Vue.js and TypeScript: Supercharging Your Development
In the ever-evolving landscape of web development, staying ahead of the curve is essential. Vue.js and TypeScript are two technologies that have gained immense popularity due to their ability to simplify and streamline the development process. When combined, they offer a dynamic duo that can supercharge your web development projects. In this article, we’ll delve into the world of Vue.js and TypeScript, exploring how their synergy can elevate your coding experience and the quality of your applications.
Table of Contents
1. Understanding Vue.js and TypeScript
1.1. Vue.js: A Breath of Fresh Air in Frontend Development
Vue.js is a progressive JavaScript framework that has taken the frontend world by storm. Known for its simplicity and versatility, Vue.js empowers developers to build robust and interactive user interfaces with ease. It follows a component-based architecture, allowing you to encapsulate different parts of your application into reusable components, resulting in a more modular and maintainable codebase.
vue <template> <div> <h1>{{ message }}</h1> <button @click="incrementCount">Increment</button> </div> </template> <script> export default { data() { return { message: 'Hello, Vue.js!', count: 0, }; }, methods: { incrementCount() { this.count++; }, }, }; </script>
2. TypeScript: Typing Magic for JavaScript
TypeScript, on the other hand, is a superset of JavaScript that brings static typing to the language. By introducing type annotations, interfaces, and other advanced type-related features, TypeScript helps catch errors at compile-time rather than runtime. This leads to more robust and maintainable code, as well as improved developer productivity through enhanced code intelligence in IDEs.
typescript interface User { id: number; username: string; email: string; } function greetUser(user: User): string { return `Hello, ${user.username}!`; }
3. The Synergy: Why Combine Vue.js and TypeScript?
3.1. Enhanced Developer Experience
Combining Vue.js and TypeScript can significantly enhance your developer experience. Vue.js’s component-based structure aligns well with TypeScript’s type system. With TypeScript, you’ll receive autocompletion, better code navigation, and early error detection. This means spending less time debugging and more time building.
3.2. Safer Codebase
TypeScript’s static typing eliminates a large portion of runtime errors by catching them during development. When applied to Vue.js components, this means that you’re less likely to encounter type-related issues or unexpected behavior at runtime. This safety net ensures your application behaves as expected, giving you more confidence in your code.
3.3. Improved Collaboration
TypeScript’s type annotations serve as a form of documentation, making it easier for team members to understand the shape of data and how different components interact. This leads to smoother collaboration, as developers can quickly grasp how to use components and APIs correctly without resorting to extensive manual documentation.
4. Setting Up Vue.js with TypeScript
Getting started with Vue.js and TypeScript is a breeze. The Vue team provides official tools and documentation to help you set up a project with these technologies. Here’s a step-by-step guide:
4.1. Vue CLI Installation
First, you need to install Vue CLI, the command-line tool for scaffolding Vue.js projects. Open your terminal and run:
bash npm install -g @vue/cli
4.2. Project Creation
Create a new Vue.js project using Vue CLI:
bash vue create my-vue-ts-app
Replace “my-vue-ts-app” with your preferred project name. During project setup, Vue CLI will ask you to select features and configurations. Choose the default preset or manually select features based on your needs.
4.3. Adding TypeScript
Once the project is created, navigate into your project folder and add TypeScript:
bash cd my-vue-ts-app vue add @vue/typescript
This command installs the necessary TypeScript dependencies and configures your project to work seamlessly with TypeScript.
5. Writing Vue Components with TypeScript
Now that your project is set up, let’s dive into creating Vue components using TypeScript. In the “src/components” folder, you can start by defining a new TypeScript component:
vue <template> <div> <h1>{{ message }}</h1> </div> </template> <script lang="ts"> import { Component, Vue } from 'vue-property-decorator'; @Component export default class MyComponent extends Vue { private message: string = 'Hello from TypeScript!'; } </script>
Notice how we’re using the lang=”ts” attribute in the <script> tag to indicate that the script is written in TypeScript. We’re also using the vue-property-decorator package to enhance our component with TypeScript decorators.
6. Leveraging Type Annotations
TypeScript’s power comes from its type annotations. Let’s say you’re building a simple todo application. You can create an interface for your todo items:
typescript interface Todo { id: number; text: string; completed: boolean; } Then, in your component, you can use this interface to define the type of your todo data: typescript Copy code <template> <div> <ul> <li v-for="todo in todos" :key="todo.id"> {{ todo.text }} </li> </ul> </div> </template> <script lang="ts"> import { Component, Vue } from 'vue-property-decorator'; interface Todo { id: number; text: string; completed: boolean; } @Component export default class TodoList extends Vue { private todos: Todo[] = [ { id: 1, text: 'Buy groceries', completed: false }, { id: 2, text: 'Do laundry', completed: true }, ]; } </script>
Here, by specifying the Todo[] type for the todos array, you’re ensuring that only objects adhering to the Todo interface structure can be added to the array.
7. Handling Events and Props
TypeScript helps you catch potential issues when working with event handlers and props. For example, when emitting an event from a child component, you can specify its type:
typescript import { Component, Vue, Emit } from 'vue-property-decorator'; @Component export default class ChildComponent extends Vue { @Emit() emitCustomEvent(payload: string): string { return payload; } }
And in the parent component, you can receive the emitted event with the correct payload type:
typescript <template> <div> <child-component @customEvent="handleCustomEvent" /> </div> </template> <script lang="ts"> import { Component, Vue } from 'vue-property-decorator'; @Component export default class ParentComponent extends Vue { private handleCustomEvent(payload: string): void { console.log(`Received payload: ${payload}`); } } </script>
8. Integrating Vue Router with TypeScript
Integrating Vue Router, the official routing library for Vue.js, with TypeScript is seamless. First, install Vue Router in your project:
bash npm install vue-router
Next, create a TypeScript definition file (e.g., vue-router.d.ts) in your project’s “src” folder to provide type information for Vue Router:
typescript import Vue from 'vue'; declare module 'vue/types/vue' { interface Vue { $router: VueRouter; $route: Route; } }
With this setup, you can now use Vue Router in your components with TypeScript’s autocompletion and type checking.
Conclusion
Vue.js and TypeScript are individually powerful technologies that bring significant advantages to modern web development. When combined, they create a synergy that not only boosts your productivity but also enhances the reliability and maintainability of your codebase. With TypeScript’s static typing and Vue.js’s intuitive component-based architecture, you’ll find yourself confidently tackling complex projects while minimizing errors and maximizing collaboration. So, if you’re ready to supercharge your web development journey, dive into the world of Vue.js and TypeScript—you’ll be amazed at the transformative impact they can have on your development process.
Remember, learning these technologies might seem like a steep hill at first, but with dedication and practice, you’ll soon find yourself harnessing their full potential to create outstanding web applications. Happy coding!
Table of Contents
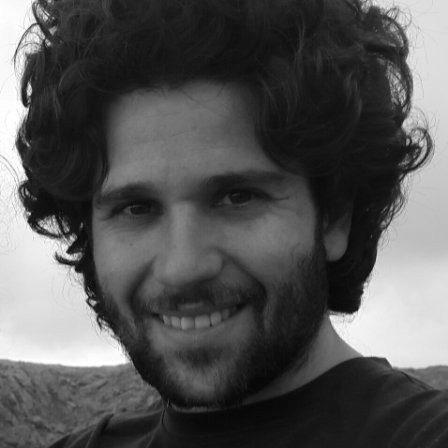
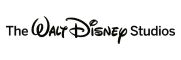