The Ultimate Guide to Android Intent Filters: System and App Events Uncovered
In the vast ecosystem of Android development, intent filters play a pivotal role by enabling apps to respond to both system and other app-initiated events. If Android development was a symphony, intent filters would be its conductors. They guide the flow of activities, help decide which component should be awakened in response to a broadcast, or assist in choosing which app should open a particular file type.
Table of Contents
In this blog post, we’ll delve into the realm of intent filters, examine their functionalities, and explore examples that demonstrate their application in the real-world scenarios.
1. What are Intent Filters?
In Android, an Intent represents an operation to be performed. This could be as straightforward as launching an activity or as specialized as dialing a phone number. Intent filters, as the name suggests, filter these intents. They allow apps to declare the types of intents they can respond to.
They’re typically declared in the `AndroidManifest.xml` of the app and are associated with either activities, services, or broadcast receivers.
Example 1: Responding to a URL
Let’s assume you’ve developed a unique e-book reader. To ensure that any e-book URL is opened with your app, you need to use an intent filter.
```xml <activity android:name=".MyEbookReaderActivity"> <intent-filter> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <data android:scheme="http" android:host="www.ebookwebsite.com" android:pathPrefix="/ebooks" /> </intent-filter> </activity> ```
Here:
– The `action` tag specifies that this filter responds to view actions.
– The `category` tags make sure that this activity is a default handler and can be invoked by a web browser.
– The `data` tag defines the specific kind of URL that this activity responds to.
With this setup, anytime a user clicks on a link starting with `http://www.ebookwebsite.com/ebooks`, your e-book reader app would be suggested to handle that link.
Example 2: Responding to System Boot
Let’s say you want your app to perform a certain action or notification every time the device completes its booting process. You’d use a Broadcast Receiver with an intent filter:
```xml <receiver android:name=".BootCompletedReceiver"> <intent-filter> <action android:name="android.intent.action.BOOT_COMPLETED"/> </intent-filter> </receiver> ```
Ensure that you also request the `RECEIVE_BOOT_COMPLETED` permission in your manifest.
Example 3: Responding to Shared Images
Imagine you’ve made an image-editing app and want it to be an option whenever someone tries to share an image from their gallery or any other app. Here’s how:
```xml <activity android:name=".ImageEditActivity" > <intent-filter> <action android:name="android.intent.action.SEND" /> <category android:name="android.intent.category.DEFAULT" /> <data android:mimeType="image/*" /> </intent-filter> </activity> ```
The `SEND` action indicates that the app can accept shared items, and the `mimeType` restricts the sharing to image types.
Example 4: Handling Outgoing Calls
Let’s assume you’re developing a custom dialer or a call-log tracker. You’d want your app to spring into action when an outgoing call is made:
```xml <receiver android:name=".OutgoingCallReceiver"> <intent-filter> <action android:name="android.intent.action.NEW_OUTGOING_CALL"/> </intent-filter> </receiver> ```
For this, you’d also need the `PROCESS_OUTGOING_CALLS` permission.
2. Best Practices with Intent Filters
- Specificity Matters: Be as specific as possible with your filters. Overly broad intent filters can make your app appear in unrelated contexts, which can frustrate users.
- Test Thoroughly: Given the multitude of Android devices and variations in custom Android versions by manufacturers, always test on multiple devices and Android versions.
- Security Concerns: Beware of implicitly broadcasting sensitive data. Always use explicit intents for sensitive operations.
- Avoid Unnecessary Duplication: If multiple components can handle the same intent, it can lead to a confusing UX. Keep user convenience in mind.
Conclusion
Android intent filters are powerful tools in the hands of developers. They help ensure that the right action happens at the right time, enhancing user experience and allowing apps to integrate seamlessly into the broader Android ecosystem. With the right intent filter setups, your app can interact harmoniously with system and other app events, making the Android world a little more interconnected.
Remember, with great power comes great responsibility. While intent filters can elevate the functionality of your app, they must be used judiciously to keep the user experience streamlined and intuitive. Happy coding!
Table of Contents
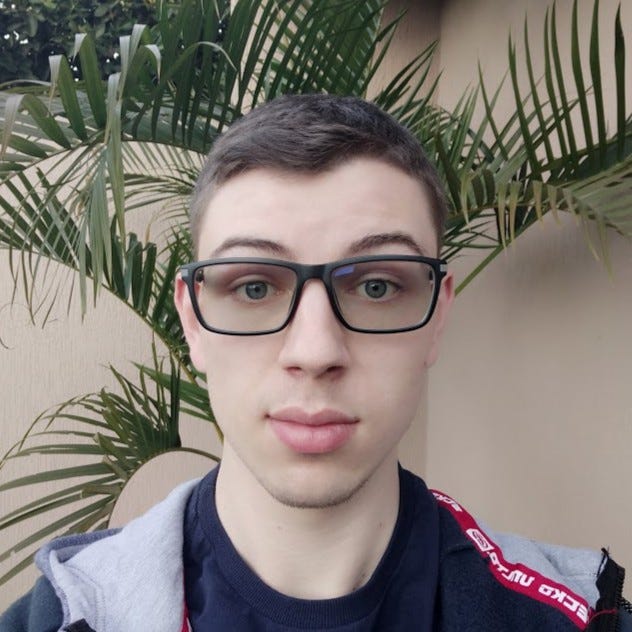
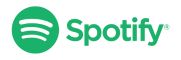