Working with Permissions in Android: Requesting and Handling Access
As an Android developer, working with permissions is an essential part of building secure and user-friendly applications. Permissions grant or deny access to various system resources and user data, ensuring that apps function within the defined security boundaries. In this comprehensive guide, we will explore how to effectively request and handle permissions in Android, along with best practices to create a seamless user experience.
1. Understanding Android Permissions
Before diving into the process of requesting and handling permissions, it’s important to understand the different types and levels of permissions in Android.
1.2 Permission Types
Android permissions are categorized into two types: normal and dangerous permissions.
- Normal Permissions: These permissions do not directly impact user privacy or sensitive data. Android automatically grants normal permissions at the time of installation, without user interaction.
- Dangerous Permissions: These permissions are considered sensitive as they provide access to user data or device features that can affect privacy or functionality. Examples include accessing the camera, location, or reading contacts. Dangerous permissions require explicit user approval before granting access.
1.3 Permission Levels
Android permissions are further classified into permission levels based on the scope of access they provide:
- Normal Permissions: These permissions have a protection level of “normal.” They pose minimal risk to user privacy and are automatically granted at installation.
- Dangerous Permissions: These permissions have a protection level of “dangerous.” They require explicit user approval and are requested at runtime. Failure to obtain dangerous permissions can result in the app’s restricted functionality.
2. Requesting Permissions
Now that we have a clear understanding of permissions, let’s delve into the process of requesting them in Android.
2.1 Checking Permission Status
Before requesting a dangerous permission, it’s essential to check whether the permission has already been granted by the user. This step helps avoid unnecessary permission requests and provides a better user experience.
To check the permission status, we can use the checkSelfPermission() method provided by the ContextCompat class. Here’s an example of checking the camera permission:
java if (ContextCompat.checkSelfPermission(context, Manifest.permission.CAMERA) == PackageManager.PERMISSION_GRANTED) { // Permission has already been granted // Proceed with accessing the camera } else { // Permission has not been granted // Request the permission }
2.2 Requesting Permissions at Runtime
To request a dangerous permission at runtime, we use the requestPermissions() method, which presents a system dialog to the user, asking for permission approval. This dialog contains the permission rationale and options for granting or denying access.
Here’s an example of requesting the camera permission:
java ActivityCompat.requestPermissions(activity, new String[]{Manifest.permission.CAMERA}, REQUEST_CAMERA_PERMISSION);
After requesting the permission, Android invokes the onRequestPermissionsResult() callback in the activity or fragment. We need to override this method to handle the permission result.
3. Handling Permission Results
Handling permission results is crucial to ensure a smooth user experience and maintain app functionality. Let’s explore the different scenarios that can occur after requesting permissions.
3.1 Handling Permission Granted
When the user grants the requested permission, the onRequestPermissionsResult() callback is triggered with the granted permission result. We can perform the desired action, such as accessing the requested resource. Here’s an example:
java @Override public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) { if (requestCode == REQUEST_CAMERA_PERMISSION && grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) { // Camera permission has been granted // Proceed with accessing the camera } else { // Permission denied // Handle accordingly } }
3.2 Handling Permission Denied
If the user denies the requested permission, the onRequestPermissionsResult() callback is invoked with the denied permission result. In this case, we can display a message or prompt the user to grant the permission again. It’s important to handle the denied permission scenario gracefully to maintain a positive user experience.
3.3 Handling Permission Rationale
Android provides an option to present a permission rationale dialog before requesting a permission. This dialog should explain why the permission is needed and reassure the user about the app’s intentions. By providing clear and concise explanations, we can increase the chances of obtaining permission approval.
To show the permission rationale, we can utilize the shouldShowRequestPermissionRationale() method provided by the ActivityCompat class. Here’s an example:
java if (ActivityCompat.shouldShowRequestPermissionRationale(activity, Manifest.permission.CAMERA)) { // Show permission rationale dialog // Explain why the camera permission is needed } else { // Request the permission directly ActivityCompat.requestPermissions(activity, new String[]{Manifest.permission.CAMERA}, REQUEST_CAMERA_PERMISSION); }
4. Best Practices for Permission Management
To create a seamless and secure user experience, it’s important to follow these best practices for permission management in your Android applications.
4.1 Only Request Necessary Permissions
Request only the permissions that are essential for your app’s functionality. Unnecessary permission requests can create suspicion and lead to a negative user experience. Carefully consider the features your app requires and request permissions accordingly.
4.2 Handle Permission Changes Gracefully
Permissions can change dynamically on Android, especially on devices running Android 6.0 (Marshmallow) or higher. Users can revoke or grant permissions from the system settings at any time. It’s crucial to handle these changes gracefully and ensure your app can adapt to different permission scenarios.
4.3 Provide Clear Explanations and Rationales
When requesting dangerous permissions, provide clear explanations and rationales to the user. This helps build trust and increases the likelihood of permission approval. Avoid generic or vague messages and provide context-specific explanations tailored to your app’s features.
4.4 Test on Different Permission Scenarios
During development, thoroughly test your app on different permission scenarios. Test cases should include granting and denying permissions, as well as revoking permissions after they have been granted. This ensures that your app behaves correctly in various permission scenarios and provides a smooth user experience.
Conclusion
In this comprehensive guide, we explored the process of requesting and handling permissions in Android. We discussed the different types and levels of permissions, along with the best practices for permission management. By following these guidelines, you can create secure and user-friendly applications that respect user privacy and provide a seamless experience. Effective permission management is essential for building trust and maintaining a positive relationship with your users.
Table of Contents
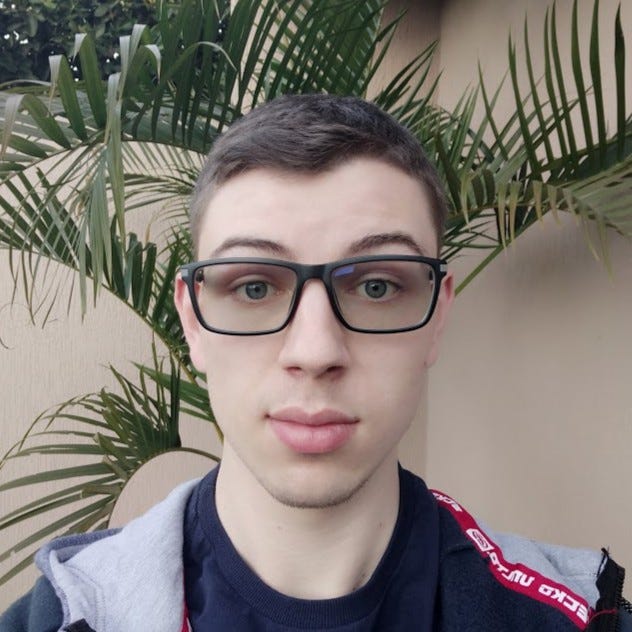
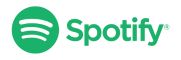