AI Development and Speech Recognition: Enabling Voice Interfaces
Speech recognition technology is revolutionizing the way we interact with machines, enabling voice interfaces that offer a more natural and intuitive user experience. By leveraging AI, developers can create systems that accurately interpret and respond to spoken language, making applications more accessible and efficient.
Understanding Speech Recognition in AI
Speech recognition involves converting spoken language into text or commands that a machine can understand. AI plays a crucial role in improving the accuracy and efficiency of speech recognition systems by learning from vast datasets and adapting to different accents, dialects, and languages.
Developing Speech Recognition Systems with AI
AI-based speech recognition systems typically involve several key components: data collection, model training, real-time processing, and integration with voice interfaces. Below are some examples demonstrating how AI can be used in developing speech recognition systems.
1. Collecting and Preparing Voice Data
The first step in building a speech recognition system is to collect and prepare voice data. This data is used to train AI models to recognize and understand spoken words.
Example: Collecting Voice Data
You can use tools like `Google Speech-to-Text` API to collect and transcribe voice data, which can then be used to train your AI model.
```python import os from google.cloud import speech_v1p1beta1 as speech def transcribe_audio(audio_file): client = speech.SpeechClient() with open(audio_file, 'rb') as audio: audio_content = audio.read() audio = speech.RecognitionAudio(content=audio_content) config = speech.RecognitionConfig( encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16, sample_rate_hertz=16000, language_code='en-US', ) response = client.recognize(config=config, audio=audio) for result in response.results: print(f'Transcript: {result.alternatives[0].transcript}') # Example usage transcribe_audio('path/to/audio/file.wav') ```
2. Training AI Models for Speech Recognition
Once you have collected sufficient voice data, the next step is to train AI models. Machine learning frameworks like TensorFlow or PyTorch are commonly used for this purpose.
Example: Training a Simple Speech Recognition Model
Here’s a basic example of how to train a neural network model using TensorFlow for speech recognition.
```python import tensorflow as tf from tensorflow.keras import layers, models # Load dataset (e.g., from a dataset like LibriSpeech) # (train_audio, train_labels), (test_audio, test_labels) = ... # Define the model architecture model = models.Sequential([ layers.Input(shape=(16000, 1)), # Assuming 1-second audio clips layers.Conv1D(16, kernel_size=3, activation='relu'), layers.MaxPooling1D(pool_size=2), layers.Conv1D(32, kernel_size=3, activation='relu'), layers.MaxPooling1D(pool_size=2), layers.Flatten(), layers.Dense(64, activation='relu'), layers.Dense(10, activation='softmax') # Assuming 10 different commands ]) # Compile and train the model model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) model.fit(train_audio, train_labels, epochs=10, validation_data=(test_audio, test_labels)) ```
3. Real-Time Speech Processing
Real-time speech recognition requires efficient processing of audio streams. AI can be used to process and interpret spoken commands on the fly, enabling interactive voice interfaces.
Example: Real-Time Speech Recognition
This example demonstrates how to implement real-time speech recognition using a pre-trained model.
```python import speech_recognition as sr def recognize_speech_from_mic(): recognizer = sr.Recognizer() mic = sr.Microphone() with mic as source: recognizer.adjust_for_ambient_noise(source) print("Listening...") audio = recognizer.listen(source) try: transcript = recognizer.recognize_google(audio) print(f"You said: {transcript}") except sr.UnknownValueError: print("Could not understand the audio") except sr.RequestError: print("Error with the speech recognition service") # Example usage recognize_speech_from_mic() ```
4. Integrating with Voice Interfaces
The final step is to integrate the speech recognition model with a voice interface, allowing users to interact with applications via spoken commands.
Example: Building a Simple Voice-Controlled Application
Here’s a simple example of how to build a voice-controlled application that responds to user commands.
```python import pyttsx3 def respond_to_command(command): engine = pyttsx3.init() if 'hello' in command: engine.say("Hello! How can I help you?") elif 'time' in command: engine.say("The current time is 2 PM.") else: engine.say("Sorry, I didn't understand that command.") engine.runAndWait() # Example usage recognize_speech_from_mic() ```
Conclusion
AI has significantly advanced the field of speech recognition, enabling more accurate and responsive voice interfaces. From collecting and processing voice data to training models and integrating with applications, AI plays a pivotal role in making voice interactions more accessible and efficient. Leveraging these technologies can lead to innovative solutions and more natural user experiences.
Further Reading:
Table of Contents
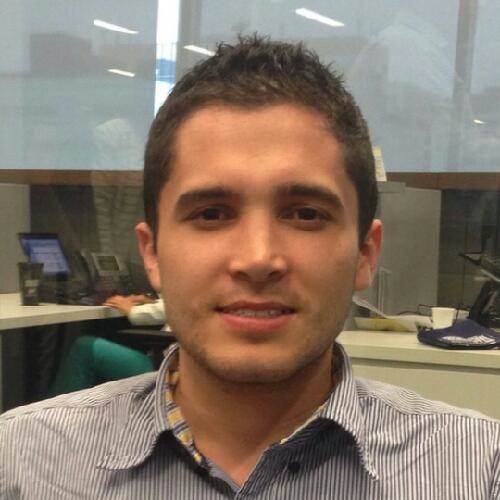
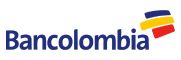