Android Testing: Strategies for Efficient and Reliable App Testing
In today’s fast-paced digital world, mobile applications have become an integral part of our lives. With the widespread adoption of Android devices, it is crucial for app developers to ensure that their Android applications are thoroughly tested to deliver a seamless user experience. This blog post will delve into the strategies for efficient and reliable Android app testing, exploring various testing techniques, frameworks, and tools that can aid developers in delivering high-quality apps.
1. The Importance of Android App Testing
App testing plays a vital role in ensuring the quality, performance, and reliability of Android applications. Testing helps identify and rectify bugs, compatibility issues, and usability problems before the app is released to the users. Comprehensive testing not only enhances the overall user experience but also helps in building trust and credibility among app users.
2. Types of Android App Testing
2.1 Unit Testing
Unit testing involves testing individual components or units of an application in isolation. By testing individual units, such as methods or classes, developers can identify and fix issues at an early stage. Robust unit testing helps in isolating bugs and ensures that each component of the app functions correctly.
java public class Calculator { public int add(int a, int b) { return a + b; } } java public class CalculatorTest { @Test public void testAdd() { Calculator calculator = new Calculator(); int result = calculator.add(2, 3); assertEquals(5, result); } }
2.2 Integration Testing
Integration testing verifies the interaction between different components of an Android app. It ensures that the integrated modules work seamlessly together and that data is transferred correctly between various components.
2.3 Functional Testing
Functional testing focuses on validating the functional aspects of an app. It ensures that the app’s features, navigation, and user interactions work as intended. Common functional testing techniques include input validation, boundary testing, and scenario-based testing.
2.4 Performance Testing
Performance testing evaluates an app’s responsiveness, stability, and resource consumption. It measures the app’s performance under different scenarios and identifies potential bottlenecks or areas for optimization. Techniques such as load testing and stress testing are commonly employed in performance testing.
2.5 User Interface Testing
User Interface (UI) testing involves testing the app’s user interface elements to ensure they are visually appealing, intuitive, and responsive. It verifies that buttons, forms, menus, and other UI components are functioning as expected.
3. Test Automation for Android Apps
3.1 Benefits of Test Automation
Test automation offers numerous advantages in the Android app testing process. It helps reduce the time and effort required for repetitive tests, enhances test coverage, improves accuracy, and facilitates faster release cycles. Automated tests can be executed on various devices and configurations, ensuring compatibility across different Android platforms.
3.2 Frameworks for Android Test Automation
There are several popular frameworks for automating Android app tests:
- Appium: An open-source framework that supports Android and iOS platforms, allowing testers to write tests using multiple programming languages.
- Espresso: A testing framework provided by Google for Android app UI testing. It offers a fluent and concise API for writing UI automation tests.
- UI Automator: Another framework provided by Google for testing Android apps. It enables cross-app and system-level UI testing.
- Robolectric: A framework that allows running unit tests on Android devices without the need for an emulator or a real device. It provides fast and reliable testing capabilities.
4. Emulators and Real Devices
To ensure comprehensive testing, developers can leverage a combination of emulators and real devices. Emulators provide a cost-effective way to test apps on various Android versions and configurations. However, real devices are crucial for testing performance, device-specific features, and user experience under real-world conditions. A combination of both emulators and real devices helps achieve a balanced and thorough testing approach.
5. Continuous Integration and Continuous Testing
5.1 CI/CD Pipelines
Implementing Continuous Integration (CI) and Continuous Delivery (CD) pipelines streamline the testing process by automating build, test, and deployment stages. CI/CD pipelines enable developers to validate code changes quickly and continuously, ensuring that any issues are caught early in the development cycle.
5.2 Testing in Parallel
Parallel testing involves running tests simultaneously across multiple devices or emulators, significantly reducing test execution time. By leveraging parallel testing, developers can achieve faster feedback on the app’s quality and improve overall testing efficiency.
6. Testing Tools for Android Apps
6.1 Android Testing Support Library
The Android Testing Support Library provides a collection of testing APIs and tools that simplify and accelerate the testing process. It includes features for unit testing, UI testing, and instrumented testing.
6.2 Espresso
Espresso is a widely used testing framework for Android app UI testing. It offers a rich set of APIs for interacting with UI elements and asserting their states, making it an efficient tool for UI automation.
java onView(withId(R.id.button)).perform(click()); onView(withId(R.id.textView)).check(matches(withText("Button Clicked")));
6.3 UI Automator
UI Automator is a framework provided by Google for testing the UI of Android apps. It allows cross-app and system-level UI testing, making it suitable for testing scenarios that span multiple apps.
java UiDevice device = UiDevice.getInstance(InstrumentationRegistry.getInstrumentation()); device.pressHome(); device.wait(Until.hasObject(By.text("App Name")), 5000); device.findObject(By.text("App Name")).click();
6.4 Robolectric
Robolectric is a framework that enables running unit tests on Android devices without the need for an emulator or a real device. It provides fast and reliable testing capabilities, allowing developers to test their code in isolation.
7. Best Practices for Android App Testing
7.1 Early Testing
Start testing as early as possible in the development process. Early testing helps catch issues sooner, reduces rework, and ensures a stable foundation for further development.
7.2 Test Coverage
Strive for comprehensive test coverage by targeting different types of testing (unit, integration, functional, performance) and ensuring that critical code paths are thoroughly tested.
7.3 Test Data Management
Effectively manage test data by creating reusable test cases and maintaining a test data repository. This approach simplifies test maintenance and enables easy replication of specific test scenarios.
7.4 Continuous Improvement
Continuously evaluate and refine your testing processes. Incorporate feedback from users and stakeholders to identify areas for improvement, enhance test coverage, and optimize testing efficiency.
Conclusion
Effective and reliable Android app testing is essential for delivering high-quality mobile applications. By adopting a combination of testing techniques, leveraging automation frameworks and tools, utilizing emulators and real devices, and implementing continuous testing practices, developers can ensure that their Android apps meet user expectations in terms of functionality, performance, and user experience. Embracing best practices and staying up-to-date with the latest testing advancements will contribute to the success of Android app development in today’s competitive market.
Table of Contents
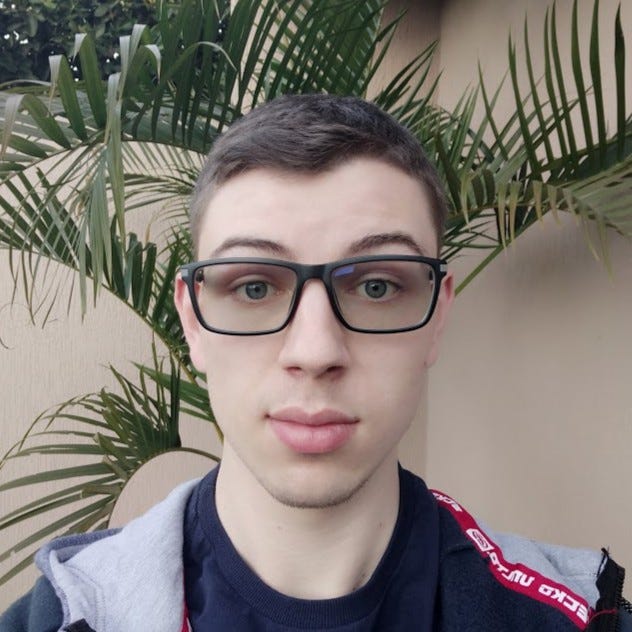
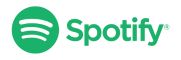