AI Development and Natural Disaster Management: Early Warning Systems
Natural disasters pose significant risks to communities worldwide. Developing effective early warning systems is crucial for minimizing the impact of these events. AI, with its advanced predictive capabilities, offers powerful tools for enhancing natural disaster management. This article explores how AI can be used to develop and implement early warning systems, with practical examples of predictive modeling and data analysis.
Understanding Early Warning Systems
Early warning systems are designed to predict and provide alerts for impending natural disasters, giving communities time to prepare and respond. Effective early warning systems require the integration of real-time data, predictive modeling, and communication networks to function optimally.
Using AI for Disaster Prediction and Analysis
AI can process large datasets, identify patterns, and make predictions more accurately than traditional methods. Below are some key aspects and code examples demonstrating how AI can be employed in early warning systems for natural disaster management.
-
Data Collection and Preprocessing
The first step in developing an AI-based early warning system is collecting and preprocessing relevant data. AI algorithms rely on historical and real-time data to make accurate predictions.
Example: Preprocessing Weather Data for AI Models
Assume you have a dataset containing historical weather data. You can use Python and libraries like `pandas` and `scikit-learn` to preprocess this data for AI model training.
```python import pandas as pd from sklearn.preprocessing import StandardScaler # Load the dataset data = pd.read_csv("weather_data.csv") # Handle missing values data.fillna(method='ffill', inplace=True) # Feature scaling scaler = StandardScaler() scaled_data = scaler.fit_transform(data[['temperature', 'humidity', 'pressure']]) # The scaled data is now ready for model training ```
-
Predictive Modeling
AI can be used to create predictive models that forecast the likelihood of natural disasters such as floods, hurricanes, and earthquakes. Machine learning algorithms can be trained on historical data to predict future events.
Example: Predicting Floods with a Neural Network
Here’s how you might build a simple neural network model to predict floods based on weather data.
```python from keras.models import Sequential from keras.layers import Dense # Build the model model = Sequential() model.add(Dense(64, input_dim=3, activation='relu')) model.add(Dense(32, activation='relu')) model.add(Dense(1, activation='sigmoid')) # Compile the model model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # Train the model model.fit(scaled_data, labels, epochs=50, batch_size=10) ```
3. Real-Time Monitoring and Alerts
AI-powered early warning systems require continuous monitoring of real-time data to generate alerts promptly. AI can analyze streaming data from sensors, satellites, and other sources to detect anomalies indicative of a potential disaster.
Example: Real-Time Earthquake Detection Using AI
```python import numpy as np import tensorflow as tf # Simulated real-time sensor data sensor_data = np.array([[0.02, 0.01, 0.98]]) # Load a pre-trained model model = tf.keras.models.load_model('earthquake_model.h5') # Predict if an earthquake is imminent prediction = model.predict(sensor_data) if prediction > 0.8: print("Earthquake Warning: High probability of an earthquake detected!") ```
4. Visualization and Decision Support
Visualization tools are essential for interpreting AI predictions and making informed decisions. AI-generated predictions can be visualized on dashboards, maps, or other interfaces to assist disaster management teams.
Example: Visualizing Flood Risk Areas with GIS
```python import geopandas as gpd import matplotlib.pyplot as plt # Load geographic data flood_data = gpd.read_file("flood_risk_areas.shp") # Plot flood risk areas flood_data.plot() plt.title("Flood Risk Areas") plt.show() ```
5. Integration with Communication Systems
An effective early warning system must integrate with communication networks to disseminate alerts to authorities and the public. AI can optimize the timing and delivery of these alerts to ensure maximum reach and effectiveness.
Example: Sending Automated Alerts Using AI
```python import smtplib from email.mime.text import MIMEText def send_alert(message): # Email configuration msg = MIMEText(message) msg['Subject'] = 'Natural Disaster Alert' msg['From'] = 'alert@disastermanagement.com' msg['To'] = 'recipient@example.com' # Send the email with smtplib.SMTP('smtp.example.com') as server: server.login('username', 'password') server.send_message(msg) # Example usage send_alert("Warning: Flood risk detected in your area. Please take necessary precautions.") ```
Conclusion
AI offers significant potential for enhancing early warning systems in natural disaster management. By leveraging AI’s predictive capabilities, organizations can better prepare for and respond to natural disasters, ultimately reducing their impact. The integration of AI into early warning systems represents a crucial advancement in safeguarding communities from the devastating effects of natural disasters.
Further Reading:
Table of Contents
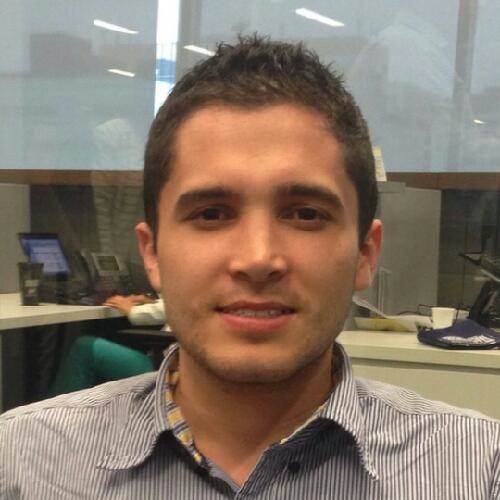
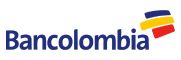