Java Essentials for Android Development: From Basic Concepts to Android-Specific Practices
In the realm of mobile application development, Android has emerged as one of the most popular platforms, and Java is widely used for its development. Companies looking to advance in this space often hire Android developers proficient in Java, despite the rise of modern programming languages like Kotlin. Java continues to play a crucial role in the Android development landscape.
This blog post aims to provide a comprehensive understanding of the key concepts of using Java in Android development, valuable to both aspiring Android developers and companies planning to hire Android developers. We will provide illustrative examples to ensure a thorough comprehension of these key concepts.
Java Basics for Android Development
Variables and Data Types
Variables in Java are essentially names given to memory locations that store data. Data types, on the other hand, define the type and size of values that can be stored in these variables. For example:
```java int myNumber = 10; String myString = "Hello, Android!";
In this code, `myNumber` and `myString` are variables, whereas `int` and `String` are data types.
Classes and Objects
Java is an Object-Oriented Programming (OOP) language, and classes and objects form the cornerstone of OOP in Java. A class is a blueprint for creating objects, and an object is an instance of a class. For example, consider this class:
```java public class Dog { String breed; int age; void bark() { System.out.println("Bark! Bark!"); } }
To create an object from this class, we can use the following code:
```java Dog myDog = new Dog(); myDog.breed = "Golden Retriever"; myDog.age = 5;
Functions (Methods)
Functions or methods are blocks of code designed to perform a specific task. In the previous `Dog` class, `bark()` is a method that makes the dog bark.
Java in Android Development
Now, let’s explore how these concepts translate into Android development with Java.
Activities
In Android, an activity represents a single screen with a user interface. It is implemented as a subclass of the `Activity` class. For instance, here’s a simple `MainActivity`:
```java public class MainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } }
The `onCreate()` method is a lifecycle callback, called when the activity is starting. `setContentView()` sets the layout for the activity to `activity_main.xml`.
XML Layouts
XML files define the layout for each activity. They specify the UI elements (like buttons and text views) and their arrangement on the screen. Here’s an example of an XML layout:
```xml <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello, Android!" /> </LinearLayout>
Intents
Intents in Android are messaging objects that you can use to request an action from another app component. There are two types of intents: explicit and implicit. An explicit intent is used to start a specific component, like starting an activity within your app:
```java Intent myIntent = new Intent(this, AnotherActivity.class); startActivity(myIntent);
An implicit intent, on the other hand, does not name a specific component. Instead, it declares a general action to perform, which allows a component from another app to handle it.
```java Intent sendIntent = new Intent(); sendIntent.setAction(Intent.ACTION_SEND ); sendIntent.putExtra(Intent.EXTRA_TEXT, "This is my text to send."); sendIntent.setType("text/plain"); startActivity(sendIntent);
AsyncTask
AsyncTask allows you to perform background tasks and publish results on the UI thread. It is commonly used for short operations (a few seconds at most). Here’s how you can use AsyncTask:
```java private class MyTask extends AsyncTask<Void, Void, String> { @Override protected String doInBackground(Void... params) { // Background work return "Done"; } @Override protected void onPostExecute(String result) { // Update UI textView.setText(result); } }
To execute this task, call `new MyTask().execute();`.
Key Libraries and APIs
Android development with Java also involves working with several important libraries and APIs. Let’s look at two of them.
Retrofit
Retrofit is a type-safe HTTP client for Android and Java. It’s used to retrieve and upload JSON (or other structured data) via a REST-based web service. Here’s a simple use of Retrofit:
```java Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://api.github.com/") .addConverterFactory(GsonConverterFactory.create()) .build(); GitHubService service = retrofit.create(GitHubService.class); Call<List<Repo>> repos = service.listRepos("octocat");
Picasso
Picasso is an image downloading and caching library for Android. It simplifies the process of displaying images from external locations. Here’s how you can load an image from a URL into an `ImageView`:
```java Picasso.get().load("http://example.com/image.jpg").into(imageView);
Conclusion
Understanding Java’s foundational concepts is crucial for Android development, whether you’re an individual learning to code or a company seeking to hire Android developers. Activities, XML Layouts, Intents, and AsyncTask are some of the Android-specific concepts that every developer should be familiar with. If you are looking to hire Android developers, these are key areas of expertise to consider. Furthermore, the ability to utilize libraries like Retrofit and Picasso can significantly streamline your development process and enhance your app’s capabilities. Having these skills makes one a more effective developer and a more attractive candidate for companies looking to hire Android developers. Happy coding!
Table of Contents
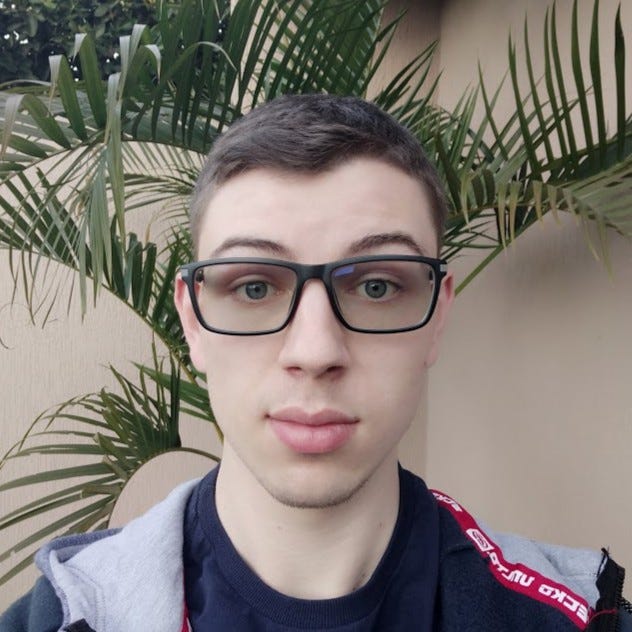
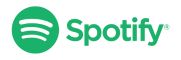