Real-Time Applications with TypeScript and Socket.io
In today’s fast-paced digital world, real-time communication and interactivity have become essential components of modern web applications. From collaborative tools to live notifications, real-time updates are expected by users. This is where technologies like TypeScript and Socket.io come into play. In this article, we will delve into the realm of real-time applications, discovering how TypeScript and Socket.io can be combined to create seamless and interactive user experiences.
Table of Contents
1. Understanding Real-Time Communication
Before we dive into the technical details, let’s understand what real-time communication entails. Real-time communication involves delivering information or updates to users as soon as they occur, without any noticeable delay. Think of a messaging app where you see messages instantly as they are sent, or a collaborative document editing tool where changes from multiple users appear in real time. Achieving this level of immediacy requires efficient communication between clients and servers.
2. Introducing Socket.io
Socket.io is a JavaScript library that simplifies real-time communication between clients and servers. It works over the WebSocket protocol, which provides full-duplex communication channels over a single TCP connection. This means that both the client and server can send data to each other simultaneously.
Socket.io not only supports WebSockets but also gracefully falls back to other transport mechanisms like long polling for environments where WebSockets are not available. This makes it highly versatile and suitable for a wide range of applications.
2.1. Setting Up a TypeScript Project
Before we proceed, let’s set up a basic TypeScript project:
bash # Create a new directory for your project mkdir RealTimeAppWithSocketIO # Move into the project directory cd RealTimeAppWithSocketIO # Initialize a package.json file npm init -y # Install TypeScript and ts-node npm install typescript ts-node --save-dev # Create a tsconfig.json file npx tsc --init # Create a src directory for your TypeScript files mkdir src # Create your entry TypeScript file, e.g., index.ts touch src/index.ts
2.2. Installing and Configuring Socket.io
To start using Socket.io in your project, you need to install it and set up a server. Follow these steps:
- Install Socket.io and its types:
bash npm install socket.io @types/socket.io --save
2. Create an Express server and integrate Socket.io:
typescript // src/index.ts import express from 'express'; import http from 'http'; import { Server } from 'socket.io'; const app = express(); const server = http.createServer(app); const io = new Server(server); const PORT = process.env.PORT || 3000; server.listen(PORT, () => { console.log(`Server is running on port ${PORT}`); });
3. Now that you have your basic server set up, you can start integrating Socket.io into your TypeScript project.
2.3. Establishing Real-Time Connections
Socket.io allows you to establish real-time connections between clients and the server. Clients can send and receive events, making it ideal for implementing features like live chats and notifications. Here’s how you can establish a connection and exchange messages:
- Set up the client-side code:
html <!-- src/index.html --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Socket.io Chat</title> </head> <body> <script src="/socket.io/socket.io.js"></script> <script> const socket = io(); // Send a message to the server socket.emit('message', 'Hello, server!'); // Receive a message from the server socket.on('message', (message) => { console.log('Message from server:', message); }); </script> </body> </html>
2. Set up the server-side code:
typescript // src/index.ts // ... (previous code) io.on('connection', (socket) => { console.log('A user connected'); // Listen for 'message' events from the client socket.on('message', (message) => { console.log('Message from client:', message); // Send a response back to the client socket.emit('message', 'Hello, client!'); }); // Disconnect event socket.on('disconnect', () => { console.log('A user disconnected'); }); });
3. Emitting and Receiving Events
In a real-time application, events play a crucial role. Socket.io allows you to emit and receive custom events, enabling seamless communication between clients and servers. Let’s explore how to emit and receive events:
- Emitting events from the client:
javascript // Inside the client-side script const data = { username: 'Alice', message: 'Hello, server!' }; // Emit a 'chat message' event to the server socket.emit('chat message', data);
2. Handling events on the server:
typescript // Inside the server-side code io.on('connection', (socket) => { // ... // Listen for 'chat message' events from the client socket.on('chat message', (data) => { console.log(`Message from ${data.username}: ${data.message}`); // Broadcast the message to all connected clients io.emit('chat message', data); }); // ... });
3. Receiving events on the client:
javascript // Inside the client-side script // Listen for 'chat message' events from the server socket.on('chat message', (data) => { console.log(`Message from ${data.username}: ${data.message}`); });
4. Use Cases of Real-Time Applications
Real-time applications built with TypeScript and Socket.io can revolutionize user experiences in various domains:
4.1. Live Chats and Collaboration
Implementing live chat functionality in your applications becomes straightforward with Socket.io. Users can exchange messages in real time, creating a seamless communication experience. Similarly, collaborative tools like Google Docs can leverage real-time updates to ensure that changes made by multiple users are instantly reflected.
4.2. Notifications and Alerts
Real-time notifications keep users informed about important events as soon as they happen. Whether it’s a new email, a social media mention, or an update to a subscribed topic, real-time notifications enhance user engagement and satisfaction.
4.3. Online Gaming
Online multiplayer games heavily rely on real-time communication between players. Socket.io can power features like in-game chats, live score updates, and player interactions, enhancing the gaming experience by making it more interactive and engaging.
Conclusion
In this article, we’ve explored the exciting realm of real-time applications using TypeScript and Socket.io. We started by understanding the significance of real-time communication in modern applications and introduced the Socket.io library. We walked through the process of setting up a TypeScript project, integrating Socket.io into both the server and client sides, and establishing real-time connections.
Additionally, we delved into emitting and receiving events to facilitate seamless communication between clients and servers. We discussed various use cases of real-time applications, from live chats and collaboration to notifications and online gaming.
The power of real-time applications lies in their ability to provide users with instant updates and interactive experiences. Whether you’re building a messaging app, a collaborative tool, or an online game, TypeScript and Socket.io can empower you to create dynamic and engaging applications that keep users connected and engaged like never before. So, why wait? Dive into the world of real-time applications and unlock a new dimension of user experiences.
Table of Contents
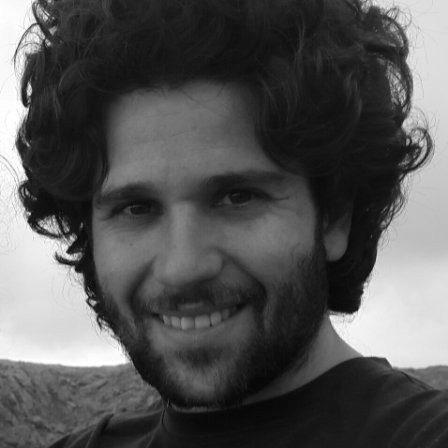
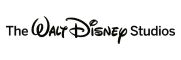