Building AI-powered Virtual Assistants with Natural Language Processing
In today’s fast-paced digital world, virtual assistants have become an integral part of our daily lives. From simple tasks like setting reminders to complex actions like making reservations, virtual assistants have proven to be invaluable tools. But have you ever wondered how these virtual assistants understand and respond to our commands with such accuracy and speed? The answer lies in Natural Language Processing (NLP), a field of artificial intelligence that focuses on the interaction between computers and human language. In this comprehensive guide, we will explore the fascinating world of building AI-powered virtual assistants using NLP techniques, covering everything from basic concepts to practical code samples.
1. Understanding Natural Language Processing
1.1. What is NLP?
Natural Language Processing (NLP) is a subfield of artificial intelligence that focuses on enabling computers to understand, interpret, and generate human language. It involves a combination of linguistics, computer science, and machine learning techniques to bridge the communication gap between humans and machines. NLP algorithms aim to process and analyze text and speech data, enabling machines to derive meaning, context, and intent from human language.
1.2. Why is NLP important for virtual assistants?
Virtual assistants rely on NLP to understand user commands, questions, and requests. Without NLP, interactions with virtual assistants would be limited to rigid, predefined commands, severely hampering their usability and effectiveness. NLP enables virtual assistants to handle a wide range of user inputs, adapt to variations in language, and provide contextually relevant responses. As NLP technology advances, virtual assistants become more capable of understanding nuanced language, resulting in more natural and engaging interactions.
2. Key Components of Virtual Assistants
Virtual assistants leverage several key components to provide seamless interactions:
2.1. Speech Recognition
Speech recognition, also known as Automatic Speech Recognition (ASR), involves converting spoken language into written text. This technology enables virtual assistants to understand and process spoken commands. ASR technology has made significant advancements in recent years, leading to highly accurate transcription of spoken language.
2.2. Intent Recognition
Intent recognition involves identifying the user’s intention behind a given command or query. For instance, if a user says, “What’s the weather like today?”, the intent is to retrieve weather information. Virtual assistants use NLP techniques to categorize user inputs into specific intents, which then guide the assistant’s response.
2.3. Entity Recognition
Entity recognition involves identifying specific pieces of information within user inputs. For example, in the query “Book a table for two at 7 PM,” the entities are “table,” “two,” and “7 PM.” Recognizing entities allows the virtual assistant to extract and utilize relevant information when fulfilling user requests.
2.4. Response Generation
Response generation is the process of crafting appropriate and contextually relevant responses to user inputs. This involves not only providing accurate information but also generating responses that mimic human conversation patterns. NLP models, such as Generative Pre-trained Transformers (GPT), have revolutionized response generation by producing coherent and contextually appropriate text.
3. Getting Started with NLP and Virtual Assistants
3.1. Choosing the Right NLP Framework
Before building a virtual assistant, you need to choose an NLP framework that aligns with your project’s requirements. Some popular NLP frameworks include:
- NLTK (Natural Language Toolkit): A comprehensive library for NLP tasks, particularly useful for beginners.
- spaCy: A fast and efficient NLP library with pre-trained models for various languages.
- Hugging Face Transformers: Known for its powerful pre-trained models like GPT-3, which can be fine-tuned for specific tasks.
3.2. Setting Up Your Development Environment
Once you’ve selected an NLP framework, set up your development environment. This typically involves installing the necessary libraries, downloading pre-trained models, and configuring your code editor or IDE. Most frameworks provide detailed documentation to guide you through the setup process.
4. Building a Simple Virtual Assistant
Let’s start building a basic virtual assistant that can understand and respond to user inputs. For this example, we’ll use Python and the NLTK library.
4.1. Handling Text Input
python import nltk from nltk.tokenize import word_tokenize nltk.download('punkt') def process_text(input_text): tokens = word_tokenize(input_text) return tokens user_input = "What's the weather like today?" tokens = process_text(user_input) print(tokens)
In this code snippet, we tokenize the user’s input text into individual words using NLTK’s word_tokenize function.
4.2. Basic Intent Recognition
python from nltk import FreqDist from nltk.corpus import stopwords nltk.download('stopwords') def recognize_intent(tokens): # Remove stopwords and non-alphabetic tokens filtered_tokens = [word for word in tokens if word.isalpha() and word.lower() not in stopwords.words('english')] # Calculate frequency distribution of tokens freq_dist = FreqDist(filtered_tokens) # Identify the most common token as the intent intent = freq_dist.max() return intent intent = recognize_intent(tokens) print("User intent:", intent)
In this code snippet, we perform basic intent recognition by identifying the most common token in the user’s input as the intent.
4.3. Crafting Meaningful Responses
python def generate_response(intent): responses = { "weather": "The weather today is sunny with a high of 25°C.", "greeting": "Hello! How can I assist you today?", "farewell": "Goodbye! Have a great day!" } response = responses.get(intent, "I'm sorry, I didn't understand that.") return response response = generate_response(intent) print("Virtual assistant:", response)
In this code snippet, we generate responses based on the recognized intent. The virtual assistant provides relevant responses for weather queries, greetings, farewells, and a default response for unrecognized intents.
