TypeScript and React: A Winning Combination
In the ever-evolving world of web development, the need for efficient and maintainable code is paramount. Enter TypeScript and React, two technologies that, when combined, form a potent alliance that greatly enhances the development process and produces robust applications. In this blog post, we’ll delve into the marriage of TypeScript and React, exploring their synergies, benefits, key features, and even provide code samples to showcase their prowess.
Table of Contents
1. Understanding TypeScript and React
Before we dive into the reasons why TypeScript and React make a winning combination, let’s briefly understand what each of these technologies brings to the table.
2. TypeScript: Adding Superpowers to JavaScript
TypeScript is a superset of JavaScript that introduces static typing. It allows developers to define the types of variables, function parameters, and return values. This additional layer of type checking catches potential errors at compile-time, providing improved code quality and better developer experience. TypeScript doesn’t replace JavaScript; rather, it builds upon it, compiling down to plain JavaScript that runs in any browser or environment.
3. React: Declarative and Component-Based UI
React, on the other hand, is a JavaScript library for building user interfaces. It follows a declarative approach, enabling developers to describe the desired UI state, and React takes care of efficiently updating the actual UI to match that state. React’s component-based architecture promotes reusability, modularity, and easier maintenance of UI elements. With its Virtual DOM, React minimizes performance bottlenecks by efficiently updating only the necessary parts of the UI.
4. The Synergy of TypeScript and React
4.1. Type Safety for React Components
One of the primary advantages of using TypeScript with React is the enhanced type safety it brings to the development process. TypeScript allows developers to define the types of props and state within React components. This not only improves code readability but also catches type-related errors during development, reducing runtime errors and improving application stability.
tsx // TypeScript React Component interface Props { name: string; age: number; } const UserProfile: React.FC<Props> = ({ name, age }) => { return ( <div> <p>Name: {name}</p> <p>Age: {age}</p> </div> ); };
4.2. Intelligent Autocomplete and Code Navigation
TypeScript’s type inference capabilities come to the forefront when used with React. Integrated development environments (IDEs) like Visual Studio Code can provide intelligent autocomplete suggestions, helping developers write correct code faster. Additionally, TypeScript’s static typing enables powerful code navigation features, making it easier to understand complex codebases and find relevant components and functions.
4.3. Early Detection of Errors
By catching errors at compile-time, TypeScript prevents many common mistakes from making their way into the final application. This early error detection saves developers time and effort that would otherwise be spent tracking down bugs during runtime. The combination of TypeScript and React results in fewer surprises and more predictable behavior.
4.4. Refactoring Made Easy
Large React applications often require refactoring to keep the codebase maintainable. TypeScript aids in this process by providing clear insights into the relationships between components and their interfaces. When changes are made to a component’s interface, TypeScript flags the areas in the codebase that need to be updated, reducing the risk of introducing bugs during refactoring.
5. Getting Started: Setting Up TypeScript with React
Now that we’ve explored the benefits of using TypeScript and React together, let’s walk through the process of setting up a project that leverages this powerful combination.
Step 1: Create a React App
If you’re starting from scratch, create a new React app using the following command:
bash npx create-react-app my-typescript-app
Step 2: Install TypeScript
Navigate to your project directory and install TypeScript:
bash npm install typescript @types/react @types/react-dom
Step 3: Configuration
Create a tsconfig.json file in the root of your project. This file configures TypeScript settings for your project. Here’s a basic configuration to get you started:
json { "compilerOptions": { "target": "ES6", "module": "ESNext", "strict": true, "jsx": "react-jsx", "esModuleInterop": true } }
Step 4: Renaming Files
Rename your .js and .jsx files to .ts and .tsx, respectively, to indicate that they now contain TypeScript code.
Step 5: Start Coding!
You’re now set up to write React components using TypeScript. Remember to define interfaces for your props and states to fully leverage TypeScript’s type checking capabilities.
6. Leveraging TypeScript Features in React
Let’s take a closer look at how TypeScript features can be effectively utilized within React components.
6.1. Defining Props and State Interfaces
tsx interface UserProfileProps { name: string; age: number; } interface UserProfileState { isActive: boolean; }
6.2. Using Props and State
tsx class UserProfile extends React.Component<UserProfileProps, UserProfileState> { // ... }
6.3. Functional Components with Hooks
tsx interface TimerProps { interval: number; } const Timer: React.FC<TimerProps> = ({ interval }) => { const [seconds, setSeconds] = React.useState(0); React.useEffect(() => { const timer = setInterval(() => { setSeconds(prevSeconds => prevSeconds + 1); }, interval); return () => clearInterval(timer); }, [interval]); return <p>Seconds Elapsed: {seconds}</p>; };
Conclusion
The combination of TypeScript and React is undoubtedly a winning one. TypeScript’s static typing, intelligent autocompletion, and early error detection seamlessly integrate with React’s component-based architecture and declarative UI approach. This alliance empowers developers to build robust, maintainable, and efficient web applications with fewer bugs and increased productivity. As you embark on your TypeScript and React journey, remember to embrace TypeScript’s type safety and React’s reusability to unlock the full potential of this dynamic duo in modern web development.
In conclusion, by harnessing the power of TypeScript and React together, you’re not only building today’s applications but also laying the foundation for scalable and adaptable solutions in the rapidly evolving landscape of web development.
Table of Contents
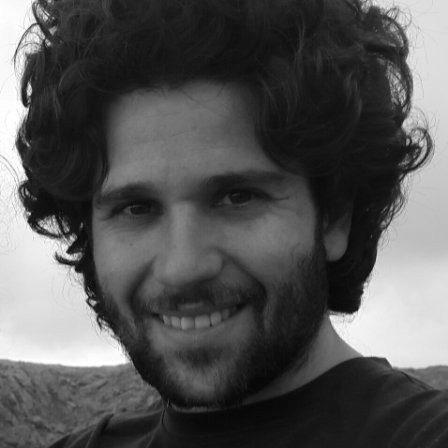
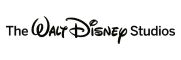