Expanding Horizons: Advanced AI Development with Java – A Deep Dive into Machine Learning Libraries and Frameworks
Artificial Intelligence (AI) and Machine Learning (ML) have rapidly evolved from niche subjects to essential technologies influencing a myriad of industries. From healthcare to finance, AI and ML have become vital tools to drive innovation and efficiency. As such, the demand to hire AI developers has exponentially grown.
Understanding these transformative technologies as a developer and learning to adeptly harness them can significantly impact your contributions in this era of digital revolution. Java, being one of the most globally widespread programming languages, is known for its versatility, robustness, and mature ecosystem. Given these attributes, it comes as no surprise that it has morphed into a favored choice for AI and ML development.
In this blog post, we will delve into AI development with Java, specifically focusing on machine learning applications. Here, we aim to provide insights that can be beneficial to those looking to hire AI developers with a firm grasp of Java, or developers seeking to broaden their skills and venture into AI and ML domain.
Introduction to Machine Learning with Java
Java is a statically-typed, object-oriented language that offers significant benefits for AI and ML development. Not only does Java’s wide array of libraries make it easier to design and implement machine learning models, but its platform independence and scalability also allow developers to deploy these models on virtually any hardware configuration.
Java’s garbage collection and exception handling mechanisms simplify memory management and debugging, enabling developers to focus more on designing and optimizing machine learning models. Moreover, the strong typing in Java allows errors to be caught early in the development process, which can be a significant advantage in complex machine learning applications.
Let’s explore some libraries and frameworks for machine learning in Java.
1. Weka
The Waikato Environment for Knowledge Analysis (Weka) is a Java-based machine learning library. It provides a collection of visualization tools and algorithms for data analysis and modeling through a user-friendly interface. Weka is widely used for teaching, research, and industrial applications, embracing a large number of machine learning algorithms.
Below is an example of how to use Weka for creating a simple classifier.
```java import weka.classifiers.trees.J48; import weka.core.Instance; import weka.core.Instances; import weka.core.converters.ConverterUtils.DataSource; public class WekaExample { public static void main(String[] args) throws Exception { // Load training dataset DataSource source = new DataSource("trainingData.arff"); Instances trainDataset = source.getDataSet(); trainDataset.setClassIndex(trainDataset.numAttributes()-1); // Build a model J48 tree = new J48(); tree.buildClassifier(trainDataset); // Load new dataset DataSource sourceNew = new DataSource("newData.arff"); Instances newDataset = sourceNew.getDataSet(); newDataset.setClassIndex(newDataset.numAttributes()-1); // Classify new instances for (Instance inst : newDataset) { double classLabel = tree.classifyInstance(inst); System.out.println("Instance classified as : " + classLabel); } } } ```
In this example, we’re loading a dataset, training a J48 decision tree classifier, then classifying new instances.
2. Deeplearning4j
Deeplearning4j, or DL4J, is a powerful, open-source, distributed deep learning library for JVM. DL4J is designed to be used in business environments on distributed GPUs and CPUs. It provides a deep learning programming paradigm, allowing you to focus more on the design of models rather than the details of implementation.
Here’s a simple example of using DL4J to create a multilayer perceptron for classification:
```java import org.deeplearning4j.nn.api.OptimizationAlgorithm; import org.deeplearning4j.nn.conf.MultiLayerConfiguration; import org.deeplearning4j.nn.conf.NeuralNetConfiguration; import org.deeplearning4j.nn.conf.layers.DenseLayer; import org.deeplearning4j.nn.conf.layers.OutputLayer; import org.deeplearning4j.nn.weights.WeightInit; import org.nd4j.linalg.activations.Activation; import org.nd4j.linalg.dataset.api.iterator.DataSetIterator; import org.nd4j.linalg.lossfunctions.LossFunctions; public class DL4JExample { public static void main(String[] args) { int seed = 123; int numInputs = 10; int numOutputs = 2; int numHiddenNodes = 20; // Load data DataSetIterator dataIter = new YourDataIteratorImplementation(); // Assumes you have a custom data iterator // Configure network MultiLayerConfiguration conf = new NeuralNetConfiguration.Builder() .seed(seed) .optimizationAlgo(OptimizationAlgorithm.STOCHASTIC_GRADIENT_DESCENT) .list() .layer(0, new DenseLayer.Builder().nIn(numInputs).nOut(numHiddenNodes).weightInit(WeightInit.XAVIER).activation(Activation.RELU).build()) .layer(1, new OutputLayer.Builder(LossFunctions.LossFunction.NEGATIVELOGLIKELIHOOD).weightInit(WeightInit.XAVIER).activation(Activation.SOFTMAX).nIn(numHiddenNodes).nOut(numOutputs).build()) .pretrain(false).backprop(true) .build(); // Train model MultiLayerNetwork model = new MultiLayerNetwork(conf); model.init(); model.fit(dataIter); // Evaluate model DataSetIterator testIter = new YourTestDataIteratorImplementation(); Evaluation eval = new Evaluation(numOutputs); while(testIter.hasNext()){ DataSet t = testIter.next(); INDArray features = t.getFeatureMatrix(); INDArray lables = t.getLabels(); INDArray predicted = model.output(features,false); eval.eval(lables, predicted); } System.out.println(eval.stats()); } } ```
In this example, we create a multilayer perceptron with a single hidden layer and an output layer. We then load data using a custom `DataSetIterator`, fit the model, and evaluate it.
