Creating AI-powered Web Applications with Django
In today’s rapidly evolving technological landscape, integrating artificial intelligence into web applications has become a game-changer. By harnessing the capabilities of AI, developers can create intelligent, responsive, and personalized web experiences that cater to user needs like never before. Django, a high-level Python web framework, provides an excellent foundation for building AI-powered web applications that can process data, make predictions, and adapt to user behavior.
In this comprehensive guide, we’ll walk you through the process of creating AI-powered web applications using Django. Whether you’re a seasoned Django developer or just starting, this guide will provide you with the insights and tools you need to infuse your projects with AI capabilities.
1. Introduction to AI-Powered Web Applications
1.1. What are AI-powered web applications?
AI-powered web applications leverage machine learning algorithms and artificial intelligence techniques to enhance user experiences and automate complex tasks. These applications can understand user preferences, make intelligent recommendations, process natural language, recognize patterns in images, and perform real-time data analysis, among other capabilities.
1.2. Benefits of integrating AI into web apps
- Personalization: AI enables web apps to personalize content and recommendations based on user behavior and preferences, resulting in a more engaging user experience.
- Efficiency: Tasks that would otherwise be time-consuming or error-prone can be automated with AI, freeing up valuable human resources.
- Data Analysis: AI-powered apps can analyze large volumes of data in real-time, providing valuable insights and facilitating data-driven decision-making.
- User Engagement: Chatbots and virtual assistants can improve user engagement by providing instant assistance and support.
2. Getting Started with Django
Django provides a solid foundation for building web applications with AI capabilities. If you’re new to Django, here’s a brief overview of how to get started:
2.1. Setting up a Django project
Install Python: Ensure you have Python installed on your system. You can download it from the official website.
Install Django: Use pip, Python’s package manager, to install Django. Run the following command in your terminal:
bash pip install Django
Create a project: Create a new Django project by running the following command:
bash django-admin startproject projectname
2.2. Creating Django apps for AI integration
Django follows the concept of apps, which are modular components that can be added to your project. To integrate AI capabilities, you can create separate apps dedicated to specific AI features.
Create a new app: Navigate to your project directory and run the following command:
bash python manage.py startapp aiapp
Configure the app: Add the newly created app to the INSTALLED_APPS list in the project’s settings.py file.
3. Machine Learning and AI Integration
Choosing the right AI technologies is crucial for the success of your AI-powered web application. Depending on the tasks you want to accomplish, you might consider technologies such as:
- Machine Learning Libraries: TensorFlow, PyTorch, scikit-learn
- Natural Language Processing Tools: NLTK, spaCy
- Computer Vision Frameworks: OpenCV, TensorFlow Object Detection API
Collect and prepare data relevant to your AI tasks. Clean and preprocess the data to ensure accurate results from your AI models.
4. Building AI-Powered Features
4.1. Sentiment analysis for user-generated content
Implement sentiment analysis using machine learning to understand the sentiment behind user-generated content, such as reviews or comments. Use pre-trained models or train your own using labeled data.
python # Sample code for sentiment analysis with NLTK import nltk from nltk.sentiment.vader import SentimentIntensityAnalyzer nltk.download('vader_lexicon') def analyze_sentiment(text): sia = SentimentIntensityAnalyzer() sentiment_score = sia.polarity_scores(text) if sentiment_score['compound'] >= 0.05: return 'Positive' elif sentiment_score['compound'] <= -0.05: return 'Negative' else: return 'Neutral'
4.2. Personalized recommendations using collaborative filtering
Implement collaborative filtering to provide personalized recommendations to users based on their interactions and preferences. This involves building a recommendation engine that suggests items similar to those the user has shown interest in.
python # Sample code for collaborative filtering with scikit-learn from sklearn.metrics.pairwise import cosine_similarity from sklearn.preprocessing import normalize def collaborative_filtering(user_preferences): # Create a user-item matrix and normalize it user_item_matrix = normalize(user_preferences) # Calculate cosine similarity between items item_similarity = cosine_similarity(user_item_matrix.T) # Generate recommendations for a specific user user_id = 123 user_vector = user_item_matrix[user_id] recommendation_scores = item_similarity.dot(user_vector) recommended_items = recommendation_scores.argsort()[::-1] return recommended_items
5. Natural Language Processing (NLP) Capabilities
5.1. Creating a chatbot interface with Django
Integrate a chatbot into your web application to provide real-time assistance and information retrieval for users.
