Interview Guide: Hire AI Developers
Artificial Intelligence (AI) has revolutionized various industries, and the demand for skilled AI developers is on the rise. When hiring AI developers, it’s essential to assess their technical expertise, problem-solving abilities, and experience in AI technologies to find the perfect fit for your AI projects. This comprehensive interview guide will help you identify top AI talent and build a strong AI development team.
Table of Contents
1. Top Skills of AI Developers to Look Out For:
Before diving into the interview process, let’s highlight the key skills you should look for in AI developers:
- Strong Programming Skills: AI developers should be proficient in programming languages like Python, R, or Java.
- Machine Learning Algorithms: Familiarity with a wide range of machine learning algorithms for tasks like classification, regression, and clustering.
- Deep Learning Frameworks: Experience with popular deep learning libraries like TensorFlow or PyTorch for building neural networks.
- Data Preprocessing: Knowledge of data preprocessing techniques and feature engineering for effective AI model training.
- Model Evaluation and Optimization: Understanding of evaluation metrics and techniques to optimize AI models’ performance.
- AI Ethics and Bias: Awareness of ethical considerations and bias mitigation in AI model development.
2. Interview Questions and Sample Answers:
Q1. Can you explain the difference between supervised and unsupervised learning in AI? Provide examples of each.
Sample Answer:
Supervised learning involves training a model with labeled data, where the input and corresponding output are provided. For example, image classification is a supervised learning task where images are labeled with corresponding object classes. Unsupervised learning, on the other hand, deals with unlabelled data and seeks to find patterns or clusters within the data. Clustering customer segments in a retail dataset is an example of unsupervised learning.
Q2. How do you handle imbalanced datasets in machine learning projects?
Sample Answer:
Handling imbalanced datasets is crucial to prevent biased model performance. Techniques like oversampling, undersampling, and using algorithms like SMOTE (Synthetic Minority Over-sampling Technique) can help balance the dataset. Additionally, using appropriate evaluation metrics like precision-recall instead of accuracy is essential when dealing with imbalanced data.
Q3. Explain the concept of gradient descent and how it is used to optimize machine learning models.
Sample Answer:
Gradient descent is an optimization algorithm used to minimize the loss function during model training. It calculates the gradient (slope) of the loss function with respect to the model parameters and adjusts the parameters in the opposite direction of the gradient to reach the minimum loss. This process is repeated iteratively until convergence, resulting in optimal model parameters.
Q4. How do you build and train a neural network using TensorFlow?
Sample Answer:
In TensorFlow, we use the ‘tf.keras’ API to build neural networks. Here’s an example of creating a simple feedforward neural network for binary classification:
import tensorflow as tf model = tf.keras.Sequential([ tf.keras.layers.Dense(64, activation='relu', input_shape=(input_dim,)), tf.keras.layers.Dense(32, activation='relu'), tf.keras.layers.Dense(1, activation='sigmoid') ]) model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) model.fit(X_train, y_train, epochs=10, batch_size=32)
Q5. What is transfer learning in deep learning, and how is it beneficial for AI projects?
Sample Answer:
Transfer learning is a technique where a pre-trained neural network is used as a starting point for a new task. By leveraging knowledge learned from a large dataset in a related domain, transfer learning can significantly reduce training time and data requirements for the new task. It allows developers to build powerful AI models even with limited data.
Q6. How do you prevent overfitting in machine learning models?
Sample Answer:
Overfitting occurs when a model performs well on the training data but poorly on unseen data. To prevent overfitting, I use techniques like cross-validation, regularization (L1 and L2), and dropout layers in neural networks. These methods help generalize the model and reduce overfitting by penalizing complex model architectures.
Q7. Explain the concept of natural language processing (NLP) and its applications in AI.
Sample Answer:
Natural Language Processing (NLP) involves the interaction between computers and human language. It enables machines to understand, interpret, and generate human language. NLP finds applications in sentiment analysis, chatbots, machine translation, and text summarization, among others.
Q8. Explain the concept of backpropagation in neural networks and how it enables model training.
Sample Answer:
Backpropagation is an optimization algorithm used to train neural networks. It calculates the gradients of the loss function with respect to the model’s parameters and updates the parameters in the opposite direction of the gradients to minimize the loss. This process is iteratively repeated during training to optimize the model for better performance.
Q9. What is the purpose of activation functions in neural networks? Provide examples of common activation functions.
Sample Answer:
Activation functions introduce non-linearity to neural networks, allowing them to learn complex patterns and relationships in the data. Examples of activation functions include:
-
- ReLU (Rectified Linear Activation)
- Sigmoid
- Tanh (Hyperbolic Tangent)
- Leaky ReLU
Q10. Explain the concept of the bias-variance tradeoff in machine learning and how it impacts model performance.
Sample Answer:
The bias-variance tradeoff refers to the tradeoff between a model’s bias and variance. A high-bias model oversimplifies the data and leads to underfitting, while a high-variance model fits the training data too closely and leads to overfitting. Achieving an optimal balance between bias and variance is essential to build a model that generalizes well to unseen data.
Q11. How do you evaluate the performance of a regression model in AI? Provide examples of evaluation metrics.
Sample Answer:
For evaluating regression models, common metrics include Mean Squared Error (MSE), Root Mean Squared Error (RMSE), and Mean Absolute Error (MAE). These metrics measure the difference between predicted and actual values, indicating how well the model performs in estimating continuous target variables.
