Using Enums in TypeScript: Simplifying Code
In the world of programming, simplicity is a cherished virtue. As projects grow and codebases expand, maintaining clean, readable, and maintainable code becomes essential. This is where TypeScript, a statically typed superset of JavaScript, comes into play. TypeScript offers various features that enhance code quality and organization, and one such feature is enums. Enums provide an elegant way to represent a set of related constants, making your code more expressive and reducing the chances of errors. In this article, we’ll dive into the world of enums in TypeScript and explore how they can simplify your codebase.
Table of Contents
1. Understanding Enums
1.1. What are Enums?
An enum, short for enumeration, is a data type in TypeScript that allows you to define a set of named constants. These constants represent a finite set of possible values. Enums provide a clear and organized way to work with predefined values, enhancing both code readability and maintainability.
1.2. Enum Syntax
The syntax for declaring an enum in TypeScript is straightforward:
typescript enum Days { Sunday, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday }
In this example, we’ve defined an enum named Days that represents the days of the week. Each day is assigned a numeric value, starting from 0 by default. It’s important to note that you can assign specific numeric values to enum members if needed:
typescript enum Days { Sunday = 1, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday }
2. Benefits of Using Enums
2.1. Improved Readability
Enums provide meaningful names to represent values, making your code more human-readable. Imagine encountering the value 3 in your code without context. Now, consider encountering Days.Wednesday instead. The latter is not only clearer but also easier to understand.
2.2. Type Safety
TypeScript is all about static typing, and enums fit perfectly into this paradigm. When you use an enum value, TypeScript ensures that you’re using a valid value from the predefined set. This helps catch errors at compile time, reducing the likelihood of runtime issues.
2.3. Auto-completion and Intellisense
IDEs and code editors that support TypeScript can provide auto-completion and intellisense for enum members. This not only boosts your productivity but also minimizes mistakes related to typos or incorrect values.
3. Practical Use Cases
3.1. Switch Statements
Enums shine when used in switch statements. Consider a scenario where you’re handling different actions based on user input. Using enums can make your code cleaner and more maintainable:
typescript enum ActionTypes { Add, Edit, Delete } function performAction(action: ActionTypes) { switch (action) { case ActionTypes.Add: // Logic for adding break; case ActionTypes.Edit: // Logic for editing break; case ActionTypes.Delete: // Logic for deleting break; default: // Default case } }
3.2. Configuration Options
Enums are great for representing configuration options in your application. Let’s say you have various settings for a user’s profile visibility. Instead of using magic numbers or strings, enums provide a clear structure:
typescript enum ProfileVisibility { Public, Friends, Private } const userSettings = { profileVisibility: ProfileVisibility.Friends };
3.3. Status Codes
HTTP status codes are a common use case for enums. Instead of memorizing status code numbers, you can use enums to make your code more understandable:
typescript enum HttpStatusCode { OK = 200, BadRequest = 400, Unauthorized = 401, NotFound = 404, InternalServerError = 500 }
4. Enums and Enums Members Behind the Scenes
Under the hood, enums are more than just named constants. They are objects with both forward and reverse mappings. This means you can access the enum value by its name as well as access the enum name by its value. For example:
typescript enum Colors { Red, Green, Blue } const colorName: string = Colors[1]; // Returns 'Green' const colorValue: number = Colors.Green; // Returns 1
5. Enums vs. Union Types
While enums offer several benefits, there are situations where union types might be a better fit. Union types allow you to define a type that can be one of several possible types. They are often used when the set of possible values is not fixed or when you need to combine different types.
For instance, if you’re dealing with colors that could be strings or hex values, a union type might be more suitable:
typescript type Color = string | number; const textColor: Color = "#FF5733"; const bgColor: Color = 16777215; // Hex value for white
6. Best Practices
To make the most of enums in TypeScript, consider the following best practices:
- Use Descriptive Names: Choose meaningful names for your enum and its members to enhance code readability.
- Start with Zero: Unless you have a compelling reason, let your enum members start from 0 for consistency.
- Explicit Values: If your enum values have a specific meaning, assign them explicit numeric values.
- Consider Alternatives: While enums are powerful, assess whether a union type or another approach might be more appropriate for your specific use case.
Conclusion
Enums in TypeScript offer a powerful tool for simplifying code by providing a clear and organized way to represent predefined values. With their enhanced readability, type safety, and versatility, enums help you write more maintainable and error-resistant code. By understanding how to use enums effectively and following best practices, you can take advantage of this feature to streamline your TypeScript projects and create code that is both elegant and robust. So, the next time you encounter a set of related constants, consider reaching for enums to simplify your TypeScript codebase.
Table of Contents
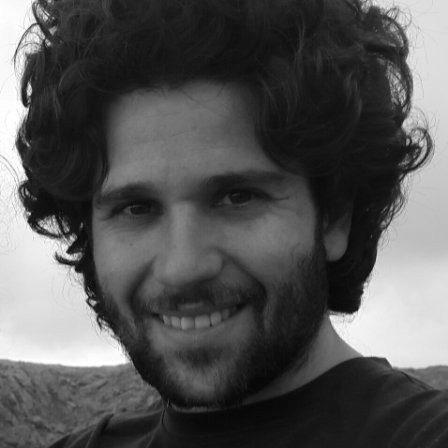
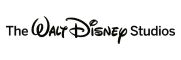