Using PHP for AI Development: A Practical Approach
Artificial Intelligence (AI) has become a driving force behind many modern applications, enhancing user experiences, automating tasks, and providing valuable insights. While languages like Python are commonly associated with AI development, PHP, a server-side scripting language primarily used for web development, can also be harnessed to create AI-powered applications. In this blog, we’ll delve into the intriguing world of using PHP for AI development and explore practical approaches, use cases, implementation strategies, and even provide code samples to get you started on your AI journey.
1. Introduction to PHP and AI
1.1. Understanding PHP and its Applications
PHP, originally designed for web development, has been a staple in creating dynamic and interactive websites. Its simplicity, ease of use, and vast community support have contributed to its popularity. However, AI’s rapid growth has led developers to explore the potential of combining PHP with AI techniques to create intelligent applications that can process, analyze, and generate insights from data.
1.2. The Growing Intersection of PHP and AI
While languages like Python and R are dominant players in AI development due to their extensive libraries and frameworks, PHP’s role should not be underestimated. PHP has evolved beyond web development, with libraries like PHP-ML and PHPSA providing machine learning capabilities. This intersection of web development and AI allows developers to build end-to-end applications that incorporate AI features.
2. Practical Use Cases
2.1. Sentiment Analysis for Customer Feedback
Consider an e-commerce platform that wants to gauge customer sentiments towards products. PHP can be employed to collect and preprocess customer reviews, while AI models can perform sentiment analysis to determine whether reviews are positive, negative, or neutral. This information can be used to improve product offerings and customer satisfaction.
2.2. AI-Driven Image Recognition for E-commerce
In the realm of e-commerce, image recognition plays a pivotal role. With PHP, developers can create a user-friendly interface to upload product images, and AI algorithms can analyze these images for object recognition, enabling automatic tagging and classification of products. This enhances search functionalities and provides a more immersive shopping experience.
2.3. Personalized Recommendations using AI
PHP’s capability to interact with databases and process user data makes it an ideal candidate for building recommendation systems. By integrating AI algorithms, PHP-powered applications can analyze user behavior, preferences, and purchase history to generate personalized product recommendations, increasing cross-selling and user engagement.
3. Getting Started with PHP and AI
3.1. Setting Up Your Development Environment
To begin your journey into PHP-powered AI development, ensure you have PHP installed on your machine. You can easily set up a development environment using tools like XAMPP or WampServer. These packages include Apache, MySQL, and PHP, creating a local server environment for testing and development.
3.2. Integrating AI Libraries and Tools
PHP-ML, a machine learning library for PHP, is a valuable resource for AI development. It provides various algorithms for tasks such as classification, regression, clustering, and more. By integrating PHP-ML into your project, you can leverage its capabilities to build and train AI models within your PHP application.
4. Implementing AI in PHP: Step by Step
4.1. Loading and Preprocessing Data
Data preparation is a critical step in AI development. PHP’s data manipulation abilities can be utilized to load, clean, and preprocess datasets. For instance, if you’re building a sentiment analysis model, you can use PHP to read customer reviews from a database, tokenize the text, and remove stopwords.
php // Sample PHP code for loading and preprocessing data $reviews = // Fetch reviews from database $cleanedReviews = []; foreach ($reviews as $review) { $tokens = tokenizeAndClean($review); $cleanedReviews[] = $tokens; }
4.2. Building and Training AI Models
PHP-ML simplifies the process of building and training AI models. Whether you’re developing a decision tree classifier or a neural network, PHP-ML provides intuitive APIs. Below is an example of training a basic decision tree classifier for sentiment analysis:
php // Sample PHP code for building and training an AI model use Phpml\Classification\DecisionTree; use Phpml\ModelManager; $classifier = new DecisionTree(); $classifier->train($cleanedReviews, $sentiments); // $sentiments: corresponding labels // Save the trained model $modelManager = new ModelManager(); $modelManager->saveToFile($classifier, 'sentiment_model.php');
4.3. Making Predictions and Inferences
Once your model is trained, you can use it to make predictions and inferences on new data. PHP’s integration with AI libraries enables seamless prediction capabilities within your application:
php // Sample PHP code for making predictions using the trained model $newReview = // Get a new review $newTokens = tokenizeAndClean($newReview); $predictedSentiment = $classifier->predict($newTokens); echo "Predicted sentiment: $predictedSentiment";
5. Code Samples and Examples
Code Sample 1: Sentiment Analysis with PHP and AI
php // Sample PHP code for sentiment analysis $review = "This product exceeded my expectations. Highly recommended!"; $tokens = tokenizeAndClean($review); $predictedSentiment = $classifier->predict($tokens); echo "Predicted sentiment: $predictedSentiment";
Code Sample 2: Image Recognition Using PHP and AI
php // Sample PHP code for image recognition $imagePath = "path/to/image.jpg"; $encodedImage = base64_encode(file_get_contents($imagePath)); // Send image data to AI model for recognition $recognizedObject = recognizeImage($encodedImage); echo "Recognized object: $recognizedObject";
6. Challenges and Best Practices
6.1. Handling Performance Considerations
AI development can be computationally intensive. PHP might not be as performant as languages like Python for certain AI tasks. Consider optimizing critical components by leveraging PHP extensions or integrating PHP with other languages if needed.
6.2. Ensuring Data Privacy and Security
AI applications often involve sensitive data. It’s essential to implement robust security measures to protect user data and ensure compliance with data protection regulations. Apply encryption, authentication, and authorization mechanisms as necessary.
7. Future Prospects and Innovations
7.1. The Evolving Role of PHP in AI
As AI continues to evolve, PHP’s role in AI development is likely to expand further. The PHP community might create more AI-focused libraries and tools, making it easier for developers to build sophisticated AI applications without switching languages.
7.2. Emerging Trends in AI Development with PHP
With the rise of edge computing and the Internet of Things (IoT), AI applications are increasingly being deployed on resource-constrained devices. PHP’s lightweight nature makes it a candidate for driving AI-powered IoT applications.
Conclusion
Integrating AI capabilities into PHP development opens up new possibilities for creating intelligent, data-driven applications. From sentiment analysis to image recognition, PHP’s synergy with AI libraries empowers developers to innovate in various domains. By following best practices and staying updated on emerging trends, developers can harness the power of PHP for AI and drive the next wave of AI-powered applications. So, whether you’re a seasoned PHP developer or just beginning, dive into the world of PHP-powered AI and witness the transformative impact it can have on your projects.
Table of Contents
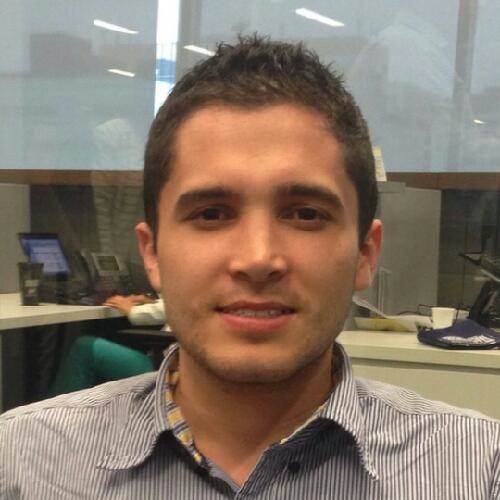
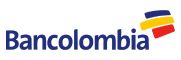