3. MOA (Massive Online Analysis)
MOA is a popular open-source software designed specifically for machine learning and data mining on data streams in real-time. It includes a collection of ML algorithms, including decision trees, rule induction algorithms, clustering, outlier detection, and ensemble methods.
MOA is most beneficial for tasks that need to handle a significant amount of data that cannot fit in main memory, as its environment is designed for developing new algorithms in the field of data stream mining.
Here’s a brief example of how to use MOA to train a decision tree:
```java import com.yahoo.labs.samoa.instances.Instance; import moa.classifiers.trees.HoeffdingTree; import moa.streams.generators.RandomTreeGenerator; public class MoaExample { public static void main(String[] args) { // Generate a stream RandomTreeGenerator stream = new RandomTreeGenerator(); stream.prepareForUse(); // Create a classifier HoeffdingTree tree = new HoeffdingTree(); tree.setModelContext(stream.getHeader()); tree.prepareForUse(); // Train the classifier on 10000 instances int numInstances = 10000; for (int i = 0; i < numInstances; i++) { Instance inst = stream.nextInstance(); tree.trainOnInstance(inst); } // Use the classifier (tree) to make predictions on new data... } } ```
4. Smile (Statistical Machine Intelligence and Learning Engine)
Smile is a comprehensive ML library that provides a range of ML algorithms, natural language processing, linear algebra, graph processing, and visualization methods. It’s optimized for speed and memory usage and is excellent for developing production-grade, performance-critical applications.
Here’s an example of how to use Smile for k-means clustering:
```java import smile.clustering.KMeans; import smile.plot.swing.ScatterPlot; public class SmileExample { public static void main(String[] args) { double[][] data = { // Insert your dataset here {1.0, 2.0}, {1.5, 2.5}, {5.0, 6.0}, {8.0, 9.0} }; // Perform k-means clustering KMeans kmeans = KMeans.fit(data, 2); // Display the cluster results ScatterPlot plot = ScatterPlot.of(data, kmeans.y, '*'); plot.canvas().setAxisLabels("X", "Y"); plot.window(); } } ```
5. DL4J’s Skymind Intelligence Layer (SKIL)
Skymind Intelligence Layer (SKIL) is not just a library; it’s an entire platform for deploying, managing, and monitoring machine learning models. It offers a range of features that make it easier to manage workflows and deploy models at scale. One of its unique features is Zeppelin integration, which allows for interactive data analytics.
SKIL is based on the DL4J suite and supports a range of other libraries, including TensorFlow, Keras, and PyTorch.
Here’s how you can deploy a DL4J model using SKIL:
```java import ai.skymind.skil.Model; import ai.skymind.skil.Service; import ai.skymind.skil.Workspace; import ai.skymind.skil.Deployment; import org.deeplearning4j.nn.multilayer.MultiLayerNetwork; import java.io.File; public class SkilExample { public static void main(String[] args) throws Exception { // Load a DL4J model MultiLayerNetwork network = MultiLayerNetwork.load(new File("myModel.zip"), false); // Deploy the model using SKIL Workspace ws = new Workspace("myWorkspace"); Deployment dep = new Deployment(ws, "myDeployment"); Model model = new Model(network, ws); Service service = new Service(dep, model, "myService"); } } ```
In this example, we load a DL4J model and then deploy it using SKIL. A `Workspace` is a SKIL concept that groups related deployments, while a `Deployment` is a collection of services, and a `Service` wraps a machine learning model and exposes endpoints to interact with the model.
Conclusion
The introduction of powerful libraries and frameworks for machine learning in Java has revolutionized AI development, paving the way for more efficient and effective solutions. By leveraging these resources, Java developers can design, implement, and deploy robust machine learning models, driving innovation and optimizing operations across a multitude of industries. This evolution has opened a new avenue to hire AI developers with expertise in Java, enhancing the quality and capability of AI applications.
Despite the rise of Python as the go-to language for AI and ML, Java maintains its place as a reliable, robust, and versatile alternative, particularly in enterprise settings. With its mature ecosystem and extensive support for parallel computing, Java is superbly equipped to handle the challenges of AI development in the era of big data.
Whether you’re a seasoned Java developer ready to explore the world of machine learning or an AI enthusiast eager to harness the power of Java, the opportunities for AI development with Java are vast and rewarding. This transformation has fueled the demand to hire AI developers who can navigate these new tools and deliver high-quality, efficient, and innovative solutions.
Table of Contents
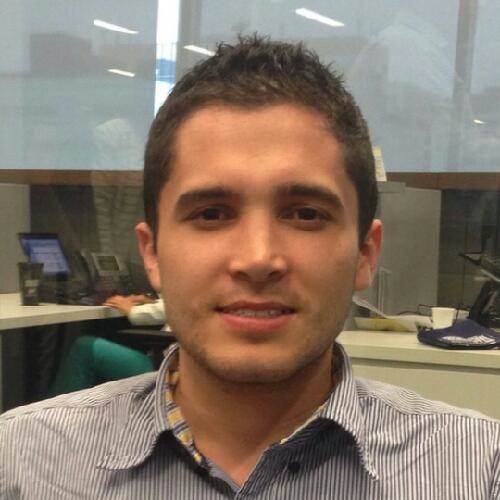
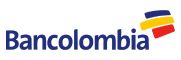