AI Development and Quality Control: Ensuring Product Excellence
Quality control is vital in product development to ensure that products meet the desired standards and specifications. With the advent of AI technologies, quality control processes can be significantly improved, offering more precise, efficient, and automated solutions. This blog explores how AI can be harnessed to elevate quality control practices and ensure product excellence.
Understanding Quality Control in Product Development
Quality control (QC) involves the processes and procedures that ensure a product meets the required standards and specifications. Effective QC helps in identifying defects, improving product reliability, and enhancing customer satisfaction.
Utilizing AI for Quality Control
AI offers powerful tools and techniques that can enhance quality control processes. Below are some key aspects and practical examples demonstrating how AI can be employed to ensure product excellence.
1. Automated Defect Detection
AI-powered systems can automate the detection of defects in products by analyzing images or data from production lines. Machine learning algorithms can be trained to identify defects with high accuracy.
Example: Using a Convolutional Neural Network (CNN) for Image-Based Defect Detection
```python import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense # Define the CNN model model = Sequential([ Conv2D(32, (3, 3), activation='relu', input_shape=(128, 128, 3)), MaxPooling2D(pool_size=(2, 2)), Conv2D(64, (3, 3), activation='relu'), MaxPooling2D(pool_size=(2, 2)), Flatten(), Dense(128, activation='relu'), Dense(1, activation='sigmoid') ]) # Compile the model model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # Train the model on defect detection data # model.fit(train_images, train_labels, epochs=10, batch_size=32) ```
2. Predictive Maintenance
AI can predict when equipment is likely to fail, allowing for timely maintenance before a breakdown occurs. This proactive approach helps in maintaining production quality and avoiding costly downtimes.
Example: Predictive Maintenance Using Time Series Analysis
```python import pandas as pd from sklearn.ensemble import RandomForestRegressor from sklearn.model_selection import train_test_split # Load time series data data = pd.read_csv('maintenance_data.csv') # Feature and target variable X = data[['feature1', 'feature2', 'feature3']] y = data['time_to_failure'] # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train the model model = RandomForestRegressor() model.fit(X_train, y_train) # Predict time to failure # predictions = model.predict(X_test) ```
3. Process Optimization
AI algorithms can analyze production processes to identify inefficiencies and suggest improvements. This optimization can lead to higher product quality and reduced production costs.
Example: Using Reinforcement Learning for Process Optimization
```python import gym from stable_baselines3 import PPO # Create an environment env = gym.make('ProductionLine-v0') # Define the reinforcement learning model model = PPO('MlpPolicy', env, verbose=1) # Train the model model.learn(total_timesteps=10000) # Optimize the production process based on the trained model # model.predict(observation) ```
4. Quality Prediction
AI can predict the quality of products based on various factors and inputs. This predictive capability helps in adjusting production parameters to ensure high-quality outputs.
Example: Predicting Product Quality Using Regression Analysis
```python import pandas as pd from sklearn.linear_model import LinearRegression from sklearn.model_selection import train_test_split # Load quality prediction data data = pd.read_csv('quality_data.csv') # Feature and target variable X = data[['feature1', 'feature2', 'feature3']] y = data['quality_score'] # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Train the model model = LinearRegression() model.fit(X_train, y_train) # Predict product quality # predictions = model.predict(X_test) ```
Conclusion
AI technologies offer transformative potential for quality control in product development. From automating defect detection and predicting maintenance needs to optimizing production processes and predicting product quality, AI can significantly enhance quality control practices. Embracing these AI-driven approaches leads to superior product excellence and increased customer satisfaction.
Further Reading:
Table of Contents
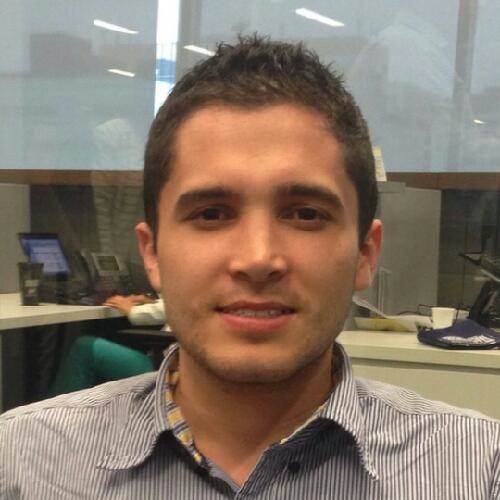
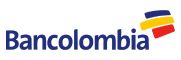