Interview Guide: Hire Android Developers
Android is one of the most widely used mobile operating systems, and hiring skilled Android developers is essential for building successful mobile applications.
Table of Contents
This comprehensive interview guide will help you identify top Android talent and build a proficient development team.
1. Key Skills of Android Developers to Look Out For:
Before diving into the interview process, take note of the crucial skills to look for in Android developers:
- Java/Kotlin Proficiency: Strong knowledge of Java or Kotlin programming languages, with a focus on Android development.
- Android SDK & APIs: Experience with Android SDK and various APIs to build feature-rich and user-friendly apps.
- UI/UX Design: Familiarity with Android UI design principles and the ability to create visually appealing and responsive user interfaces.
- Data Storage & Databases: Knowledge of data storage options in Android, such as SharedPreferences, SQLite, and Room Persistence Library.
- Networking & RESTful APIs: Experience in handling network requests and integrating data from RESTful APIs.
- Memory Management & Performance Optimization: Understanding of memory management and performance optimization techniques for Android apps.
2. An Overview of the Android Developer Hiring Process:
- Defining Job Requirements & Skills: Clearly outline the technical skills and experience you are seeking in an Android developer, including Java/Kotlin proficiency, Android SDK knowledge, and UI/UX design expertise.
- Creating an Effective Job Description: Craft a comprehensive job description with a clear title, specific requirements, and details about the projects the developer will work on.
- Preparing Interview Questions: Develop a list of technical questions to assess candidates’ Android knowledge, problem-solving abilities, and experience in building mobile applications.
- Evaluating Candidates: Use a combination of technical assessments, coding challenges, and face-to-face interviews to evaluate potential candidates and determine the best fit for your organization.
3. Technical Interview Questions and Sample Answers:
Q1. Explain the activity lifecycle in Android. How do you handle configuration changes during an activity’s lifecycle?
Sample Answer:
The activity lifecycle in Android consists of several stages, including onCreate, onStart, onResume, onPause, onStop, and onDestroy. These methods are called as an activity transitions between different states. To handle configuration changes, such as screen rotation, we can override the onConfigurationChanged method in the activity and manage the necessary UI updates or data retention to ensure a smooth user experience.
Q2. What is the ViewHolder pattern, and why is it important in Android development?
Sample Answer:
The ViewHolder pattern is used in Android’s RecyclerView to improve the performance of list-based views. It involves creating a ViewHolder object to hold references to the views within each item of the RecyclerView. By using the ViewHolder pattern, we can avoid repetitive findViewById calls, which significantly improves scrolling performance and reduces the load on the UI thread.
Q3. How do you manage background tasks in Android to avoid blocking the main UI thread? Provide an example of using AsyncTask.
Sample Answer:
To avoid blocking the main UI thread and keep the app responsive, we use background tasks in Android. AsyncTask is one of the ways to achieve this. For example:
private class MyTask extends AsyncTask<Void, Void, String> { @Override protected String doInBackground(Void... params) { // Perform background computation here return "Result"; } @Override protected void onPostExecute(String result) { // Update UI with the result after background task is completed } }
Q4. Explain the differences between a Fragment and an Activity in Android. When would you use each?
Sample Answer:
In Android, an Activity represents a single screen with a user interface, while a Fragment is a portion of an activity that can be combined with other fragments to create a multi-pane user interface. We use Activities to represent complete screens and handle user interactions. Fragments, on the other hand, are used for UI modularity and flexibility, especially in tablet layouts or complex UI designs.
Q5. How do you work with data storage options in Android, and what are the differences between SharedPreferences and SQLite?
Sample Answer:
In Android, we have multiple data storage options, including SharedPreferences and SQLite. SharedPreferences are used for storing simple key-value pairs, such as user preferences. On the other hand, SQLite is a relational database that offers a more robust and structured way to store data. We use SharedPreferences for lightweight data storage and quick retrieval, while SQLite is suitable for complex data structures and efficient querying.
Q6. Explain the concept of Content Providers in Android and how they facilitate data sharing between apps.
Sample Answer:
Content Providers in Android enable data sharing between different apps in a secure and controlled manner. They allow one app to access and modify data from another app’s database or files. Content Providers are particularly useful for sharing data with other apps while maintaining data security and integrity. For example, the Contacts app can provide a Content Provider that allows other apps to access contact information without exposing the underlying data structure.
Q7. How do you handle network requests and API integration in Android? Provide an example of using Retrofit for RESTful API calls.