python # Sample code for creating a simple chatbot using ChatterBot library from chatterbot import ChatBot from chatterbot.trainers import ChatterBotCorpusTrainer chatbot = ChatBot('MyChatBot') trainer = ChatterBotCorpusTrainer(chatbot) # Train the chatbot on English language data trainer.train('chatterbot.corpus.english') def get_chatbot_response(user_input): response = chatbot.get_response(user_input) return str(response)
5.2. Implementing AI-driven language translation
Enable your web app to translate text between different languages using AI-powered translation models.
python # Sample code for language translation using the Google Cloud Translation API from google.cloud import translate_v2 as translate def translate_text(text, target_language): client = translate.Client() translated_text = client.translate(text, target_language=target_language) return translated_text['translatedText']
6. Computer Vision Integration
6.1. Image recognition for content categorization
Implement image recognition to automatically categorize and tag images uploaded by users.
python # Sample code for image recognition using TensorFlow and Keras import tensorflow as tf from tensorflow.keras.applications import MobileNetV2 from tensorflow.keras.applications.mobilenet_v2 import preprocess_input, decode_predictions from tensorflow.keras.preprocessing import image import numpy as np def recognize_image(image_path): model = MobileNetV2(weights='imagenet') img = image.load_img(image_path, target_size=(224, 224)) img_array = image.img_to_array(img) img_array = np.expand_dims(img_array, axis=0) img_array = preprocess_input(img_array) predictions = model.predict(img_array) decoded_predictions = decode_predictions(predictions) return decoded_predictions
6.2. Facial recognition for enhanced user experiences
Implement facial recognition to provide personalized experiences based on user identities.
python # Sample code for facial recognition using OpenCV import cv2 def recognize_face(image_path, known_faces): image = cv2.imread(image_path) gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Load a pre-trained face recognition model face_recognizer = cv2.face.LBPHFaceRecognizer_create() face_recognizer.read('face_recognizer_model.xml') label, confidence = face_recognizer.predict(gray) if confidence < 100: recognized_person = known_faces[label] return recognized_person else: return "Unknown"
7. Real-time Data Processing
7.1. Using AI to analyze real-time data streams
Implement AI-powered data analysis on real-time data streams to extract valuable insights and trends.
python # Sample code for real-time data analysis using pandas and scikit-learn import pandas as pd from sklearn.cluster import KMeans def analyze_realtime_data(data_stream): df = pd.DataFrame(data_stream) # Perform clustering on the data kmeans = KMeans(n_clusters=3) df['cluster_label'] = kmeans.fit_predict(df) return df
7.2. Developing interactive and dynamic AI-infused dashboards
Create interactive dashboards that visualize AI-generated insights in real-time.
python # Sample code for interactive dashboards using Plotly import plotly.express as px def create_dashboard(data): fig = px.scatter(data, x='timestamp', y='value', color='cluster_label', title='Real-time Data Analysis', template='plotly_dark') return fig
Conclusion
Integrating AI into your Django web applications can elevate their capabilities to new heights. From sentiment analysis and personalized recommendations to NLP and computer vision, AI-powered features open up a world of possibilities for creating intelligent, user-centric web experiences. By following the steps outlined in this guide and experimenting with the provided code samples, you can embark on a journey to create innovative AI-infused web applications that cater to the evolving needs of your users. Happy coding!
Table of Contents
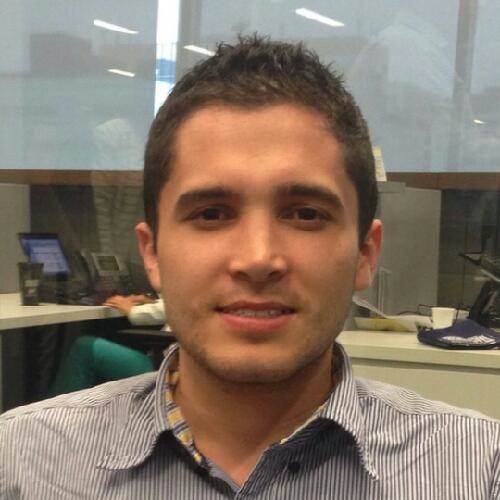
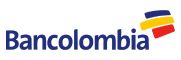