5. Advanced NLP Techniques for Virtual Assistants
5.1. Sentiment Analysis for User Understanding
Sentiment analysis involves determining the emotional tone of a piece of text. Adding sentiment analysis to your virtual assistant can help it understand user emotions and tailor responses accordingly.
python from nltk.sentiment import SentimentIntensityAnalyzer nltk.download('vader_lexicon') def analyze_sentiment(text): sia = SentimentIntensityAnalyzer() sentiment_scores = sia.polarity_scores(text) sentiment = "positive" if sentiment_scores['compound'] > 0 else "negative" if sentiment_scores['compound'] < 0 else "neutral" return sentiment user_input = "I'm really excited about the upcoming event!" sentiment = analyze_sentiment(user_input) print("User sentiment:", sentiment)
In this code snippet, we use the VADER sentiment analyzer from NLTK to analyze the sentiment of the user’s input.
5.2. Context Maintenance for Multi-turn Conversations
To enable more natural interactions, virtual assistants must maintain context across multiple turns of a conversation. This involves remembering previous user inputs and responses to provide meaningful replies.
python context = [] def respond_to_user(input_text): tokens = process_text(input_text) intent = recognize_intent(tokens) response = generate_response(intent) context.append((input_text, response)) return response user_input1 = "What's the weather like tomorrow?" user_input2 = "Should I bring an umbrella?" response1 = respond_to_user(user_input1) response2 = respond_to_user(user_input2) print("Virtual assistant:", response1) print("Virtual assistant:", response2)
In this code snippet, the virtual assistant maintains a context list to remember user inputs and responses. This allows the assistant to generate contextually relevant responses, even in multi-turn conversations.
6. Code Sample: Building an NLP-powered Intent Classifier
6.1. Using Machine Learning Libraries
For more complex intent recognition, machine learning models can be trained to classify user inputs into specific intents. Here’s a basic example using scikit-learn:
python from sklearn.feature_extraction.text import CountVectorizer from sklearn.naive_bayes import MultinomialNB from sklearn.pipeline import Pipeline from sklearn.metrics import accuracy_score from sklearn.model_selection import train_test_split # Sample intent data data = [ ("What's the weather like today?", "weather"), ("How do I get to the nearest park?", "directions"), ("Tell me a joke!", "entertainment"), # ... more training data ... ] # Split data into features and labels X = [item[0] for item in data] y = [item[1] for item in data] # Split data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Build a pipeline with text vectorization and classifier pipeline = Pipeline([ ('vectorizer', CountVectorizer()), ('classifier', MultinomialNB()) ]) # Train the model pipeline.fit(X_train, y_train) # Predict intents on test data y_pred = pipeline.predict(X_test) # Calculate accuracy accuracy = accuracy_score(y_test, y_pred) print("Intent classification accuracy:", accuracy)
In this code snippet, we use scikit-learn to build a simple intent classifier using the Multinomial Naive Bayes algorithm.
7. Deploying Your Virtual Assistant
Deploying an AI-powered virtual assistant involves considerations for scalability, security, and accessibility. Cloud platforms like AWS, Azure, and Google Cloud provide services for hosting and scaling AI applications.
7.1. Cloud Hosting Considerations
When deploying your virtual assistant, consider the following:
- Scalability: Ensure your deployment can handle varying levels of user interactions without performance issues.
- Security: Implement encryption, access controls, and regular security audits to protect user data and maintain privacy.
- Latency: Optimize your deployment for low-latency responses, especially for real-time interactions.
8. The Future of AI-powered Virtual Assistants
AI-powered virtual assistants continue to evolve, with advancements in both technology and user expectations.
8.1. Voice Assistants and Beyond
Voice assistants, like Amazon’s Alexa and Google Assistant, have gained widespread adoption, allowing users to interact with technology using natural speech. As voice recognition and synthesis technologies improve, voice assistants will become even more integrated into daily life.
8.2. Ethical Considerations and User Privacy
As virtual assistants become more capable, ethical considerations become increasingly important. Developers must prioritize user privacy, data security, and transparency. Striking a balance between convenience and privacy will be crucial to building trust with users.
Conclusion
In conclusion, building AI-powered virtual assistants with NLP opens up a world of possibilities for enhancing user experiences. By understanding NLP concepts, leveraging key components, and implementing advanced techniques, you can create virtual assistants that provide valuable and engaging interactions in various domains.
Remember, the journey of building virtual assistants is an ongoing one, as technology continues to advance and user expectations evolve. Stay curious, keep learning, and be at the forefront of shaping the future of AI-powered interactions.
By following this comprehensive guide, you’ve gained insights into the world of AI-powered virtual assistants, Natural Language Processing, and the key components that make these assistants so effective. Whether you’re just starting out or looking to enhance your existing virtual assistant projects, the concepts, techniques, and code samples covered here will serve as a solid foundation for creating intelligent and user-friendly virtual assistants. Happy building!
Table of Contents
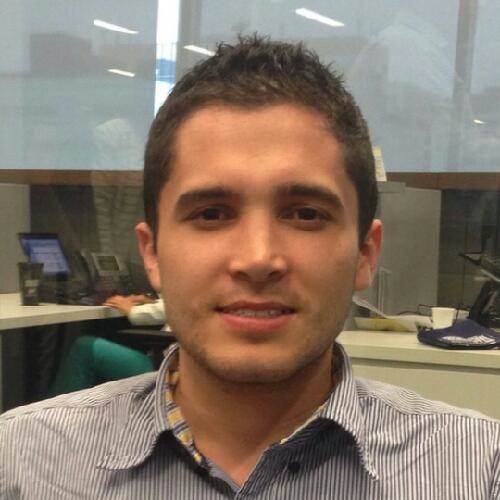
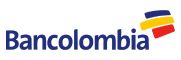