Efficient AI Modeling: A Comprehensive Guide to Mastering AI Development with C++
Artificial Intelligence (AI) continues to be a transformative force across various industries, spearheading groundbreaking advancements in sectors such as healthcare, finance, and automation. As AI becomes increasingly prevalent, many organizations are choosing to hire AI developers to leverage this powerful technology.
While AI has become more accessible due to the widespread proliferation of high-level programming languages like Python, for efficiency and performance-critical applications, the power of C++ is indispensable. This is an important factor to consider when you aim to hire AI developers for your projects.
This blog post will introduce you to the fascinating world of AI development using C++, from basic concepts to advanced techniques. It’s not only a valuable resource for those who aspire to become proficient in AI development with C++, but also for organizations looking to hire AI developers with the ability to develop high-performance AI models. We’ll take a deep dive into how to harness the robustness of C++ to bring the utmost efficiency to your AI applications.
Why C++ for AI?
C++ is widely appreciated for its high performance and control over system resources. This is especially useful in AI applications where large volumes of data must be processed quickly and efficiently. It’s not uncommon for the runtime of an AI model developed in Python to significantly decrease when re-implemented in C++.
Getting Started: Basics of AI with C++
If you’re just starting out in the field of AI and chose C++ as your go-to language, it’s essential to have a solid grasp of fundamental concepts. Here’s how to get started:
Choosing the Right Libraries
There are several open-source libraries that greatly simplify the development of AI in C++. These include libraries such as:
– MLPack: A machine learning library that provides a range of pre-implemented algorithms.
– Shark: This provides algorithms for linear and non-linear optimization, kernel-based learning algorithms, neural networks, and other machine learning algorithms.
– Dlib: A versatile library with a C++ API. It provides machine learning algorithms and tools for creating complex software in C++ to solve real-world problems.
A Simple Example
For starters, let’s understand how we can implement a simple linear regression model in C++ using the `dlib` library. We will try to fit a line to the given data points.
First, include the necessary headers.
```cpp #include <iostream> #include <dlib/svm.h> ```
Next, define your input matrix and target vector.
```cpp dlib::matrix<double> X(5,1); dlib::matrix<double> Y(5,1); X = 1, 2, 3, 4, 5; Y = 2, 3, 4, 5, 6; ```
Now, you can train your model and predict.
```cpp dlib::linear_regression_function< dlib::matrix<double,0,1> > linReg; linReg.train(X, Y); double prediction = linReg(dlib::matrix<double>(1,1,6)); ```
This simple example demonstrates how to train a linear regression model using C++. Of course, real-world applications will be more complex, but this serves as a good starting point.
Taking it to the Next Level: Advanced AI with C++
When you are ready to dive into more advanced AI applications, deep learning is the next natural step. Deep learning is a subset of machine learning where artificial neural networks, algorithms inspired by the human brain, learn from large amounts of data.
To effectively leverage these advanced AI capabilities, you might find it beneficial to hire AI developers with experience in these areas. With their knowledge and expertise, they can navigate the complex layers of deep learning applications, designing solutions that can process and learn from vast amounts of data.
In essence, when you hire AI developers with deep learning expertise, you’re investing in the future of your organization, enabling it to stay competitive in a world increasingly driven by AI innovations.
Advanced Libraries
For advanced applications, such as deep learning, there are powerful libraries available such as:
– Caffe: It’s a deep learning framework developed by the Berkeley Vision and Learning Center (BVLC). It’s perfect for implementing convolutional neural networks and other deep learning models.
– TensorFlow: TensorFlow is an open-source software library developed by Google. It’s primarily used for machine learning and neural network training, but it also includes a C++ API.
– Tiny-dnn: A header-only, dependency-free deep learning framework in C++14. It’s suitable for developing deep learning algorithms for resource-constrained edge devices.
Implementing a Neural Network
As an example, let’s create a simple feed-forward neural network with TensorFlow’s C++ API. Suppose we are trying to classify images of hand-written digits.
Include the necessary headers:
```cpp #include <tensorflow/core/public/session.h> #include <tensorflow/core/platform/env.h> ```
First, you need to restore a pre-trained model:
```cpp std::unique_ptr<tensorflow::Session> session; tensorflow::Status status = tensorflow::NewSession(tensorflow::SessionOptions(), &session); if (!status.ok()) { std::cerr << status.ToString() << "\n"; return 1; } tensorflow::GraphDef graph_def; status = ReadBinaryProto(tensorflow::Env::Default(), "/path/to/model", &graph_def); if (!status.ok()) { std::cerr << status.ToString() << "\n"; return 1; } status = session->Create(graph_def); if (!status.ok()) { std::cerr << status.ToString() << "\n"; return 1; } ```
To classify an image, you will need to feed it into your model:
```cpp tensorflow::Tensor input_tensor(tensorflow::DT_FLOAT, tensorflow::TensorShape({1, img_height, img_width, num_channels})); auto tensor_mapped = input_tensor.tensor<float, 4>(); // populate tensor_mapped with the pixel values of your image std::vector<std::pair<std::string, tensorflow::Tensor>> inputs = { {"input", input_tensor}, }; std::vector<tensorflow::Tensor> outputs; status = session->Run(inputs, {"output"}, {}, &outputs); if (!status.ok()) { std::cerr << status.ToString() << "\n"; return 1; } auto output = outputs[0].tensor<float, 2>(); int predicted_class = std::distance(output.data(), std::max_element(output.data(), output.data() + num_classes)); ```
Conclusion
AI development with C++ presents a captivating journey that blends the power and efficiency of C++ with the intelligence of AI models. Whether you’re just starting out or looking to delve into advanced concepts like deep learning, C++ has the necessary tools and libraries to help you achieve your objectives.
If you’re looking to hire AI developers, consider those with proficiency in C++. This language, while more complex than some high-level languages, offers efficiency and control over hardware, making it an invaluable skill for any AI engineer. Investing in learning C++ is well worth the effort, as it equips developers with the ability to create AI models that not only work effectively but also perform exceptionally well.
When you hire AI developers with a mastery of C++, you ensure the development of high-performance, efficient AI applications that meet your business needs and drive innovation.
Table of Contents
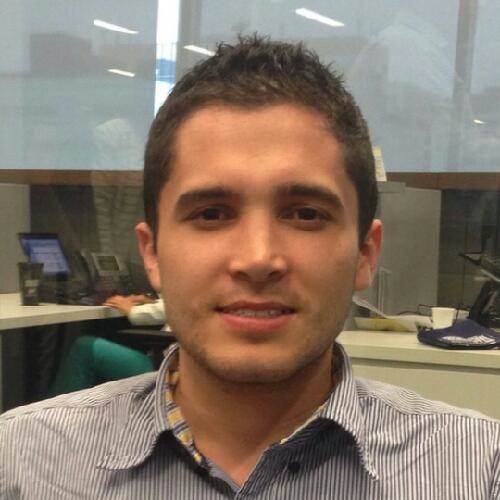
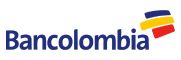