The Evolution of AI: From Machine Learning to Deep Learning
Artificial Intelligence (AI) has emerged as one of the most revolutionary technologies of the modern era, transforming industries, enhancing decision-making processes, and reshaping the way we interact with technology. Central to this transformation is the evolution of AI itself, which can be traced through its key phases: from traditional machine learning to the groundbreaking realm of deep learning. In this blog, we embark on a journey through time to explore how AI has evolved, the role of machine learning, and the advent of deep learning, complete with code samples that illustrate these concepts.
1. Introduction: The Genesis of AI and Machine Learning
Artificial Intelligence, the concept of enabling machines to mimic human-like cognitive functions, dates back to the mid-20th century. However, it wasn’t until the advent of computational technology that AI’s potential started to be realized. The initial focus was on rule-based systems and expert systems, where explicit rules were programmed to make decisions based on a set of conditions. This approach had limitations, as it required domain experts to manually encode rules for every scenario, making it challenging to handle complex and dynamic tasks.
Machine Learning (ML) emerged as a paradigm shift, enabling machines to learn patterns and insights from data rather than relying solely on programmed rules. ML algorithms could adapt and improve their performance over time, making them capable of handling a wider range of tasks. The key idea behind ML is to train algorithms on data so that they can recognize patterns and make predictions or decisions based on new, unseen data. This marked the first significant leap in the evolution of AI.
2. The Rise of Machine Learning: Key Concepts and Techniques
Machine learning can be categorized into supervised, unsupervised, and reinforcement learning, each catering to different types of tasks and data. Let’s delve into these categories:
2.1. Supervised Learning:
Supervised learning involves training a model on labeled data, where the input data is paired with the correct output or label. The model learns to map inputs to outputs by generalizing patterns from the training data. Common algorithms include decision trees, support vector machines (SVMs), and the ever-popular neural networks.
Code Sample 1: Training a Decision Tree Classifier
python from sklearn.tree import DecisionTreeClassifier # Sample data X = [[0, 0], [1, 1]] y = [0, 1] # Create a decision tree classifier clf = DecisionTreeClassifier() # Train the classifier on the data clf.fit(X, y) # Make predictions new_data = [[0.8, 0.8]] predictions = clf.predict(new_data) print(predictions) # Output: [1]
2.2. Unsupervised Learning:
Unsupervised learning deals with unlabeled data, where the model aims to discover patterns or structures within the data. Clustering and dimensionality reduction are common tasks within this category. K-Means clustering and Principal Component Analysis (PCA) are widely used techniques.
Code Sample 2: K-Means Clustering
python from sklearn.cluster import KMeans # Sample data X = [[1, 2], [1.5, 1.8], [5, 8], [8, 8], [1, 0.6], [9, 11]] # Create a KMeans clusterer kmeans = KMeans(n_clusters=2) # Fit the model to the data kmeans.fit(X) # Get cluster labels for each data point labels = kmeans.labels_ print(labels) # Output: [0, 0, 1, 1, 0, 1]
2.3. Reinforcement Learning:
Reinforcement learning involves an agent learning to take actions in an environment to maximize a reward. The agent learns through trial and error, receiving feedback on the quality of its actions. Deep Q-Networks (DQNs) are a popular approach within reinforcement learning.
Code Sample 3: Training a Deep Q-Network
python import gym import numpy as np from keras.models import Sequential from keras.layers import Dense from keras.optimizers import Adam # Create a neural network model model = Sequential() model.add(Dense(24, input_shape=(state_size,), activation='relu')) model.add(Dense(24, activation='relu')) model.add(Dense(action_size, activation='linear')) model.compile(loss='mse', optimizer=Adam(lr=learning_rate)) # Define the Q-learning algorithm def q_learning(state, action, reward, next_state, done): target = reward + gamma * np.max(model.predict(next_state)[0]) target_vec = model.predict(state) target_vec[0][action] = target model.fit(state, target_vec, epochs=1, verbose=0) # Training loop for episode in range(num_episodes): state = env.reset() for step in range(max_steps_per_episode): action = choose_action(state) next_state, reward, done, _ = env.step(action) q_learning(state, action, reward, next_state, done) if done: break state = next_state
3. The Revolution of Deep Learning: Unlocking Unprecedented Potential
While traditional machine learning paved the way for AI advancement, its potential was limited by the need for feature engineering and its inability to effectively handle complex, unstructured data such as images, audio, and text. The arrival of deep learning revolutionized AI by introducing neural networks with multiple layers (deep neural networks). These networks could automatically learn intricate features from raw data, significantly reducing the reliance on manual feature engineering.
3.1. The Birth of Deep Learning
Deep learning’s breakthrough came with the advent of Convolutional Neural Networks (CNNs) for image analysis and Recurrent Neural Networks (RNNs) for sequence data. CNNs use convolutional layers to detect local patterns in images, enabling them to excel at tasks like image classification, object detection, and facial recognition. RNNs, on the other hand, can capture sequential dependencies in data, making them suitable for tasks like language modeling, machine translation, and speech recognition.
