AI Development and Cybersecurity: Fortifying Digital Defenses
In the rapidly evolving landscape of cybersecurity, artificial intelligence (AI) has emerged as a pivotal tool for fortifying digital defenses. AI can analyze vast amounts of data, detect anomalies, and respond to threats with remarkable efficiency. This article explores how AI can be integrated into cybersecurity strategies and provides practical examples of how it can be utilized to enhance security.
Understanding AI in Cybersecurity
AI in cybersecurity involves using machine learning algorithms, natural language processing, and other AI technologies to improve threat detection, response, and prevention. By analyzing patterns and anomalies in data, AI can help organizations proactively address security threats.
Using AI for Cyber Threat Detection
AI excels at identifying patterns and anomalies that might indicate a security threat. Machine learning models can be trained to recognize malicious activities and adapt to new threats over time. Below are key aspects and code examples demonstrating how AI can be used for threat detection.
1. Anomaly Detection
Anomaly detection algorithms can identify unusual patterns in network traffic or system behavior, which may indicate a potential security threat.
Example: Anomaly Detection with Isolation Forest
Here’s how you might use an Isolation Forest algorithm to detect anomalies in network traffic data.
```python from sklearn.ensemble import IsolationForest import numpy as np # Sample data: network traffic metrics data = np.array([ [0.1, 0.2], [0.2, 0.3], [0.4, 0.5], [10.0, 10.0] # Anomalous data point ]) # Create and train the model model = IsolationForest(contamination=0.1) model.fit(data) # Predict anomalies predictions = model.predict(data) print("Predictions:", predictions) ```
2. Threat Intelligence Integration
AI can be used to aggregate and analyze data from various threat intelligence sources, providing a comprehensive view of potential threats.
Example: Integrating Threat Intelligence with Natural Language Processing
Here’s a basic example of using NLP to analyze threat intelligence reports.
```python from textblob import TextBlob # Sample threat report text text = "A new malware strain is spreading rapidly across systems." # Perform sentiment analysis blob = TextBlob(text) sentiment = blob.sentiment print(f"Sentiment: {sentiment}") ```
3. Automated Response Systems
AI can help automate responses to detected threats, reducing response times and mitigating damage.
Example: Automating Response with a Simple Rule-Based System
Here’s a basic example of how you might use a rule-based system to automate responses to detected threats.
```python def respond_to_threat(threat_type): responses = { "malware": "Quarantine affected systems.", "phishing": "Alert users and block phishing emails.", "anomaly": "Investigate unusual activity." } return responses.get(threat_type, "Unknown threat type.") # Example usage threat_type = "malware" response = respond_to_threat(threat_type) print("Response:", response) ```
Enhancing Security with AI-Driven Analytics
AI-driven analytics can provide deeper insights into security data, helping organizations to understand trends and predict future threats.
Example: Using AI for Predictive Analytics
Here’s an example of using AI for predictive analytics to forecast potential threats.
```python from sklearn.linear_model import LinearRegression import numpy as np # Sample data: past threat occurrences X = np.array([[1], [2], [3], [4], [5]]) # Time (e.g., days) y = np.array([1, 2, 3, 4, 5]) # Threat count # Create and train the model model = LinearRegression() model.fit(X, y) # Predict future threats future_time = np.array([[6]]) predicted_threats = model.predict(future_time) print("Predicted Threats:", predicted_threats) ```
Conclusion
AI offers powerful capabilities for enhancing cybersecurity by improving threat detection, response, and analytics. By leveraging AI technologies, organizations can develop more robust defenses against evolving cyber threats. Integrating AI into your cybersecurity strategy will help you stay ahead of potential threats and fortify your digital defenses.
Further Reading
Table of Contents
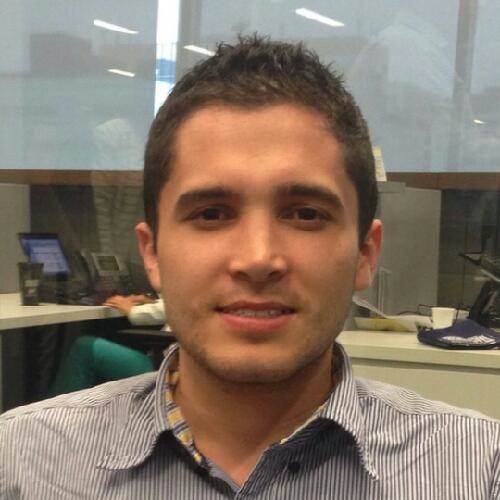
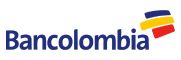