Exploring Deep Learning: Frameworks for AI Development
In the realm of Artificial Intelligence (AI), deep learning has emerged as a dominant force, revolutionizing various industries with its remarkable capabilities. From image recognition to natural language processing, deep learning has made groundbreaking advancements in solving complex problems. Behind this success lies the power of deep learning frameworks, which serve as the backbone for developing and deploying AI models.
In this blog, we’ll delve into the world of deep learning frameworks, exploring their significance, and discussing some of the popular frameworks used for AI development. Through code samples and demonstrations, we’ll demonstrate how these frameworks enable researchers and developers to create cutting-edge AI solutions.
1. Understanding the Role of Deep Learning Frameworks:
Before we dive into the specifics, it’s essential to understand why deep learning frameworks are vital for AI development. These frameworks provide a high-level abstraction, making it easier for developers to construct complex neural networks without having to delve into low-level implementation details. Additionally, they facilitate seamless GPU acceleration, optimizing the computation process and accelerating training times.
2. TensorFlow: Empowering Large-Scale AI Deployments:
2.1 Introduction to TensorFlow
TensorFlow, developed by the Google Brain team, is one of the most widely used deep learning frameworks today. It offers a flexible architecture that allows developers to build and deploy AI models efficiently.
2.2 Creating a Neural Network with TensorFlow
python import tensorflow as tf # Define a sequential model model = tf.keras.Sequential([ tf.keras.layers.Dense(64, activation='relu', input_shape=(input_dim,)), tf.keras.layers.Dense(10, activation='softmax') ]) # Compile the model model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
2.3 Leveraging TensorFlow’s Ecosystem
TensorFlow’s ecosystem extends beyond its core framework, providing additional libraries like TensorFlow Serving for deployment, TensorFlow Lite for mobile and edge devices, and TensorFlow Extended (TFX) for end-to-end ML pipelines.
3. PyTorch: Dynamic Computation for Research-Oriented Projects:
3.1 Introduction to PyTorch
PyTorch is an open-source deep learning framework that has gained immense popularity among researchers due to its dynamic computation graph. This feature enables flexible model building and makes it well-suited for research-oriented projects.
3.2 Building a Convolutional Neural Network with PyTorch
python import torch import torch.nn as nn import torch.optim as optim class CNN(nn.Module): def __init__(self): super(CNN, self).__init__() self.conv1 = nn.Conv2d(in_channels=1, out_channels=16, kernel_size=3) self.conv2 = nn.Conv2d(in_channels=16, out_channels=32, kernel_size=3) self.fc = nn.Linear(in_features=32*5*5, out_features=10) def forward(self, x): x = F.relu(self.conv1(x)) x = F.relu(self.conv2(x)) x = F.avg_pool2d(x, 2) x = x.view(-1, 32*5*5) x = self.fc(x) return x # Create the model and define the loss function and optimizer model = CNN() criterion = nn.CrossEntropyLoss() optimizer = optim.Adam(model.parameters(), lr=0.001)
3.3 TorchScript and Production Deployment
PyTorch offers TorchScript, a way to convert PyTorch models into a deployable format with the TorchScript JIT compiler. This enables seamless integration of PyTorch models into production environments.
4. High-Level Abstraction for Quick Prototyping:
4.1 Introduction to Keras
Keras, an open-source neural network API written in Python, provides an easy-to-use interface for building and experimenting with deep learning models. It is designed to be user-friendly and allows for rapid prototyping.
4.2 Designing a Recurrent Neural Network with Keras
python from keras.models import Sequential from keras.layers import Embedding, LSTM, Dense model = Sequential() model.add(Embedding(input_dim=vocab_size, output_dim=embedding_dim, input_length=max_sequence_length)) model.add(LSTM(64)) model.add(Dense(num_classes, activation='softmax')) model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
4.3 TensorFlow as the Backend for Keras
Keras can use TensorFlow as its backend, providing users the best of both worlds: Keras’s simplicity and TensorFlow’s extensive features.
5. MXNet: Scalability and Efficiency Combined:
5.1 Introduction to MXNet
MXNet is a deep learning framework known for its scalability and efficiency. It supports both imperative and symbolic programming, making it suitable for a wide range of applications.
5.2 Symbolic API in MXNet
MXNet’s symbolic API allows developers to build and optimize complex neural network architectures with ease.
python import mxnet as mx # Define a symbolic computation graph data = mx.symbol.Variable('data') fc = mx.symbol.FullyConnected(data=data, num_hidden=128) act = mx.symbol.Activation(data=fc, act_type='relu') output = mx.symbol.SoftmaxOutput(data=act, name='softmax')
5.3 Gluon API: Imperative Programming in MXNet
MXNet also provides Gluon, an imperative programming API that offers a more flexible and intuitive way to build models.
6. Choosing the Right Framework for Your AI Projects:
6.1 Factors to Consider
When selecting a deep learning framework, several factors come into play, including ease of use, community support, performance, and compatibility with your existing infrastructure.
6.2 Frameworks in Research vs. Production
The choice of framework may also vary depending on whether your project is research-oriented or aimed at production deployment.
Conclusion:
Deep learning frameworks are the backbone of modern AI development, empowering researchers and developers to create powerful and innovative AI solutions. In this blog, we explored some of the most popular frameworks, including TensorFlow, PyTorch, Keras, and MXNet, along with code samples showcasing their capabilities. As the field of AI continues to evolve, these frameworks will remain crucial tools for driving AI innovation and pushing the boundaries of what’s possible.
Table of Contents
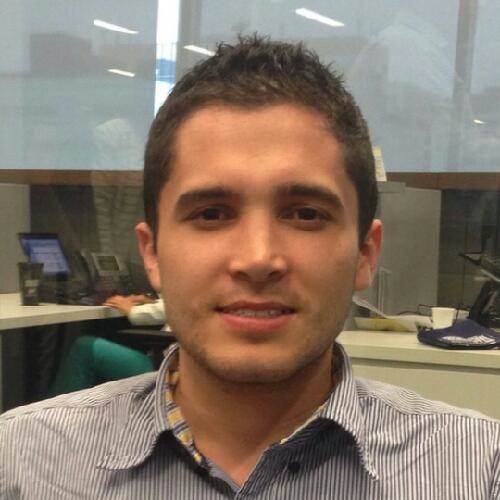
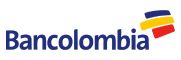