Q12. Explain the concept of transfer learning and how it can be applied in computer vision tasks.
Sample Answer:
Transfer learning involves using pre-trained models as a starting point for a new task. In computer vision, this means using a pre-trained model trained on a large dataset like ImageNet and fine-tuning it on a smaller dataset specific to the new task. Transfer learning can save time and resources, especially when training large convolutional neural networks.
Q13. How do you implement early stopping in model training to prevent overfitting?
Sample Answer:
Early stopping is a technique used to prevent overfitting during model training. It involves monitoring the model’s performance on a validation dataset during training. If the validation performance starts to degrade, training is stopped early to avoid overfitting. Implementing early stopping in Keras can be achieved using the ‘EarlyStopping’ callback.
from tensorflow.keras.callbacks import EarlyStopping early_stopping = EarlyStopping(monitor='val_loss', patience=5, restore_best_weights=True) model.fit(X_train, y_train, validation_data=(X_val, y_val), callbacks=[early_stopping])
Q14. Explain the concept of word embeddings in natural language processing (NLP) and their significance.
Sample Answer:
Word embeddings are dense vector representations of words that capture semantic relationships between words. They map words to a continuous vector space, where similar words are closer to each other. Word embeddings improve NLP models’ performance by encoding contextual information, making them more effective in tasks like word similarity and sentiment analysis.
Q15. How do you implement cross-validation in machine learning to evaluate model performance?
Sample Answer:
Cross-validation is a technique used to assess a model’s performance on different subsets of data. For example, in k-fold cross-validation, the dataset is divided into k subsets (folds). The model is trained and evaluated k times, each time using a different fold as the validation set. The final performance is the average of all k evaluations. Implementing cross-validation in scikit-learn can be done using the ‘cross_val_score’ function.
from sklearn.model_selection import cross_val_score from sklearn.model_selection import KFold from sklearn.linear_model import LogisticRegression model = LogisticRegression() kfold = KFold(n_splits=5, shuffle=True, random_state=42) scores = cross_val_score(model, X, y, cv=kfold) print('Cross-validation scores:', scores)
Q16. Explain the concept of backpropagation in neural networks and how it enables model training.
Sample Answer:
Backpropagation is an optimization algorithm used to train neural networks. It calculates the gradients of the loss function with respect to the model’s parameters and updates the parameters in the opposite direction of the gradients to minimize the loss. This process is iteratively repeated during training to optimize the model for better performance.
Q17. How do you interpret the confusion matrix in classification tasks, and what insights does it provide?
Sample Answer:
A confusion matrix is a table used to evaluate the performance of a classification model. It provides insights into the model’s true positive, true negative, false positive, and false negative predictions. From the confusion matrix, we can calculate metrics like precision, recall, F1-score, and accuracy, which help assess the model’s ability to correctly classify different classes.
These technical questions and coding samples will help you assess AI developer skills and their proficiency in key AI concepts and techniques. By evaluating their responses and practical knowledge, you can identify top AI talent who can contribute to your organization’s AI projects and drive innovation.
3. The Importance of the Interview Process in Hiring AI Developers
The interview process plays a vital role in identifying AI developers who possess the technical skills and problem-solving abilities required for complex AI projects. Assessing candidates’ knowledge of AI algorithms, and programming languages, and their ability to apply AI techniques to real-world scenarios ensures that you hire AI developers who can deliver cutting-edge solutions.
4. How CloudDevs Can Help You Find the Right AI Developers
The process of hiring AI developers can be time-consuming and challenging. However, partnering with CloudDevs can simplify the process and help you find top AI talent efficiently.
CloudDevs offers a curated pool of pre-screened senior AI developers with expertise in machine learning, deep learning, and natural language processing. By collaborating with CloudDevs, you gain access to a talent pool that has already been vetted for technical proficiency and problem-solving capabilities. This saves you time and effort, allowing you to focus on interviewing candidates who are the best fit for your AI projects.
In conclusion, the interview process is a critical step in hiring AI developers who can contribute to your organization’s AI initiatives. By evaluating technical skills, knowledge of AI algorithms, and practical experience, you can build a strong AI development team capable of driving innovation and solving complex business challenges. Partnering with CloudDevs ensures that you find and hire top-tier AI developers who will accelerate your AI projects’ success and propel your organization’s growth.
Table of Contents
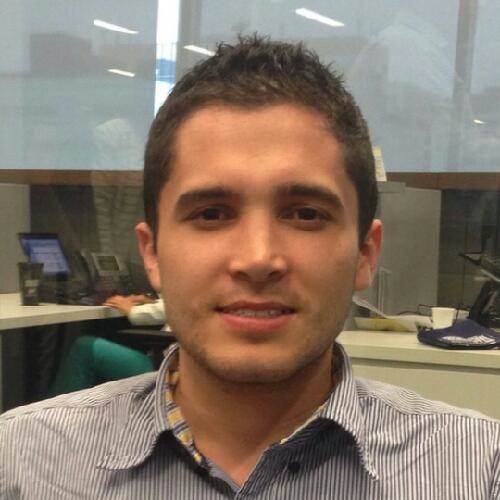
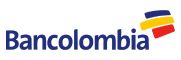