Exploring Third-Party Libraries in TypeScript
TypeScript has quickly gained popularity in the world of web development due to its static typing and object-oriented features. One of the major advantages of TypeScript is its compatibility with third-party libraries, allowing developers to easily integrate powerful tools and functionalities into their projects. In this blog, we will delve into the world of third-party libraries in TypeScript, exploring how they can boost productivity and streamline the development process.
Table of Contents
1. The Role of Third-Party Libraries
Third-party libraries are pre-written packages of code created by other developers that address common programming tasks. These libraries provide a wealth of pre-built functionalities that developers can easily integrate into their projects, saving time and effort. TypeScript’s strong typing and compatibility with JavaScript make it an excellent choice for utilizing third-party libraries, as it provides better type checking and improved documentation.
2. Benefits of Using Third-Party Libraries in TypeScript
2.1. Accelerated Development
Integrating third-party libraries allows developers to skip the time-consuming process of building functionalities from scratch. These libraries are created by experts in the field, ensuring high-quality code that has been tested and refined over time. By utilizing these ready-made solutions, developers can focus on the unique aspects of their projects and bring them to completion faster.
2.2. Improved Code Quality
TypeScript’s static typing feature plays a crucial role when working with third-party libraries. The strong type checking helps catch errors at compile time rather than runtime, reducing the likelihood of bugs and enhancing overall code quality. This results in more maintainable and stable applications.
2.3. Ecosystem and Community
The TypeScript community is active and vibrant, continuously creating and updating third-party libraries. This thriving ecosystem means developers have access to a wide range of libraries, each catering to different needs. Whether it’s for UI components, state management, or API interactions, chances are there’s already a library available to simplify the task.
3. Choosing the Right Third-Party Libraries
While the benefits of using third-party libraries are evident, it’s essential to choose the right ones for your project. Here’s a step-by-step guide:
3.1. Identify Your Needs
Before integrating any library, identify the specific needs of your project. Are you looking for a UI framework, data visualization tools, or state management solutions? Having a clear understanding of your requirements will help you narrow down your options.
3.2. Research and Evaluate
Thoroughly research and evaluate different libraries that cater to your needs. Consider factors like documentation, community support, and active development. A well-maintained library with comprehensive documentation and a large community is usually a safe bet.
3.3. Compatibility with TypeScript
Ensure that the library you’re considering is compatible with TypeScript. Many popular libraries already have TypeScript type definitions available, which greatly simplifies the integration process and enhances type safety.
3.4. Check for Customization
Examine the library’s customization options. Every project is unique, so it’s crucial to choose a library that allows you to tailor its functionalities to fit your specific requirements.
3.5. Performance Considerations
While third-party libraries can speed up development, they can also impact performance if not used judiciously. Test the library’s performance in different scenarios to ensure it meets your application’s performance requirements.
4. Integrating Third-Party Libraries into TypeScript Projects
Integrating third-party libraries into TypeScript projects is generally straightforward, thanks to the extensive TypeScript type definition ecosystem. Here’s a basic guide:
4.1. Installing Libraries
Use a package manager like npm or yarn to install the desired library. For example:
bash npm install library-name
4.2. Type Definitions
Check if the library has official TypeScript type definitions (usually available as @types/library-name). If not, you might find community-contributed type definitions.
4.3. Importing and Using
Import the library into your TypeScript code and start using its functionalities. Make sure to follow the library’s documentation for proper usage.
typescript import { SomeFunction } from 'library-name'; const result = SomeFunction(parameter);
4.4. Type Safety
TypeScript’s type definitions ensure type safety while using third-party libraries. This helps catch potential errors during development rather than at runtime.
5. Commonly Used Third-Party Libraries in TypeScript
Let’s explore some popular third-party libraries that TypeScript developers frequently use:
5.1. React
For building user interfaces, React is a top choice among developers. Its component-based architecture aligns well with TypeScript’s static typing, resulting in robust and maintainable UI code.
5.2. Redux
When it comes to state management, Redux remains a widely adopted solution. TypeScript’s typing capabilities complement Redux’s concepts, providing a reliable and predictable state management solution.
5.3. Axios
For handling HTTP requests, Axios is a popular choice. Its simplicity and TypeScript support make it an efficient tool for interacting with APIs.
5.4. Lodash
Lodash offers a plethora of utility functions that simplify common programming tasks. TypeScript’s compatibility ensures type safety while using Lodash’s functions.
Conclusion
Incorporating third-party libraries into TypeScript projects can significantly enhance development speed, code quality, and functionality. By leveraging the power of the TypeScript ecosystem, developers can tap into a wide array of pre-built solutions, allowing them to focus on building unique and innovative features. Remember to research thoroughly, choose wisely, and make the most out of TypeScript’s type safety to ensure a seamless integration process. Happy coding!
Table of Contents
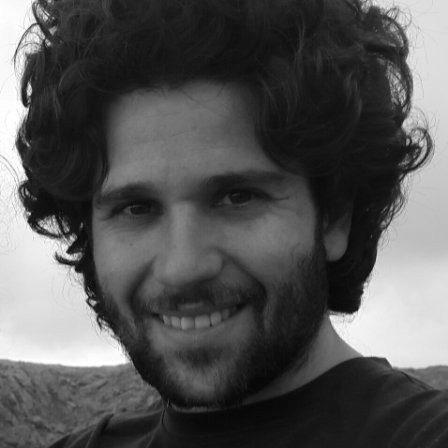
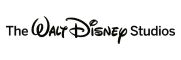