Android Multithreading: Concurrent Programming Techniques
In the fast-paced world of mobile app development, responsiveness and performance are key factors that determine the success of an Android application. As smartphones and tablets continue to advance in processing power, developers must leverage the full potential of multi-core processors to ensure their apps run smoothly and efficiently. This is where multithreading and concurrent programming techniques come into play.
1. What is Multithreading?
Multithreading is the ability of a program to execute multiple threads simultaneously, allowing it to perform multiple tasks concurrently. In the context of Android, this means that your application can perform different operations at the same time, resulting in better overall performance and user experience.
2. The Importance of Multithreading in Android:
Android devices are equipped with multi-core processors that can handle multiple threads efficiently. However, many Android applications still run on a single thread, which can lead to performance bottlenecks and unresponsive user interfaces. By implementing multithreading, you can take full advantage of the available hardware resources and distribute the workload across multiple threads, making your app more responsive and efficient.
3. Concurrency in Android:
Concurrency is a broader concept that encompasses multithreading. It involves designing your application to handle multiple tasks and processes simultaneously, regardless of whether they are executed on a single core or multiple cores. Android provides various tools and APIs to achieve concurrency and parallelism effectively.
4. Common Multithreading Challenges:
Before diving into concurrent programming techniques in Android, let’s explore some common challenges developers face when dealing with multithreading:
- Race Conditions: These occur when multiple threads access and modify shared data concurrently, leading to unexpected and erroneous behavior.
- Deadlocks: Deadlocks happen when two or more threads are blocked forever, waiting for each other to release resources.
- Thread Synchronization: Ensuring proper synchronization between threads is crucial to avoid data corruption and maintain consistency.
- Thread Coordination: Coordinating the execution of multiple threads can be complex, especially when one thread depends on the result of another.
- Performance Overhead: Creating and managing threads can introduce overhead, affecting overall performance if not handled efficiently.
Now that we understand the challenges, let’s explore some popular concurrent programming techniques in Android.
4.1. AsyncTask:
AsyncTask is a simple and widely used class in Android for performing background operations on a separate thread. It provides an easy way to perform tasks in the background and update the UI thread with the results.
java public class MyAsyncTask extends AsyncTask<Void, Integer, String> { @Override protected void onPreExecute() { super.onPreExecute(); // Perform setup tasks before executing background operation (UI thread) } @Override protected String doInBackground(Void... voids) { // Perform background computation here (background thread) return "Task completed successfully!"; } @Override protected void onProgressUpdate(Integer... progress) { super.onProgressUpdate(progress); // Update UI with progress information (UI thread) } @Override protected void onPostExecute(String result) { super.onPostExecute(result); // Update UI with the result of background operation (UI thread) } }
Using AsyncTask, you can easily execute background tasks and update the UI thread without managing threads explicitly.
4.2. Thread and Runnable:
Java’s native Thread class and the Runnable interface are the building blocks of multithreading. You can create and start a thread by extending the Thread class or implementing the Runnable interface.
java public class MyRunnable implements Runnable { @Override public void run() { // Perform background computation here } } // Creating and starting a new thread using Runnable Thread myThread = new Thread(new MyRunnable()); myThread.start();
While using Thread and Runnable gives you more control over threads, you need to handle synchronization and communication between threads manually.
4.3. Handler and Looper:
Android’s Handler and Looper classes provide a way to communicate between the UI thread and background threads. They allow you to schedule tasks to be executed on the UI thread from a background thread.
java public class MyThread extends Thread { private Handler handler; @Override public void run() { // Perform background computation here // Use the handler to communicate with the UI thread handler.post(new Runnable() { @Override public void run() { // Update UI with the result of background operation } }); } } // In the UI thread, create a Handler attached to the UI thread's Looper Handler handler = new Handler(Looper.getMainLooper());
Using Handler and Looper, you can ensure that UI updates happen on the UI thread, avoiding potential conflicts with background threads.
4.4. ThreadPoolExecutor:
ThreadPoolExecutor is a more advanced way to manage a pool of threads efficiently. It allows you to specify the core pool size, maximum pool size, and keep-alive time for threads, providing better control over resource utilization.
java // Creating a ThreadPoolExecutor with four threads Executor executor = new ThreadPoolExecutor(4, 4, 60, TimeUnit.SECONDS, new LinkedBlockingQueue<>()); // Submit tasks to the executor executor.execute(new Runnable() { @Override public void run() { // Perform background computation here } });
Using a ThreadPoolExecutor, you can optimize the use of threads and execute multiple tasks concurrently without creating and destroying threads repeatedly.
4.5. Kotlin Coroutines:
Kotlin Coroutines provide a more intuitive and concise way to handle multithreading and concurrency in Android. Coroutines allow you to write asynchronous code in a sequential manner, simplifying complex tasks.
kotlin // Define a coroutine using the 'suspend' keyword suspend fun doBackgroundTask(): String { // Perform background computation here return "Task completed successfully!" } // Launch the coroutine from a coroutine scope (e.g., ViewModelScope or GlobalScope) viewModelScope.launch { val result = doBackgroundTask() // Update UI with the result }
Kotlin Coroutines handle thread management automatically and can switch between threads seamlessly, making code cleaner and more maintainable.
Conclusion
Android multithreading and concurrent programming techniques are essential for developing high-performance and responsive applications. By understanding the challenges and leveraging tools like AsyncTask, Thread, Handler, ThreadPoolExecutor, and Kotlin Coroutines, developers can optimize their apps for multi-core processors and enhance the user experience. So, start exploring these techniques in your Android projects and witness the benefits of concurrent programming firsthand. Happy coding!
Table of Contents
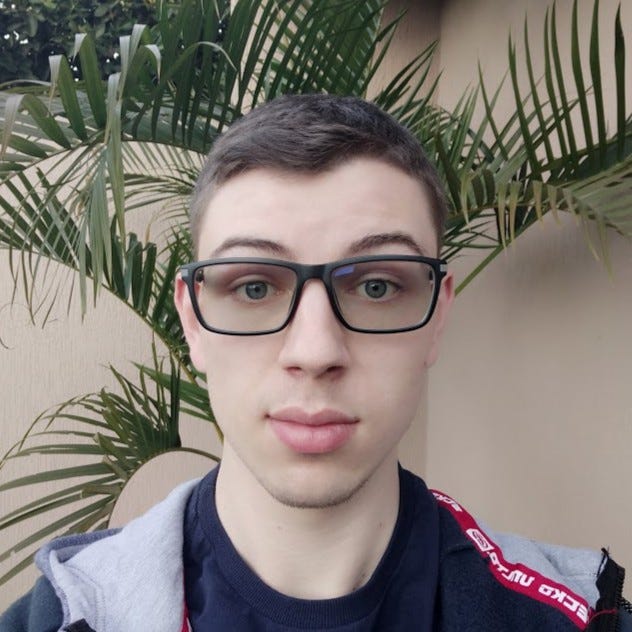
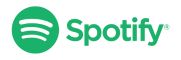