Sample Answer:
In Android, we use libraries like Retrofit to handle network requests and API integration. Retrofit simplifies the process of making RESTful API calls. For example:
public interface ApiService { @GET("users/{userId}") Call<User> getUser(@Path("userId") int userId); } Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://api.example.com/") .addConverterFactory(GsonConverterFactory.create()) .build(); ApiService apiService = retrofit.create(ApiService.class); Call<User> call = apiService.getUser(1); call.enqueue(new Callback<User>() { @Override public void onResponse(Call<User> call, Response<User> response) { if (response.isSuccessful()) { User user = response.body(); // Handle the user data } } @Override public void onFailure(Call<User> call, Throwable t) { // Handle network errors } });
Q8. Explain the concept of Parcelable in Android and why it is used for inter-process communication.
Sample Answer:
Parcelable is an Android-specific interface used for inter-process communication (IPC). It allows us to serialize and deserialize custom objects to pass data between different Android components, such as Activities and Services. Parcelable is preferred over Serializable in Android because it offers better performance, especially when transferring large data objects between processes.
Q9. How do you handle user input validation in Android to ensure data integrity and a smooth user experience?
Sample Answer:
User input validation is crucial to ensure data integrity and provide a smooth user experience. In Android, we can use various validation techniques, such as InputFilter, regular expressions, or TextWatcher. For example, using a TextWatcher, we can monitor text changes in an EditText and validate the input in real-time, providing feedback to the user about the validity of their input.
Q10. Explain the principles of Material Design in Android and how you implement them in your apps.
Sample Answer:
Material Design is a design language developed by Google, providing guidelines for creating visually appealing and intuitive user interfaces in Android apps. It emphasizes simplicity, consistency, and meaningful motion. To implement Material Design, we use components like MaterialButton, MaterialCardView, and BottomNavigationView. We also follow the principles of elevation, color theming, and appropriate typography to ensure a cohesive and user-friendly app design.
4. The Importance of the Interview Process to Find the Right Android Talent:
The interview process is a critical step in identifying the right Android developers for your mobile app projects. Android development requires a diverse set of skills, including programming proficiency, UI/UX design, and knowledge of Android SDK and APIs. Thorough technical interviews help evaluate candidates’ problem-solving abilities and their ability to build scalable and efficient Android applications.
5. How CloudDevs Can Help You Hire Android Developers:
Hiring top Android developers can be a challenging task, especially when you need to find developers with specific expertise. CloudDevs offers a reliable platform to simplify your hiring process and connect you with pre-screened senior Android developers.
Here’s how CloudDevs can assist you:
- Discuss your project requirements and desired skill sets with a dedicated consultant.
- Receive a curated shortlist of top Android developers within a short period.
- Conduct technical interviews and coding assessments to evaluate candidates’ proficiency.
- Start a risk-free trial with your selected Android developer to ensure a perfect fit for your projects.
With CloudDevs, you can build a highly capable Android development team and focus on creating innovative and user-friendly mobile applications. Leave the hiring process to the experts at CloudDevs and embark on your Android app development journey with confidence.
Table of Contents
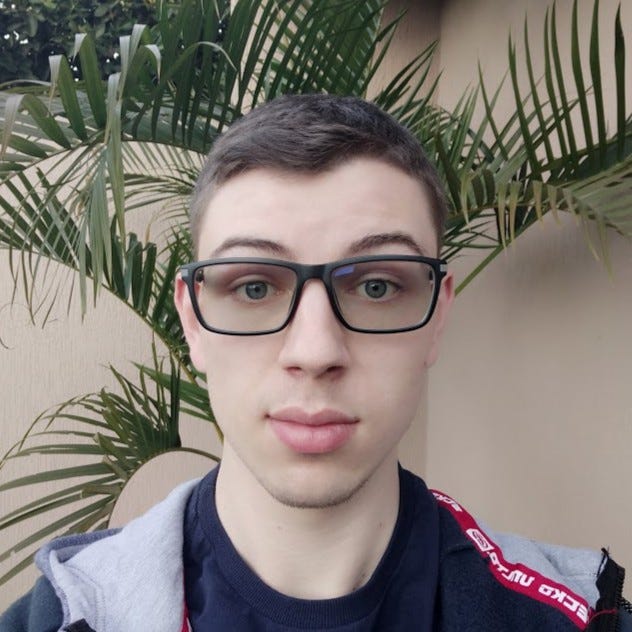
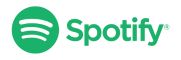