PyTorch: Deep Learning and AI Development
In the rapidly evolving field of artificial intelligence, deep learning has emerged as a game-changer. Among the numerous tools and frameworks available, PyTorch has gained significant popularity due to its flexibility, ease of use, and extensive support from the research community. This blog will take you on a journey through the fundamentals of PyTorch, showcasing its capabilities, code samples, and how it is leveraged in AI development.
1. Understanding PyTorch
1.1 What is PyTorch?
PyTorch is an open-source machine learning library developed by Facebook’s AI Research lab (FAIR). It is based on the Torch library and provides a flexible and dynamic computational graph, making it a top choice for researchers and developers alike. PyTorch is renowned for its support of tensor operations and its unique feature, “autograd,” which enables automatic differentiation.
1.2 Key Features of PyTorch
- Dynamic Computational Graphs: Unlike other frameworks, PyTorch employs dynamic computation, allowing for efficient handling of variable-sized inputs and dynamic structures.
- Automatic Differentiation: With PyTorch’s “autograd” feature, it automatically computes gradients for tensor operations, simplifying the process of implementing complex neural networks.
- TorchScript: PyTorch enables easy integration between eager mode and TorchScript, offering both flexibility and performance.
- Support for GPU Acceleration: PyTorch’s seamless GPU support enhances the training speed of deep learning models significantly.
- Rich Ecosystem: The framework boasts a vast ecosystem of libraries, tools, and pre-trained models, fostering rapid development and experimentation.
2. Getting Started with PyTorch
2.1 Installation
To get started with PyTorch, ensure you have Python installed. Then, use pip to install the library:
bash pip install torch torchvision
2.2 Tensors: The Foundation of PyTorch
At the core of PyTorch are tensors, which are similar to NumPy arrays but with added GPU support. Tensors enable easy computation and automatic differentiation, making them ideal for building neural networks. Let’s create a simple tensor:
python import torch # Create a 1-dimensional tensor x = torch.tensor([1, 2, 3, 4, 5]) print(x)
2.3 Automatic Differentiation with PyTorch
The automatic differentiation feature in PyTorch is crucial for training complex neural networks. It automatically tracks operations performed on tensors and calculates gradients. Here’s an example of how to use automatic differentiation:
python import torch # Define a tensor with requires_grad=True to enable automatic differentiation x = torch.tensor(2.0, requires_grad=True) # Define a function (e.g., y = x^2 + 3x + 1) y = x**2 + 3*x + 1 # Compute gradients y.backward() # Access the gradients print(x.grad)
2.4 Building a Neural Network
PyTorch simplifies the process of building neural networks with its torch.nn module. Here’s a basic example of creating a simple feedforward neural network:
python import torch import torch.nn as nn class SimpleNeuralNet(nn.Module): def __init__(self): super(SimpleNeuralNet, self).__init__() self.fc1 = nn.Linear(784, 128) self.fc2 = nn.Linear(128, 10) def forward(self, x): x = torch.relu(self.fc1(x)) x = self.fc2(x) return x # Create an instance of the neural network model = SimpleNeuralNet()
2.5 Data Loading with PyTorch
To train a neural network, you need to load and preprocess data. PyTorch offers the torch.utils.data module to facilitate this. Here’s a snippet demonstrating data loading for a classification task:
python import torch from torchvision import datasets, transforms # Define transformations for the data transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))]) # Load MNIST dataset train_dataset = datasets.MNIST(root="./data", train=True, transform=transform, download=True) train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=64, shuffle=True)
2.6 Training and Evaluating a Model
