reject) => { // Simulating an API call with setTimeout setTimeout(() => { const data = "Hello, TypeScript Promises!"; // Resolve the Promise with the fetched data resolve(data); }, 2000); }); }
In the example above, fetchData returns a Promise that resolves with a string after a simulated delay of 2 seconds.
1.3 Consuming a Promise
To use the resolved value of a Promise, we use the .then() method. The .then() method takes two callback functions: one for success (onFulfilled) and one for failure (onRejected). In most cases, we only provide the success callback.
typescript fetchData().then((data) => { console.log(data); // Output: "Hello, TypeScript Promises!" });
1.4 Handling Errors with Promises
Handling errors with Promises is done using the .catch() method. If the Promise is rejected, the .catch() method will be called with the reason for the rejection.
typescript function fetchError(): Promise<never> { return new Promise((resolve, reject) => { setTimeout(() => { // Simulating an error during the API call reject(new Error("Failed to fetch data.")); }, 2000); }); } fetchError() .then((data) => { // This will not execute }) .catch((error) => { console.error(error.message); // Output: "Failed to fetch data." });
2. Introducing Async/Await
2.1 What is Async/Await?
Async/Await is a syntax built on top of Promises that provides a more concise and synchronous-looking way of dealing with asynchronous operations. It allows developers to write asynchronous code that resembles synchronous code, making it easier to read and maintain.
2.2 Using the async Keyword
To define an asynchronous function, we use the async keyword before the function declaration:
typescript async function fetchAsyncData(): Promise<string> { const data = await fetchData(); return data; }
In the example above, fetchAsyncData is an asynchronous function that calls the fetchData function and awaits its result.
2.3 Awaiting the Result
The await keyword can only be used inside an async function. It pauses the execution of the function until the awaited Promise is resolved or rejected. When the Promise is resolved, the value is returned. If the Promise is rejected, an error is thrown.
typescript async function printData(): Promise<void> { try { const data = await fetchAsyncData(); console.log(data); // Output: "Hello, TypeScript Promises!" } catch (error) { console.error(error.message); } } printData();
In the example above, printData is an asynchronous function that awaits the result of fetchAsyncData and then logs the data to the console.
3. Benefits of Async/Await
Using Promises and Async/Await in TypeScript offers several advantages:
3.1 Readability and Maintainability
Async/Await allows developers to write asynchronous code in a more sequential and synchronous style. This improves code readability and makes it easier to understand and maintain, especially for complex asynchronous operations.
3.2 Error Handling
Async/Await simplifies error handling by allowing us to use try-catch blocks. This provides a more natural way to handle errors and avoids deeply nested error handling commonly seen with Promises.
3.3 Debugging
Debugging asynchronous code can be challenging. Async/Await provides a better debugging experience as it allows you to set breakpoints and inspect variables at each step of execution.
3.4 Chaining Asynchronous Operations
Async/Await allows for better control flow and easy chaining of asynchronous operations. This is especially useful when multiple asynchronous tasks depend on the results of each other.
3.5 Compatibility with Promises
Async/Await is built on top of Promises, making it fully compatible with existing Promise-based code. You can mix and match Promises and Async/Await in your TypeScript project as needed.
4. Async/Await vs. Promises
Both Async/Await and Promises are essential tools for handling asynchronous operations in TypeScript. Understanding when to use each approach is crucial for writing clean and efficient code.
4.1 Promises:
- Promises are well-suited for handling a single asynchronous operation or when parallel execution is required.
- They allow for better composability when chaining multiple asynchronous operations.
- Error handling is done using .then() and .catch() methods.
4.2 Async/Await:
- Async/Await is ideal for writing sequential and easy-to-read asynchronous code.
- It simplifies error handling with try-catch blocks.
- Best suited for cases where multiple asynchronous operations depend on the results of each other.
Choosing between Promises and Async/Await depends on the specific requirements of your project and your personal coding style. Often, a combination of both approaches can lead to the best results.
Conclusion
Asynchronous programming is a critical aspect of modern web development, and TypeScript provides powerful tools like Promises and Async/Await to handle it effectively. By using Promises, you can create efficient and composable asynchronous code, while Async/Await offers a more readable and synchronous-style alternative. Understanding when and how to use each approach will enable you to write cleaner, more maintainable, and error-resilient code.
Embrace the power of Promises and Async/Await in TypeScript, and take your asynchronous programming skills to the next level! Happy coding!
Table of Contents
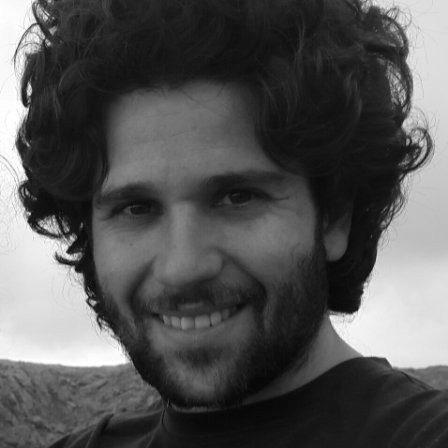
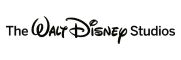