Testing TypeScript Code: Strategies and Best Practices
In the world of modern software development, testing plays a pivotal role in ensuring the reliability, maintainability, and robustness of code. TypeScript, a statically typed superset of JavaScript, has gained significant popularity due to its ability to catch errors at compile time and enhance code quality. However, writing TypeScript code is just the beginning; comprehensive testing is essential to validate the functionality of your applications. In this article, we will dive into strategies and best practices for testing TypeScript code, along with code samples and tools to streamline the testing process.
Table of Contents
1. Why Test TypeScript Code?
Testing TypeScript code offers numerous advantages that contribute to the overall success of your software projects:
1.1. Early Bug Detection:
TypeScript’s static typing helps catch many errors at compile time. However, runtime errors and logical issues can still occur. Tests provide an additional layer of confidence by identifying issues early in the development process.
1.2. Code Confidence:
Robust test suites increase the developers’ confidence in making changes to the codebase. Refactoring or adding new features becomes less daunting when a solid suite of tests is in place.
1.3. Maintainability:
Tests act as living documentation for your codebase. They provide insights into the expected behavior of different components, making it easier for new developers to understand and contribute to the project.
1.4. Regression Prevention:
As your codebase evolves, new features or bug fixes might inadvertently introduce regressions. A comprehensive test suite helps prevent these regressions by ensuring that existing functionality remains intact.
2. Testing Strategies
When it comes to testing TypeScript code, there are several strategies and levels of testing that you can employ:
2.1. Unit Testing:
Unit testing focuses on testing individual units or components of your code in isolation. Mocks and stubs are often used to isolate dependencies. It helps verify that each unit works as expected and adheres to its design.
2.2. Integration Testing:
Integration testing involves testing interactions between different components or modules. It ensures that these components work together seamlessly and fulfill the desired functionality.
2.3. End-to-End Testing:
End-to-end (E2E) testing simulates real user scenarios and interactions with the application. It helps validate the entire application flow and is particularly useful for catching issues that might arise from the integration of different components.
2.4. Snapshot Testing:
Snapshot testing captures the output of a component and compares it to a previously stored “snapshot.” It’s particularly effective for UI components, ensuring that changes to the UI are intentional and expected.
3. Best Practices for Testing TypeScript Code
To create effective tests for your TypeScript code, consider the following best practices:
3.1. Use Descriptive Test Names:
Choose descriptive and meaningful names for your test cases. This makes it easier to understand the purpose of the test and the expected behavior.
3.2. Arrange-Act-Assert (AAA) Pattern:
Follow the AAA pattern for structuring your test cases. Arrange the necessary prerequisites, perform the action, and then assert the expected outcomes.
typescript // Example of the AAA pattern in a unit test test("calculateTotalPrice should correctly sum the prices", () => { // Arrange const items = [{ price: 10 }, { price: 20 }, { price: 30 }]; // Act const total = calculateTotalPrice(items); // Assert expect(total).toBe(60); });
3.3. Use Mocks and Stubs:
When unit testing, use mocks and stubs to isolate dependencies. This ensures that you’re testing the specific unit’s behavior without interference from external components.
3.4. Implement Test Coverage:
Strive for high test coverage to ensure that your tests cover as much of your codebase as possible. This helps identify untested or poorly tested areas.
3.5. Continuous Integration (CI) Testing:
Integrate your tests into your CI/CD pipeline. Automated tests run on every code commit, providing rapid feedback and preventing the introduction of bugs into the main codebase.
3.6. Use Testing Frameworks:
Choose a testing framework that suits your project, such as Jest or Mocha. These frameworks offer powerful assertion libraries and utilities for test setup and teardown.
4. Testing Tools for TypeScript
There are several tools available that can streamline your TypeScript testing workflow:
4.1. Jest:
Jest is a widely used testing framework that comes with built-in mocking, assertion, and snapshot testing capabilities. It’s particularly popular for React applications but can be used for testing TypeScript code in general.
typescript // Example of a Jest test test("add function should correctly add two numbers", () => { expect(add(2, 3)).toBe(5); });
4.2. Mocha:
Mocha is another flexible testing framework that provides support for different assertion libraries and allows you to customize your testing setup.
4.3. Chai:
Chai is an assertion library often used with Mocha. It provides various assertion styles (should, expect, assert) to match your preferred testing style.
4.4. Cypress:
Cypress is a powerful E2E testing framework that enables you to write tests that simulate user interactions and behaviors. It’s especially useful for testing complex frontend applications.
typescript // Example of a Cypress E2E test it("should navigate to the login page and successfully log in", () => { cy.visit("/login"); cy.get("#username").type("user123"); cy.get("#password").type("password123"); cy.get("#login-button").click(); cy.url().should("include", "/dashboard"); });
4.5. Testing Utilities:
For more specialized testing needs, you can utilize libraries like @testing-library/react for React components or ts-mockito for mocking TypeScript classes.
Conclusion
Testing TypeScript code is an integral part of delivering high-quality software. It boosts confidence, prevents regressions, and enhances the overall maintainability of your codebase. By incorporating various testing strategies, following best practices, and leveraging powerful testing tools, you can ensure that your TypeScript applications are reliable, robust, and ready to meet user expectations. Remember, the investment you make in testing pays off in the long run by reducing bugs, increasing developer efficiency, and delivering a seamless user experience. So, go ahead and start building those comprehensive test suites to propel your TypeScript projects to success!
Table of Contents
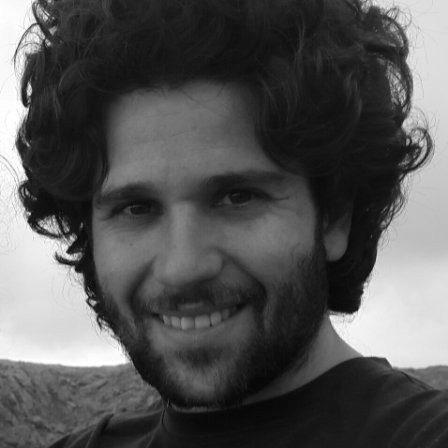
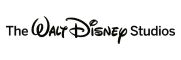