Now that we have a basic understanding of PyTorch, let’s train and evaluate our previously defined neural network:
python import torch import torch.nn as nn import torch.optim as optim from torchvision import datasets, transforms # ... (Previous code for model definition and data loading) # Define the loss function and optimizer criterion = nn.CrossEntropyLoss() optimizer = optim.SGD(model.parameters(), lr=0.01) # Training loop epochs = 10 for epoch in range(epochs): running_loss = 0.0 for inputs, labels in train_loader: optimizer.zero_grad() outputs = model(inputs.view(-1, 784)) loss = criterion(outputs, labels) loss.backward() optimizer.step() running_loss += loss.item() print(f"Epoch {epoch + 1}/{epochs}, Loss: {running_loss / len(train_loader)}") # Evaluation correct = 0 total = 0 with torch.no_grad(): for inputs, labels in test_loader: outputs = model(inputs.view(-1, 784)) _, predicted = torch.max(outputs.data, 1) total += labels.size(0) correct += (predicted == labels).sum().item() print(f"Accuracy on the test set: {100 * correct / total}%")
3. Advanced Features of PyTorch
3.1 Transfer Learning
Transfer learning is a popular technique that allows us to leverage pre-trained models for our tasks. PyTorch provides an extensive collection of pre-trained models through the torchvision.models module. Here’s an example of how to use transfer learning:
python import torch import torch.nn as nn import torchvision.models as models # Load a pre-trained ResNet model model = models.resnet18(pretrained=True) # Freeze the pre-trained layers for param in model.parameters(): param.requires_grad = False # Replace the fully connected layer to match the number of classes in the new task num_classes = 10 model.fc = nn.Linear(model.fc.in_features, num_classes)
3.2 Distributed Training
PyTorch supports distributed training across multiple GPUs and machines using torch.distributed package. It enables scaling up deep learning models for large datasets and complex tasks. Here’s a high-level example of distributed training:
python import torch import torch.distributed as dist import torch.nn as nn import torch.optim as optim from torch.utils.data import DataLoader from torchvision import datasets, transforms # Initialize distributed training dist.init_process_group(backend="nccl") # Define the model and optimizer model = SimpleNeuralNet() model = model.to(dist.get_rank()) # Move the model to the current GPU optimizer = optim.SGD(model.parameters(), lr=0.01) # Load data and distribute it across GPUs train_dataset = datasets.MNIST(root="./data", train=True, transform=transforms.ToTensor(), download=True) train_sampler = torch.utils.data.distributed.DistributedSampler(train_dataset) train_loader = DataLoader(train_dataset, batch_size=64, sampler=train_sampler) # Distributed training loop epochs = 10 for epoch in range(epochs): model.train() for inputs, labels in train_loader: inputs = inputs.to(dist.get_rank()) labels = labels.to(dist.get_rank()) optimizer.zero_grad() outputs = model(inputs.view(-1, 784)) loss = criterion(outputs, labels) loss.backward() optimizer.step() # Synchronize the gradients across all processes for param in model.parameters(): dist.all_reduce(param.grad.data, op=dist.ReduceOp.SUM) param.grad.data /= dist.get_world_size() # Clean up distributed training dist.destroy_process_group()
Conclusion
PyTorch has emerged as a leading framework for deep learning and AI development, thanks to its flexibility, ease of use, and extensive community support. In this blog, we explored the basics of PyTorch, including tensors, automatic differentiation, and building neural networks. We also delved into advanced features like transfer learning and distributed training. Now equipped with this knowledge, you can dive deeper into the world of PyTorch, unlocking endless possibilities for your AI projects. Happy coding and exploring!
Remember, AI development is an ongoing journey, and PyTorch is your faithful companion in this exciting ride!
Table of Contents
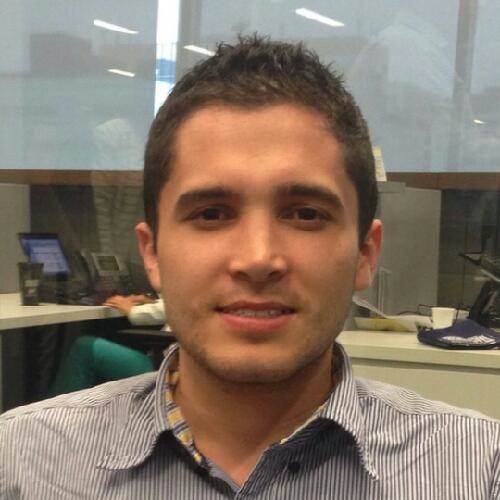
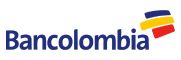