Android Development Essentials: A Comprehensive Guide for Beginners
Welcome to the world of Android programming! As the most widely used mobile operating system worldwide, Android offers tremendous opportunities for both new and experienced developers, as well as companies looking to hire Android developers. This guide is designed to take you through the essential concepts of Android programming, with practical examples for each concept. Whether you’re a beginner wanting to start your development journey, or an organization seeking to understand the competencies to look for when you hire Android developers, this guide is for you.
By the end of this post, you will be more familiar with the key aspects of Android development, ready to create fantastic apps or make informed hiring decisions. So, let’s get started!
What is Android Programming?
Android programming refers to the development of applications (apps) that run on devices using the Android operating system, such as smartphones and tablets. These apps can be developed using various programming languages such as Java and Kotlin, although Google currently recommends Kotlin as the language of choice for Android development.
Setting up the Environment
Before you dive into Android programming, or before you seek to hire Android developers for your project, you’ll need to understand the basics of the development environment. The primary tool for Android development is Android Studio, which is an Integrated Development Environment (IDE) specifically designed for Android. This platform offers a suite of powerful tools for coding, debugging, testing, and performance tuning, making it an essential knowledge area for any developer you plan to hire.
To install Android Studio, you can follow the instructions on the official Android Developer website. Once you have it installed, you are ready to either start creating your first Android application or to effectively gauge the technical competency of potential Android developers for hire. Understanding these tools will also help you communicate more effectively with your Android development team.
Building Your First Android App
Let’s dive into creating a simple app that greets the user by their name. This will introduce you to activities, layouts, and event handling – three fundamental components of Android development.
- Creating a New Project: Open Android Studio and select ‘New Project.’ Choose an ‘Empty Activity’ template. Name the application “MyFirstApp,” and select ‘Kotlin’ as the programming language, then click ‘Finish.’
- Understanding the Project Structure: The project will open with a structure comprising numerous directories and files. The most important are:
– `MainActivity.kt`: This is the main activity (or screen) of your app.
– `activity_main.xml`: This is the layout file that defines the user interface for your activity.
- Designing the User Interface: Open `activity_main.xml`. You’ll see a graphical interface at the top and an XML code editor at the bottom. Drag and drop a ‘Plain Text’ field and a ‘Button’ from the palette onto the phone screen in the graphical interface. The XML editor should reflect these changes.
```xml <EditText android:id="@+id/editTextTextPersonName" android:layout_width="wrap_content" android:layout_height="wrap_content" android:ems="10" android:hint="Enter your name" ... /> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Greet Me" ... />
4. Coding the Activity: Now, open `MainActivity.kt`. Here, you’ll code the behavior of your app. Declare references to your UI elements and implement an event listener for the button.
```kotlin class MainActivity : AppCompatActivity() { private lateinit var editText: EditText private lateinit var button: Button override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) editText = findViewById(R.id.editTextTextPersonName) button = findViewById(R.id.button) button.setOnClickListener { val name = editText.text.toString() Toast.makeText(this, "Hello, $name!", Toast.LENGTH_LONG).show() } } }
Run your app on an emulator or a real device. You should see a text field and a button. When you enter your name and press the button, you’ll be greeted with a “Hello, [YourName]!” message.
Understanding Android App Components
Android apps consist of several components that dictate the overall behavior of the application. Let’s take a closer look at these:
- Activities: An activity represents a single screen with a user interface. Our first app, for example, consisted of just one activity.
- Services: A service is a component that runs in the background to perform long-running operations or to perform work for remote processes.
- Content Providers: These manage a shared set of app data that you can store in the file system, a SQLite database, on the web, or on any other persistent storage location that your app can access.
- Broadcast Receivers: These respond to system-wide broadcast announcements.
These components are loosely bound using intents, a messaging object which facilitates communication between components.
Understanding the Activity Lifecycle
A crucial concept in Android programming is understanding the activity lifecycle, which defines a series of states an activity can be in, from creation to destruction. These states are tied to ‘lifecycle callback’ methods that you can override in your activity code:
- onCreate(): The system calls this when creating your activity. You should perform all static setup here.
- onStart(): This gets called when the activity becomes visible to the user.
- onResume(): This gets invoked when the user starts interacting with the application.
- onPause() and onStop(): These are called when the activity is no longer in the foreground.
- onDestroy(): The system invokes this method before the activity is destroyed.
Debugging and Testing
Debugging and testing are integral parts of software development. Android Studio offers numerous tools for these tasks, like Logcat for real-time monitoring of log messages, the Debugger for inspecting your code’s runtime behavior, and a robust testing framework that includes unit and UI testing.
Conclusion
Embarking on the journey to learn Android programming can be both exciting and rewarding. The ecosystem is vast, offering virtually endless possibilities not just for aspiring developers, but also for businesses looking to hire Android developers. In this blog post, we’ve only scratched the surface of Android development, but hopefully, you now have a solid foundation and understanding of what it takes to create an Android app from scratch. This knowledge is not only invaluable to budding programmers, but also to those who want to hire Android developers, as it provides insight into the skills and knowledge that an expert Android developer should possess.
Remember, the journey of a thousand miles begins with a single step. So, whether you’re an individual ready to dive into coding, or an organization preparing to hire Android developers, keep exploring, keep experimenting, and have fun while you’re at it. Your journey into the world of Android programming has just begun!
Table of Contents
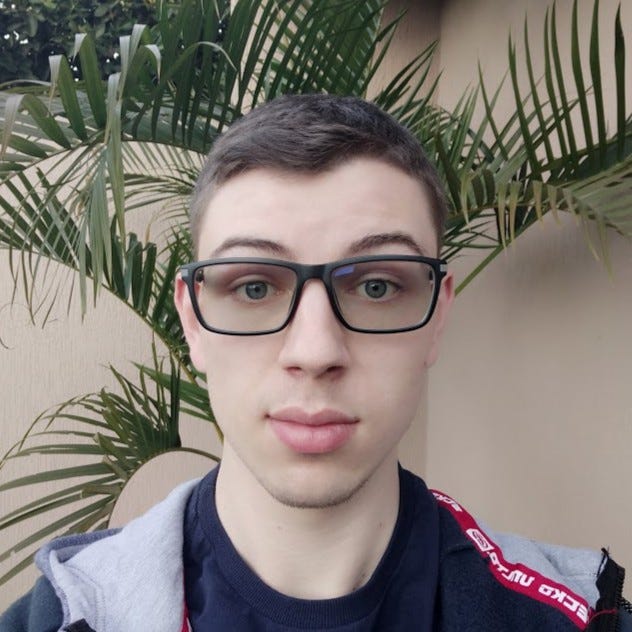
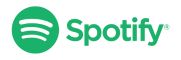