AI Development and Natural Language Understanding in Virtual Assistants
Introduction to Natural Language Understanding (NLU)
Natural Language Understanding (NLU) is a critical component in the development of intelligent virtual assistants. NLU enables these systems to comprehend, interpret, and respond to user inputs in a way that feels natural and intuitive. With the rapid advancements in AI, NLU has become a pivotal factor in enhancing user experiences, making interactions with virtual assistants more efficient and human-like.
The Role of AI in NLU for Virtual Assistants
Artificial Intelligence (AI) drives the sophistication of NLU in virtual assistants, allowing these systems to handle complex queries, context-aware responses, and even emotional understanding. This section explores the various AI techniques and models that contribute to the development of NLU in virtual assistants.
1. Building an NLU Pipeline
Creating an effective NLU system involves several key components, including tokenization, intent recognition, and entity extraction. AI models like Transformer-based architectures (e.g., BERT, GPT) are instrumental in these tasks.
Example: Tokenization and Intent Recognition
Consider a virtual assistant that processes a user’s request to “book a flight to New York.” The first step is tokenizing the sentence and recognizing the user’s intent.
```python from transformers import pipeline Initialize a pre-trained model pipeline for intent recognition nlp = pipeline("zero-shot-classification", model="facebook/bart-large-mnli") User input user_input = "Book a flight to New York" Possible intents intents = ["book_flight", "cancel_booking", "weather_query"] Recognize intent result = nlp(user_input, intents) print(f"Recognized intent: {result['labels'][0]}") ```
2. Contextual Understanding
One of the most challenging aspects of NLU is maintaining context across multiple user interactions. AI models like RNNs and Transformers excel at capturing the context, enabling virtual assistants to follow conversations seamlessly.
Example: Contextual Response Generation
Here’s an example using a Transformer model to generate context-aware responses in a virtual assistant.
```python from transformers import GPT2LMHeadModel, GPT2Tokenizer Load pre-trained model and tokenizer tokenizer = GPT2Tokenizer.from_pretrained("gpt2") model = GPT2LMHeadModel.from_pretrained("gpt2") Simulate a conversation conversation = [ "User: What's the weather like today?", "Assistant: It's sunny and 75 degrees.", "User: Should I wear a jacket?", ] Generate a response considering context input_ids = tokenizer.encode(" ".join(conversation), return_tensors="pt") output = model.generate(input_ids, max_length=50) response = tokenizer.decode(output[0], skip_special_tokens=True) print(response) ```
3. Emotion Detection in Conversations
To make virtual assistants more empathetic, AI models can be trained to detect and respond to users’ emotions. This can be achieved through sentiment analysis, where the system gauges the emotional tone of the user’s input and adjusts its responses accordingly.
Example: Sentiment Analysis for Emotion Detection
Using a sentiment analysis model, a virtual assistant can detect whether the user is happy, sad, or frustrated.
```python from transformers import pipeline Initialize sentiment analysis pipeline sentiment_pipeline = pipeline("sentiment-analysis") User input with emotional content user_input = "I'm really upset with the service I received." Analyze sentiment sentiment = sentiment_pipeline(user_input) print(f"Detected sentiment: {sentiment[0]['label']} with score {sentiment[0]['score']}") ```
4. Personalization through NLU
Personalizing interactions is key to creating a more engaging user experience. NLU can be enhanced by incorporating user preferences and historical data to deliver customized responses.
Example: Personalized Recommendations
Here’s how an AI-driven virtual assistant might suggest a restaurant based on user preferences.
```python Simulated user preference data user_preferences = { "cuisine": "Italian", "location": "downtown", "price_range": "medium" } Function to generate personalized restaurant recommendation def recommend_restaurant(preferences): Sample recommendations based on user preferences recommendations = [ {"name": "Luigi's Italian Bistro", "location": "downtown", "price_range": "medium"}, {"name": "Mamma Mia", "location": "uptown", "price_range": "high"} ] Filter recommendations based on preferences suitable_options = [r for r in recommendations if r["location"] == preferences["location"] and r["price_range"] == preferences["price_range"]] if suitable_options: return f"How about {suitable_options[0]['name']} for dinner tonight?" else: return "Sorry, I couldn't find any restaurants matching your preferences." Get recommendation print(recommend_restaurant(user_preferences)) ```
Conclusion
Advancements in AI have significantly enhanced the capabilities of virtual assistants through improved Natural Language Understanding. By integrating AI techniques such as intent recognition, contextual understanding, emotion detection, and personalization, virtual assistants are becoming more intelligent and user-friendly. As AI continues to evolve, we can expect even more sophisticated NLU systems that will further blur the lines between human and machine interactions.
Further Reading:
Table of Contents
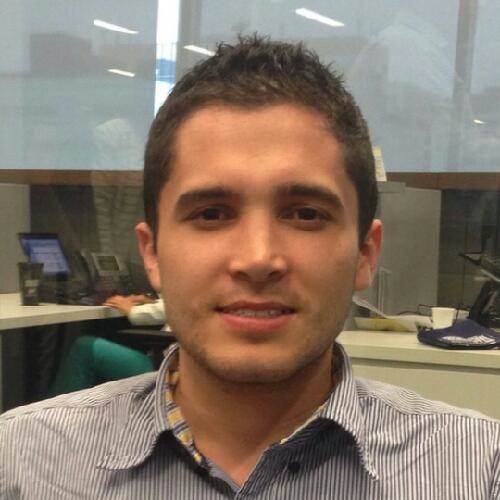
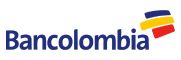