3.1.1. Convolutional Neural Networks (CNNs)
CNNs have transformed the field of computer vision, enabling machines to perceive and understand visual information. The architecture consists of convolutional layers that apply filters to extract features from images. These features are then fed into fully connected layers for classification or regression.
Code Sample 4: Building a Simple CNN for Image Classification
python import tensorflow as tf from tensorflow.keras import layers, models # Create a simple CNN model model = models.Sequential() model.add(layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1))) model.add(layers.MaxPooling2D((2, 2))) model.add(layers.Conv2D(64, (3, 3), activation='relu')) model.add(layers.MaxPooling2D((2, 2))) model.add(layers.Conv2D(64, (3, 3), activation='relu')) model.add(layers.Flatten()) model.add(layers.Dense(64, activation='relu')) model.add(layers.Dense(10, activation='softmax')) # Compile the model model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
3.1.2. Recurrent Neural Networks (RNNs)
RNNs excel in handling sequential data, where the order of elements matters. They maintain a hidden state that captures information from previous steps, allowing them to capture temporal dependencies. However, traditional RNNs suffer from vanishing gradient problems, which limit their ability to capture long-range dependencies.
Code Sample 5: Building a Simple RNN for Sequence Prediction
python import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import SimpleRNN, Dense # Create a simple RNN model model = Sequential() model.add(SimpleRNN(32, input_shape=(None, 1), return_sequences=True)) model.add(SimpleRNN(32, return_sequences=True)) model.add(Dense(1)) # Compile the model model.compile(optimizer='adam', loss='mean_squared_error')
3.2. The Emergence of Deep Learning Architectures
As deep learning gained momentum, new architectures were developed to tackle specific challenges. Two notable architectures are Generative Adversarial Networks (GANs) and Long Short-Term Memory (LSTM) networks.
3.2.1. Generative Adversarial Networks (GANs)
GANs introduced a novel concept of pitting two neural networks against each other: a generator and a discriminator. The generator aims to create realistic data, while the discriminator’s task is to distinguish real data from generated data. This adversarial process leads to the generation of highly realistic images, audio, and even text.
Code Sample 6: Building a Simple GAN
python import tensorflow as tf from tensorflow.keras import layers, models # Generator model generator = models.Sequential([ layers.Dense(128, input_shape=(random_dim,), activation='relu'), layers.Dense(784, activation='sigmoid'), layers.Reshape((28, 28, 1)) ]) # Discriminator model discriminator = models.Sequential([ layers.Flatten(input_shape=(28, 28, 1)), layers.Dense(128, activation='relu'), layers.Dense(1, activation='sigmoid') ]) # Compile the discriminator discriminator.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # Combined GAN model discriminator.trainable = False gan_input = tf.keras.Input(shape=(random_dim,)) x = generator(gan_input) gan_output = discriminator(x) gan = models.Model(gan_input, gan_output) gan.compile(optimizer='adam', loss='binary_crossentropy')
3.2.2. Long Short-Term Memory (LSTM) Networks
LSTM networks are a type of RNN that addresses the vanishing gradient problem by introducing memory cells and gating mechanisms. This architecture is particularly effective for tasks involving sequences of varying lengths, like language modeling and sentiment analysis.
Code Sample 7: Building an LSTM Network for Text Generation
python import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Embedding, LSTM, Dense # Create an LSTM model for text generation model = Sequential() model.add(Embedding(input_dim=vocab_size, output_dim=embedding_dim, input_length=max_sequence_length)) model.add(LSTM(128)) model.add(Dense(vocab_size, activation='softmax')) # Compile the model model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
Conclusion
The evolution of AI from traditional machine learning to deep learning has been a journey marked by transformative breakthroughs. With deep learning’s ability to autonomously learn intricate patterns from raw data, AI has achieved remarkable progress in various domains, from computer vision and natural language processing to healthcare and autonomous driving. As technology continues to advance, the boundaries of what AI can achieve will be continually pushed, opening up new possibilities for innovation and improving the human experience.
In this blog, we’ve explored the foundational concepts of machine learning, journeyed through the rise of deep learning, and delved into various deep learning architectures. From supervised learning with decision trees to the adversarial magic of GANs, we’ve seen the evolution that has brought us to the forefront of AI’s potential. The future is bound to bring even more remarkable developments, making the path from machine learning to deep learning just the beginning of an exciting AI revolution.
Table of Contents
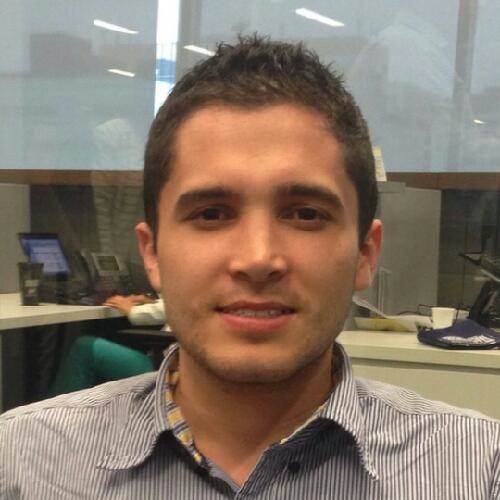
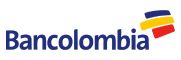